Forked MMA7660 , extend implementation by using i2c asynch API, to sleep while waiting for transfer -> blocking asynch :-D
Fork of MMA7660 by
MMA7660.h
00001 /* Copyright (c) <year> <copyright holders>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "mbed.h" 00020 00021 00022 #ifndef MMA7660_H 00023 #define MMA7660_H 00024 00025 #define MMA7660_ADDRESS 0x98 00026 #define MMA7660_SENSITIVITY 21.33 00027 00028 #define MMA7660_XOUT_R 0x00 00029 #define MMA7660_YOUT_R 0x01 00030 #define MMA7660_ZOUT_R 0x02 00031 #define MMA7660_TILT_R 0x03 00032 #define MMA7660_INT_R 0x06 00033 #define MMA7660_MODE_R 0x07 00034 #define MMA7660_SR_R 0x08 00035 00036 00037 /** An interface for the MMA7660 triple axis accelerometer 00038 * 00039 * @code 00040 * //Uses the measured z-acceleration to drive leds 2 and 3 of the mbed 00041 * 00042 * #include "mbed.h" 00043 * #include "MMA7660.h" 00044 * 00045 * MMA7660 MMA(p28, p27); 00046 * 00047 * DigitalOut connectionLed(LED1); 00048 * PwmOut Zaxis_p(LED2); 00049 * PwmOut Zaxis_n(LED3); 00050 * 00051 * int main() { 00052 * if (MMA.testConnection()) 00053 * connectionLed = 1; 00054 * 00055 * while(1) { 00056 * Zaxis_p = MMA.z(); 00057 * Zaxis_n = -MMA.z(); 00058 * } 00059 * 00060 * } 00061 * @endcode 00062 */ 00063 class MMA7660 00064 { 00065 public: 00066 /** 00067 * The 6 different orientations and unknown 00068 * 00069 * Up & Down = X-axis 00070 * Right & Left = Y-axis 00071 * Back & Front = Z-axis 00072 * 00073 */ 00074 enum Orientation {Up, Down, 00075 Right, Left, 00076 Back, Front, 00077 Unknown 00078 }; 00079 00080 /** 00081 * Creates a new MMA7660 object 00082 * 00083 * @param sda - I2C data pin 00084 * @param scl - I2C clock pin 00085 * @param active - true (default) to enable the device, false to keep it standby 00086 * @param asynch - use asynch i2c - the API is blocking which does not break the current app 00087 */ 00088 MMA7660(PinName sda, PinName scl, bool active = true, bool asynch = false); 00089 00090 /** 00091 * Tests if communication is possible with the MMA7660 00092 * 00093 * Because the MMA7660 lacks a WHO_AM_I register, this function can only check 00094 * if there is an I2C device that responds to the MMA7660 address 00095 * 00096 * @param return - true for successfull connection, false for no connection 00097 */ 00098 bool testConnection( void ); 00099 00100 /** 00101 * Sets the active state of the MMA7660 00102 * 00103 * Note: This is unrelated to awake/sleep mode 00104 * 00105 * @param state - true for active, false for standby 00106 */ 00107 void setActive( bool state); 00108 00109 /** 00110 * Reads acceleration data from the sensor 00111 * 00112 * When the parameter is a pointer to an integer array it will be the raw data. 00113 * When it is a pointer to a float array it will be the acceleration in g's 00114 * 00115 * @param data - pointer to array with length 3 where the acceleration data will be stored, X-Y-Z 00116 */ 00117 void readData( int *data); 00118 void readData( float *data); 00119 00120 /** 00121 * Get X-data 00122 * 00123 * @param return - X-acceleration in g's 00124 */ 00125 float x( void ); 00126 00127 /** 00128 * Get Y-data 00129 * 00130 * @param return - Y-acceleration in g's 00131 */ 00132 float y( void ); 00133 00134 /** 00135 * Get Z-data 00136 * 00137 * @param return - Z-acceleration in g's 00138 */ 00139 float z( void ); 00140 00141 /** 00142 * Sets the active samplerate 00143 * 00144 * The entered samplerate will be rounded to nearest supported samplerate. 00145 * Supported samplerates are: 120 - 64 - 32 - 16 - 8 - 4 - 2 - 1 samples/second. 00146 * 00147 * @param samplerate - the samplerate that will be set 00148 */ 00149 void setSampleRate(int samplerate); 00150 00151 /** 00152 * Returns if it is on its front, back, or unknown side 00153 * 00154 * This is read from MMA7760s registers, page 12 of datasheet 00155 * 00156 * @param return - Front, Back or Unknown orientation 00157 */ 00158 Orientation getSide( void ); 00159 00160 /** 00161 * Returns if it is on it left, right, down or up side 00162 * 00163 * This is read from MMA7760s registers, page 12 of datasheet 00164 * 00165 * @param return - Left, Right, Down, Up or Unknown orientation 00166 */ 00167 Orientation getOrientation ( void ); 00168 00169 00170 private: 00171 00172 void callback(int event); 00173 00174 /** 00175 * Writes data to the device 00176 * 00177 * @param adress - register address to write to 00178 * @param data - data to write 00179 */ 00180 void write( char address, char data); 00181 00182 /** 00183 * Read data from the device 00184 * 00185 * @param adress - register address to write to 00186 * @return - data from the register specified by RA 00187 */ 00188 char read( char adress); 00189 00190 /** 00191 * Read multiple regigsters from the device, more efficient than using multiple normal reads. 00192 * 00193 * @param adress - register address to write to 00194 * @param length - number of bytes to read 00195 * @param data - pointer where the data needs to be written to 00196 */ 00197 void read( char adress, char *data, int length); 00198 00199 /** 00200 * Reads single axis 00201 */ 00202 float getSingle(int number); 00203 00204 I2C _i2c; 00205 bool active; 00206 float samplerate; 00207 volatile bool callback_done; 00208 event_callback_t event; 00209 const bool asynch; 00210 }; 00211 00212 00213 #endif
Generated on Wed Jul 13 2022 08:47:22 by
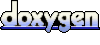