1-Wire® library for mbed. Complete 1-Wire library that supports our silicon masters along with a bit-bang master on the MAX32600MBED platform with one common interface for mbed. Slave support has also been included and more slaves will be added as time permits.
Dependents: MAXREFDES131_Qt_Demo MAX32630FTHR_iButton_uSD_Logger MAX32630FTHR_DS18B20_uSD_Logger MAXREFDES130_131_Demo ... more
DS2482SingleChannel Class Reference
DS2482_100 I2C to 1-wire Master. More...
#include <DS2482SingleChannel.h>
Inherits OneWire::DS248x.
Public Types | |
enum | I2CAddress |
Valid slave addresses for DS2482-100. More... | |
enum | Register |
Device register pointers. More... | |
enum | OWSpeed |
Speed of the 1-Wire bus. More... | |
enum | OWLevel |
Level of the 1-Wire bus. More... | |
enum | SearchDirection |
Search direction for the triplet operation. More... | |
enum | CmdResult |
Result of all 1-Wire commands. More... | |
Public Member Functions | |
DS2482SingleChannel (mbed::I2C &i2c_bus, uint8_t adrs) | |
Construct to use an existing I2C interface. | |
OneWireMaster::CmdResult | reset (void) |
Performs a soft reset on the DS248x. | |
OneWireMaster::CmdResult | writeConfig (const Config &config, bool verify) |
Write a new configuration to the DS248x. | |
Config | currentConfig () const |
Read the current DS248x configuration. | |
OneWireMaster::CmdResult | readRegister (Register reg, uint8_t &buf, bool skipSetPointer=false) const |
Reads a register from the DS248x. | |
virtual OneWireMaster::CmdResult | OWInitMaster () |
virtual OneWireMaster::CmdResult | OWTriplet (SearchDirection &searchDirection, uint8_t &sbr, uint8_t &tsb) |
virtual OneWireMaster::CmdResult | OWReset () |
Reset all of the devices on the 1-Wire bus and check for a presence pulse. | |
virtual OneWireMaster::CmdResult | OWTouchBitSetLevel (uint8_t &sendRecvBit, OWLevel afterLevel) |
Send and receive one bit of communication and set a new level on the 1-Wire bus. | |
virtual OneWireMaster::CmdResult | OWReadByteSetLevel (uint8_t &recvByte, OWLevel afterLevel) |
Receive one byte of communication and set a new level on the 1-Wire bus. | |
virtual OneWireMaster::CmdResult | OWWriteByteSetLevel (uint8_t sendByte, OWLevel afterLevel) |
Send one byte of communication and set a new level on the 1-Wire bus. | |
virtual OneWireMaster::CmdResult | OWSetSpeed (OWSpeed newSpeed) |
Set the 1-Wire bus communication speed. | |
virtual OneWireMaster::CmdResult | OWSetLevel (OWLevel newLevel) |
Set the 1-Wire bus level. | |
virtual CmdResult | OWWriteBlock (const uint8_t *sendBuf, size_t sendLen) |
Send a block of communication on the 1-Wire bus. | |
virtual CmdResult | OWReadBlock (uint8_t *recvBuf, size_t recvLen) |
Receive a block of communication on the 1-Wire bus. | |
CmdResult | OWWriteBitSetLevel (uint8_t sendBit, OWLevel afterLevel) |
Send one bit of communication and set a new level on the 1-Wire bus. | |
CmdResult | OWReadBitSetLevel (uint8_t &recvBit, OWLevel afterLevel) |
Receive one bit of communication and set a new level on the 1-Wire bus. | |
Protected Member Functions | |
OneWireMaster::CmdResult | sendCommand (Command cmd) const |
OneWireMaster::CmdResult | sendCommand (Command cmd, uint8_t param) const |
Detailed Description
DS2482_100 I2C to 1-wire Master.
Definition at line 44 of file DS2482SingleChannel.h.
Member Enumeration Documentation
enum CmdResult [inherited] |
Result of all 1-Wire commands.
Definition at line 67 of file OneWireMaster.h.
enum I2CAddress |
Valid slave addresses for DS2482-100.
Definition at line 48 of file DS2482SingleChannel.h.
enum OWLevel [inherited] |
Level of the 1-Wire bus.
Definition at line 53 of file OneWireMaster.h.
enum OWSpeed [inherited] |
Speed of the 1-Wire bus.
Definition at line 46 of file OneWireMaster.h.
enum SearchDirection [inherited] |
Search direction for the triplet operation.
Definition at line 60 of file OneWireMaster.h.
Constructor & Destructor Documentation
DS2482SingleChannel | ( | mbed::I2C & | i2c_bus, |
uint8_t | adrs | ||
) |
Construct to use an existing I2C interface.
- Parameters:
-
i2c_bus Configured I2C communication interface for DS248x. adrs I2C bus address of the DS248x in mbed format.
Definition at line 59 of file DS2482SingleChannel.h.
Member Function Documentation
Config currentConfig | ( | ) | const [inherited] |
OneWireMaster::CmdResult OWInitMaster | ( | void | ) | [virtual, inherited] |
Performs a device reset followed by writing the configuration byte to default values: 1-Wire Speed Standard Strong Pullup Off 1-Wire Powerdown Off Active Pullup On
Implements OneWireMaster.
Definition at line 98 of file DS248x.cpp.
