
RAMDisk example for the USBFileSystem
Dependencies: mbed USBFileSystem
Fork of USBFileSystem_RAMDISK_HelloWorld by
main.cpp
00001 /* 00002 This program is based on Richard Green's RAM_DISK program for the KL25Z 00003 00004 So all hail the Hypnotoad!!!! 00005 00006 (And Richard Green, but only after the Hypnotoad) 00007 */ 00008 00009 00010 00011 #include "mbed.h" 00012 #include "USBMSD_Ram.h" 00013 00014 DigitalOut myled(LED1); 00015 USBMSD_Ram ram; 00016 00017 void usbCallback(bool available) 00018 { 00019 if (available) { 00020 FILE *fp = fopen("/USB/IN.txt", "r"); 00021 char buffer[100]; 00022 fgets (buffer, 100, fp); 00023 printf("%s\r\n", buffer); 00024 fclose(fp); 00025 } 00026 } 00027 00028 int main() 00029 { 00030 ram.attachUSB(&usbCallback); 00031 00032 wait(0.1); 00033 printf("Hello World!\r\n"); 00034 00035 //Connect USB 00036 ram.connect(); 00037 00038 FILE* fp; 00039 char buffer[101]; 00040 printf("Type your text here! (100 max length)\n"); 00041 while(1) { 00042 gets(buffer); 00043 myled = !myled; 00044 00045 //Open file for write, keep trying until we succeed 00046 //This is needed because if USB happens to be writing it will fail 00047 while(1) { 00048 fp = fopen("/USB/OUT.txt", "w"); 00049 if (fp != NULL) 00050 break; 00051 } 00052 fprintf(fp, buffer); 00053 fclose(fp); 00054 00055 } 00056 00057 }
Generated on Fri Jul 15 2022 02:17:19 by
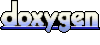