
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * FreeModbus Libary: BARE Demo Application 00003 * Copyright (C) 2006 Christian Walter <wolti@sil.at> 00004 * 00005 * This program is free software; you can redistribute it and/or modify 00006 * it under the terms of the GNU General Public License as published by 00007 * the Free Software Foundation; either version 2 of the License, or 00008 * (at your option) any later version. 00009 * 00010 * This program is distributed in the hope that it will be useful, 00011 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00012 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00013 * GNU General Public License for more details. 00014 * 00015 * You should have received a copy of the GNU General Public License 00016 * along with this program; if not, write to the Free Software 00017 * Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00018 * 00019 * File: $Id: demo.c,v 1.1 2006/08/22 21:35:13 wolti Exp $ 00020 */ 00021 00022 /* ----------------------- System includes --------------------------------*/ 00023 00024 /* ----------------------- Modbus includes ----------------------------------*/ 00025 #include "mb.h" 00026 #include "mbport.h" 00027 00028 /* ----------------------- Defines ------------------------------------------*/ 00029 #define REG_INPUT_START 1000 00030 #define REG_INPUT_NREGS 4 00031 #define SLAVE_ID 0x0A 00032 00033 /* ----------------------- Static variables ---------------------------------*/ 00034 static USHORT usRegInputStart = REG_INPUT_START; 00035 static USHORT usRegInputBuf[REG_INPUT_NREGS]; 00036 00037 /* ----------------------- Start implementation -----------------------------*/ 00038 int 00039 main( void ) 00040 { 00041 eMBErrorCode eStatus; 00042 00043 eStatus = eMBInit( MB_RTU , SLAVE_ID, 0, 9600, MB_PAR_NONE ); 00044 00045 /* Enable the Modbus Protocol Stack. */ 00046 eStatus = eMBEnable( ); 00047 00048 // Initialise some registers 00049 usRegInputBuf[1] = 0x1234; 00050 usRegInputBuf[2] = 0x5678; 00051 usRegInputBuf[3] = 0x9abc; 00052 00053 for( ;; ) 00054 { 00055 ( void )eMBPoll( ); 00056 00057 /* Here we simply count the number of poll cycles. */ 00058 usRegInputBuf[0]++; 00059 } 00060 } 00061 00062 eMBErrorCode 00063 eMBRegInputCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs ) 00064 { 00065 eMBErrorCode eStatus = MB_ENOERR ; 00066 int iRegIndex; 00067 00068 if( ( usAddress >= REG_INPUT_START ) 00069 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00070 { 00071 iRegIndex = ( int )( usAddress - usRegInputStart ); 00072 while( usNRegs > 0 ) 00073 { 00074 *pucRegBuffer++ = 00075 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00076 *pucRegBuffer++ = 00077 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00078 iRegIndex++; 00079 usNRegs--; 00080 } 00081 } 00082 else 00083 { 00084 eStatus = MB_ENOREG ; 00085 } 00086 00087 return eStatus; 00088 } 00089 00090 eMBErrorCode 00091 eMBRegHoldingCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs, eMBRegisterMode eMode ) 00092 { 00093 eMBErrorCode eStatus = MB_ENOERR ; 00094 int iRegIndex; 00095 00096 if (eMode == MB_REG_READ ) 00097 { 00098 if( ( usAddress >= REG_INPUT_START ) 00099 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00100 { 00101 iRegIndex = ( int )( usAddress - usRegInputStart ); 00102 while( usNRegs > 0 ) 00103 { 00104 *pucRegBuffer++ = 00105 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00106 *pucRegBuffer++ = 00107 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00108 iRegIndex++; 00109 usNRegs--; 00110 } 00111 } 00112 } 00113 00114 if (eMode == MB_REG_WRITE ) 00115 { 00116 if( ( usAddress >= REG_INPUT_START ) 00117 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00118 { 00119 iRegIndex = ( int )( usAddress - usRegInputStart ); 00120 while( usNRegs > 0 ) 00121 { 00122 usRegInputBuf[iRegIndex] = ((unsigned int) *pucRegBuffer << 8) | ((unsigned int) *(pucRegBuffer+1)); 00123 pucRegBuffer+=2; 00124 iRegIndex++; 00125 usNRegs--; 00126 } 00127 } 00128 } 00129 00130 return eStatus; 00131 } 00132 00133 00134 eMBErrorCode 00135 eMBRegCoilsCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNCoils, 00136 eMBRegisterMode eMode ) 00137 { 00138 return MB_ENOREG ; 00139 } 00140 00141 eMBErrorCode 00142 eMBRegDiscreteCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNDiscrete ) 00143 { 00144 return MB_ENOREG ; 00145 } 00146
Generated on Sun Jul 17 2022 02:04:12 by
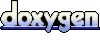