Receive one bit of communication and set a new level on the 1-Wire bus.
- Parameters:
-
[out] sendRecvBit Read data from 1-Wire bus will be returned in lsb. afterLevel Level to set the 1-Wire bus to after communication.
Definition at line 143 of file OneWireMaster.h.
OneWireMaster::CmdResult OWReadBlock | ( | uint8_t * | recvBuf, |
size_t | recvLen | ||
) | [virtual, inherited] |
Receive a block of communication on the 1-Wire bus.
- Parameters:
-
[out] recvBuf Buffer to receive the data from the 1-Wire bus. recvLen Length of the buffer to receive.
Reimplemented in DS2465.
Definition at line 53 of file OneWireMaster.cpp.
OneWireMaster::CmdResult OWReadByteSetLevel | ( | uint8_t & | recvByte, |
OWLevel | afterLevel | ||
) | [virtual, inherited] |
Receive one byte of communication and set a new level on the 1-Wire bus.
- Parameters:
-
recvByte Buffer to receive the data from the 1-Wire bus. afterLevel Level to set the 1-Wire bus to after communication.
Implements OneWireMaster.
Definition at line 265 of file DS248x.cpp.
OneWireMaster::CmdResult OWReset | ( | ) | [virtual, inherited] |
Reset all of the devices on the 1-Wire bus and check for a presence pulse.
- Returns:
- OperationFailure if reset was performed but no presence pulse was detected.
Implements OneWireMaster.
Definition at line 175 of file DS248x.cpp.
OneWireMaster::CmdResult OWSetLevel | ( | OWLevel | newLevel ) | [virtual, inherited] |
OneWireMaster::CmdResult OWSetSpeed | ( | OWSpeed | newSpeed ) | [virtual, inherited] |
Set the 1-Wire bus communication speed.
Implements OneWireMaster.
Definition at line 305 of file DS248x.cpp.
OneWireMaster::CmdResult OWTouchBitSetLevel | ( | uint8_t & | sendRecvBit, |
OWLevel | afterLevel | ||
) | [virtual, inherited] |
Send and receive one bit of communication and set a new level on the 1-Wire bus.
- Parameters:
-
[in,out] sendRecvBit Buffer containing the bit to send on 1-Wire bus in lsb. Read data from 1-Wire bus will be returned in lsb. afterLevel Level to set the 1-Wire bus to after communication.
Implements OneWireMaster.
Definition at line 205 of file DS248x.cpp.
OneWireMaster::CmdResult OWTriplet | ( | SearchDirection & | searchDirection, |
uint8_t & | sbr, | ||
uint8_t & | tsb | ||
) | [virtual, inherited] |
- Note:
- Perform a 1-Wire triplet using the DS248x command.
Reimplemented from OneWireMaster.
Definition at line 149 of file DS248x.cpp.
Send one bit of communication and set a new level on the 1-Wire bus.
- Parameters:
-
sendBit Buffer containing the bit to send on 1-Wire bus in lsb. afterLevel Level to set the 1-Wire bus to after communication.
Definition at line 138 of file OneWireMaster.h.
OneWireMaster::CmdResult OWWriteBlock | ( | const uint8_t * | sendBuf, |
size_t | sendLen | ||
) | [virtual, inherited] |
Send a block of communication on the 1-Wire bus.
- Parameters:
-
[in] sendBuf Buffer to send on the 1-Wire bus. sendLen Length of the buffer to send.
Reimplemented in DS2465.
Definition at line 37 of file OneWireMaster.cpp.
OneWireMaster::CmdResult OWWriteByteSetLevel | ( | uint8_t | sendByte, |
OWLevel | afterLevel | ||
) | [virtual, inherited] |
Send one byte of communication and set a new level on the 1-Wire bus.
- Parameters:
-
sendByte Byte to send on the 1-Wire bus. afterLevel Level to set the 1-Wire bus to after communication.
Implements OneWireMaster.
Definition at line 239 of file DS248x.cpp.
OneWireMaster::CmdResult readRegister | ( | Register | reg, |
uint8_t & | buf, | ||
bool | skipSetPointer = false |
||
) | const [inherited] |
Reads a register from the DS248x.
- Parameters:
-
reg Register to read from. [out] buf Buffer to hold read data. skipSetPointer Assume that the read pointer is already set to the correct register.
Definition at line 361 of file DS248x.cpp.
OneWireMaster::CmdResult reset | ( | void | ) | [inherited] |
Performs a soft reset on the DS248x.
- Note:
- This is note a 1-Wire Reset.
Definition at line 115 of file DS248x.cpp.
OneWireMaster::CmdResult sendCommand | ( | Command | cmd, |
uint8_t | param | ||
) | const [protected, inherited] |
- Note:
- Allow marking const since not public.
Definition at line 436 of file DS248x.cpp.
OneWireMaster::CmdResult sendCommand | ( | Command | cmd ) | const [protected, inherited] |
- Note:
- Allow marking const since not public.
Definition at line 422 of file DS248x.cpp.
OneWireMaster::CmdResult writeConfig | ( | const Config & | config, |
bool | verify | ||
) | [inherited] |
Write a new configuration to the DS248x.
- Parameters:
-
[in] config New configuration to write. verify Verify that the configuration was written successfully.
Definition at line 331 of file DS248x.cpp.
Generated on Tue Jul 12 2022 15:46:21 by
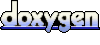