
Modbus Registers
Functions | |
eMBErrorCode | eMBRegInputCB (UCHAR *pucRegBuffer, USHORT usAddress, USHORT usNRegs) |
Callback function used if the value of a Input Register is required by the protocol stack. The starting register address is given by usAddress and the last register is given by usAddress + usNRegs - 1 . | |
eMBErrorCode | eMBRegHoldingCB (UCHAR *pucRegBuffer, USHORT usAddress, USHORT usNRegs, eMBRegisterMode eMode) |
Callback function used if a Holding Register value is read or written by the protocol stack. The starting register address is given by usAddress and the last register is given by usAddress + usNRegs - 1 . | |
eMBErrorCode | eMBRegCoilsCB (UCHAR *pucRegBuffer, USHORT usAddress, USHORT usNCoils, eMBRegisterMode eMode) |
Callback function used if a Coil Register value is read or written by the protocol stack. If you are going to use this function you might use the functions xMBUtilSetBits( ) and xMBUtilGetBits( ) for working with bitfields. | |
eMBErrorCode | eMBRegDiscreteCB (UCHAR *pucRegBuffer, USHORT usAddress, USHORT usNDiscrete) |
Callback function used if a Input Discrete Register value is read by the protocol stack. |
Detailed Description
#include "mb.h"
The protocol stack does not internally allocate any memory for the registers. This makes the protocol stack very small and also usable on low end targets. In addition the values don't have to be in the memory and could for example be stored in a flash.
Whenever the protocol stack requires a value it calls one of the callback function with the register address and the number of registers to read as an argument. The application should then read the actual register values (for example the ADC voltage) and should store the result in the supplied buffer.
If the protocol stack wants to update a register value because a write register function was received a buffer with the new register values is passed to the callback function. The function should then use these values to update the application register values.
Function Documentation
eMBErrorCode eMBRegCoilsCB | ( | UCHAR * | pucRegBuffer, |
USHORT | usAddress, | ||
USHORT | usNCoils, | ||
eMBRegisterMode | eMode | ||
) |
Callback function used if a Coil Register value is read or written by the protocol stack. If you are going to use this function you might use the functions xMBUtilSetBits( ) and xMBUtilGetBits( ) for working with bitfields.
- Parameters:
-
pucRegBuffer The bits are packed in bytes where the first coil starting at address usAddress
is stored in the LSB of the first byte in the bufferpucRegBuffer
. If the buffer should be written by the callback function unused coil values (I.e. if not a multiple of eight coils is used) should be set to zero.usAddress The first coil number. usNCoils Number of coil values requested. eMode If eMBRegisterMode::MB_REG_WRITE the application values should be updated from the values supplied in the buffer pucRegBuffer
. If eMBRegisterMode::MB_REG_READ the application should store the current values in the bufferpucRegBuffer
.
- Returns:
- The function must return one of the following error codes:
- eMBErrorCode::MB_ENOERR If no error occurred. In this case a normal Modbus response is sent.
- eMBErrorCode::MB_ENOREG If the application does not map an coils within the requested address range. In this case a ILLEGAL DATA ADDRESS is sent as a response.
- eMBErrorCode::MB_ETIMEDOUT If the requested register block is currently not available and the application dependent response timeout would be violated. In this case a SLAVE DEVICE BUSY exception is sent as a response.
- eMBErrorCode::MB_EIO If an unrecoverable error occurred. In this case a SLAVE DEVICE FAILURE exception is sent as a response.
eMBErrorCode eMBRegDiscreteCB | ( | UCHAR * | pucRegBuffer, |
USHORT | usAddress, | ||
USHORT | usNDiscrete | ||
) |
Callback function used if a Input Discrete Register value is read by the protocol stack.
If you are going to use his function you might use the functions xMBUtilSetBits( ) and xMBUtilGetBits( ) for working with bitfields.
- Parameters:
-
pucRegBuffer The buffer should be updated with the current coil values. The first discrete input starting at usAddress
must be stored at the LSB of the first byte in the buffer. If the requested number is not a multiple of eight the remaining bits should be set to zero.usAddress The starting address of the first discrete input. usNDiscrete Number of discrete input values.
- Returns:
- The function must return one of the following error codes:
- eMBErrorCode::MB_ENOERR If no error occurred. In this case a normal Modbus response is sent.
- eMBErrorCode::MB_ENOREG If no such discrete inputs exists. In this case a ILLEGAL DATA ADDRESS exception frame is sent as a response.
- eMBErrorCode::MB_ETIMEDOUT If the requested register block is currently not available and the application dependent response timeout would be violated. In this case a SLAVE DEVICE BUSY exception is sent as a response.
- eMBErrorCode::MB_EIO If an unrecoverable error occurred. In this case a SLAVE DEVICE FAILURE exception is sent as a response.
eMBErrorCode eMBRegHoldingCB | ( | UCHAR * | pucRegBuffer, |
USHORT | usAddress, | ||
USHORT | usNRegs, | ||
eMBRegisterMode | eMode | ||
) |
Callback function used if a Holding Register value is read or written by the protocol stack. The starting register address is given by usAddress
and the last register is given by usAddress + usNRegs - 1
.
- Parameters:
-
pucRegBuffer If the application registers values should be updated the buffer points to the new registers values. If the protocol stack needs to now the current values the callback function should write them into this buffer. usAddress The starting address of the register. usNRegs Number of registers to read or write. eMode If eMBRegisterMode::MB_REG_WRITE the application register values should be updated from the values in the buffer. For example this would be the case when the Modbus master has issued an WRITE SINGLE REGISTER command. If the value eMBRegisterMode::MB_REG_READ the application should copy the current values into the buffer pucRegBuffer
.
- Returns:
- The function must return one of the following error codes:
- eMBErrorCode::MB_ENOERR If no error occurred. In this case a normal Modbus response is sent.
- eMBErrorCode::MB_ENOREG If the application can not supply values for registers within this range. In this case a ILLEGAL DATA ADDRESS exception frame is sent as a response.
- eMBErrorCode::MB_ETIMEDOUT If the requested register block is currently not available and the application dependent response timeout would be violated. In this case a SLAVE DEVICE BUSY exception is sent as a response.
- eMBErrorCode::MB_EIO If an unrecoverable error occurred. In this case a SLAVE DEVICE FAILURE exception is sent as a response.
eMBErrorCode eMBRegInputCB | ( | UCHAR * | pucRegBuffer, |
USHORT | usAddress, | ||
USHORT | usNRegs | ||
) |
Callback function used if the value of a Input Register is required by the protocol stack. The starting register address is given by usAddress
and the last register is given by usAddress + usNRegs - 1
.
- Parameters:
-
pucRegBuffer A buffer where the callback function should write the current value of the modbus registers to. usAddress The starting address of the register. Input registers are in the range 1 - 65535. usNRegs Number of registers the callback function must supply.
- Returns:
- The function must return one of the following error codes:
- eMBErrorCode::MB_ENOERR If no error occurred. In this case a normal Modbus response is sent.
- eMBErrorCode::MB_ENOREG If the application can not supply values for registers within this range. In this case a ILLEGAL DATA ADDRESS exception frame is sent as a response.
- eMBErrorCode::MB_ETIMEDOUT If the requested register block is currently not available and the application dependent response timeout would be violated. In this case a SLAVE DEVICE BUSY exception is sent as a response.
- eMBErrorCode::MB_EIO If an unrecoverable error occurred. In this case a SLAVE DEVICE FAILURE exception is sent as a response.
Generated on Sun Jul 17 2022 02:04:12 by
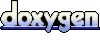