mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
Low level interface to the low power ticker of a target. More...
Modules | |
Low Power Ticker tests | |
Tests to validate the proper implementation of the low power ticker. | |
Ticker Hal | |
Low level interface to the ticker of a target. | |
Ticker Tests | |
Tests to validate the proper implementation of a ticker. | |
Functions | |
ticker_irq_handler_type | set_lp_ticker_irq_handler (ticker_irq_handler_type ticker_irq_handler) |
Set low power ticker IRQ handler. | |
const ticker_data_t * | get_lp_ticker_data (void) |
Get low power ticker's data. | |
void | lp_ticker_irq_handler (void) |
The wrapper for ticker_irq_handler, to pass lp ticker's data. | |
void | lp_ticker_init (void) |
Initialize the low power ticker. | |
void | lp_ticker_free (void) |
Deinitialize the lower power ticker. | |
uint32_t | lp_ticker_read (void) |
Read the current tick. | |
void | lp_ticker_set_interrupt (timestamp_t timestamp) |
Set interrupt for specified timestamp. | |
void | lp_ticker_disable_interrupt (void) |
Disable low power ticker interrupt. | |
void | lp_ticker_clear_interrupt (void) |
Clear the low power ticker interrupt. | |
void | lp_ticker_fire_interrupt (void) |
Set pending interrupt that should be fired right away. | |
const ticker_info_t * | lp_ticker_get_info (void) |
Get frequency and counter bits of this ticker. |
Detailed Description
Low level interface to the low power ticker of a target.
# Defined behavior * Has a reported frequency between 4KHz and 64KHz - verified by lp_ticker_info_test * Has a counter that is at least 12 bits wide - verified by lp_ticker_info_test * Continues operating in deep sleep mode - verified by lp_ticker_deepsleep_test * All behavior defined by the ticker specification
# Undefined behavior * See the ticker specification * Calling any function other than lp_ticker_init after calling lp_ticker_free
# Potential bugs * Glitches due to ripple counter - Verified by lp_ticker_glitch_test
- See also:
- Low Power Ticker tests
Function Documentation
const ticker_data_t * get_lp_ticker_data | ( | void | ) |
Get low power ticker's data.
- Returns:
- The low power ticker data
Definition at line 42 of file mbed_lp_ticker_api.c.
void lp_ticker_clear_interrupt | ( | void | ) |
Clear the low power ticker interrupt.
Pseudo Code:
void lp_ticker_clear_interrupt(void) { // Write to the ICR (interrupt clear register) of the LPTMR LPTMR_ICR = LPTMR_ICR_COMPARE_Msk; }
void lp_ticker_disable_interrupt | ( | void | ) |
Disable low power ticker interrupt.
Pseudo Code:
void lp_ticker_disable_interrupt(void) { // Disable the compare interrupt LPTMR_CTRL &= ~LPTMR_CTRL_COMPARE_ENABLE_Msk; }
void lp_ticker_fire_interrupt | ( | void | ) |
Set pending interrupt that should be fired right away.
Pseudo Code:
void lp_ticker_fire_interrupt(void) { NVIC_SetPendingIRQ(LPTMR_IRQn); }
void lp_ticker_free | ( | void | ) |
Deinitialize the lower power ticker.
Powerdown the lp ticker in preparation for sleep, powerdown, or reset.
After calling this function no other ticker functions should be called except lp_ticker_init(). Calling any function other than init after freeing is undefined.
- Note:
- This function stops the ticker from counting.
const ticker_info_t * lp_ticker_get_info | ( | void | ) |
Get frequency and counter bits of this ticker.
Pseudo Code:
const ticker_info_t* lp_ticker_get_info() { static const ticker_info_t info = { 32768, // 32KHz 16 // 16 bit counter }; return &info; }
void lp_ticker_init | ( | void | ) |
Initialize the low power ticker.
Initialize or re-initialize the ticker. This resets all the clocking and prescaler registers, along with disabling the compare interrupt.
Pseudo Code:
void lp_ticker_init() { // Enable clock gate so processor can read LPTMR registers POWER_CTRL |= POWER_CTRL_LPTMR_Msk; // Disable the timer and ensure it is powered down LPTMR_CTRL &= ~(LPTMR_CTRL_ENABLE_Msk | LPTMR_CTRL_COMPARE_ENABLE_Msk); // Configure divisors - no division necessary LPTMR_PRESCALE = 0; LPTMR_CTRL |= LPTMR_CTRL_ENABLE_Msk; // Install the interrupt handler NVIC_SetVector(LPTMR_IRQn, (uint32_t)lp_ticker_irq_handler); NVIC_EnableIRQ(LPTMR_IRQn); }
void lp_ticker_irq_handler | ( | void | ) |
The wrapper for ticker_irq_handler, to pass lp ticker's data.
Definition at line 60 of file mbed_lp_ticker_api.c.
uint32_t lp_ticker_read | ( | void | ) |
Read the current tick.
If no rollover has occurred, the seconds passed since lp_ticker_init() was called can be found by dividing the ticks returned by this function by the frequency returned by lp_ticker_get_info.
- Returns:
- The current timer's counter value in ticks
Pseudo Code:
uint32_t lp_ticker_read() { uint16_t count; uint16_t last_count; // Loop until the same tick is read twice since this // is ripple counter on a different clock domain. count = LPTMR_COUNT; do { last_count = count; count = LPTMR_COUNT; } while (last_count != count); return count; }
void lp_ticker_set_interrupt | ( | timestamp_t | timestamp ) |
Set interrupt for specified timestamp.
- Parameters:
-
timestamp The time in ticks to be set
- Note:
- no special handling needs to be done for times in the past as the common timer code will detect this and call lp_ticker_fire_interrupt() if this is the case
- calling this function with timestamp of more than the supported number of bits returned by lp_ticker_get_info results in undefined behavior.
Pseudo Code:
void lp_ticker_set_interrupt(timestamp_t timestamp) { LPTMR_COMPARE = timestamp; LPTMR_CTRL |= LPTMR_CTRL_COMPARE_ENABLE_Msk; }
ticker_irq_handler_type set_lp_ticker_irq_handler | ( | ticker_irq_handler_type | ticker_irq_handler ) |
Set low power ticker IRQ handler.
- Parameters:
-
ticker_irq_handler IRQ handler to be connected
- Returns:
- previous ticker IRQ handler
- Note:
- by default IRQ handler is set to ticker_irq_handler
- this function is primarily for testing purposes and it's not required part of HAL implementation
Definition at line 51 of file mbed_lp_ticker_api.c.
Generated on Tue Jul 12 2022 20:41:16 by
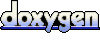