1-Wire® library for mbed. Complete 1-Wire library that supports our silicon masters along with a bit-bang master on the MAX32600MBED platform with one common interface for mbed. Slave support has also been included and more slaves will be added as time permits.
Dependents: MAXREFDES131_Qt_Demo MAX32630FTHR_iButton_uSD_Logger MAX32630FTHR_DS18B20_uSD_Logger MAXREFDES130_131_Demo ... more
OneWireMaster Class Reference
Base class for all 1-Wire Masters. More...
#include <OneWireMaster.h>
Inherited by DS2465, DS2480B, and DS248x.
Public Types | |
enum | OWSpeed |
Speed of the 1-Wire bus. More... | |
enum | OWLevel |
Level of the 1-Wire bus. More... | |
enum | SearchDirection |
Search direction for the triplet operation. More... | |
enum | CmdResult |
Result of all 1-Wire commands. More... | |
Public Member Functions | |
virtual | ~OneWireMaster () |
Allow freeing through a base class pointer. | |
virtual CmdResult | OWInitMaster ()=0 |
Initialize a master for use. | |
virtual CmdResult | OWReset ()=0 |
Reset all of the devices on the 1-Wire bus and check for a presence pulse. | |
virtual CmdResult | OWTouchBitSetLevel (uint8_t &sendRecvBit, OWLevel afterLevel)=0 |
Send and receive one bit of communication and set a new level on the 1-Wire bus. | |
virtual CmdResult | OWWriteByteSetLevel (uint8_t sendByte, OWLevel afterLevel)=0 |
Send one byte of communication and set a new level on the 1-Wire bus. | |
virtual CmdResult | OWReadByteSetLevel (uint8_t &recvByte, OWLevel afterLevel)=0 |
Receive one byte of communication and set a new level on the 1-Wire bus. | |
virtual CmdResult | OWWriteBlock (const uint8_t *sendBuf, size_t sendLen) |
Send a block of communication on the 1-Wire bus. | |
virtual CmdResult | OWReadBlock (uint8_t *recvBuf, size_t recvLen) |
Receive a block of communication on the 1-Wire bus. | |
virtual CmdResult | OWSetSpeed (OWSpeed newSpeed)=0 |
Set the 1-Wire bus communication speed. | |
virtual CmdResult | OWSetLevel (OWLevel newLevel)=0 |
Set the 1-Wire bus level. | |
virtual CmdResult | OWTriplet (SearchDirection &searchDirection, uint8_t &sbr, uint8_t &tsb) |
1-Wire Triplet operation. | |
CmdResult | OWWriteBitSetLevel (uint8_t sendBit, OWLevel afterLevel) |
Send one bit of communication and set a new level on the 1-Wire bus. | |
CmdResult | OWReadBitSetLevel (uint8_t &recvBit, OWLevel afterLevel) |
Receive one bit of communication and set a new level on the 1-Wire bus. |
Detailed Description
Base class for all 1-Wire Masters.
Definition at line 42 of file OneWireMaster.h.
Member Enumeration Documentation
enum CmdResult |
Result of all 1-Wire commands.
Definition at line 67 of file OneWireMaster.h.
enum OWLevel |
Level of the 1-Wire bus.
Definition at line 53 of file OneWireMaster.h.
enum OWSpeed |
Speed of the 1-Wire bus.
Definition at line 46 of file OneWireMaster.h.
enum SearchDirection |
Search direction for the triplet operation.
Definition at line 60 of file OneWireMaster.h.
Constructor & Destructor Documentation
virtual ~OneWireMaster | ( | ) | [virtual] |
Allow freeing through a base class pointer.
Definition at line 77 of file OneWireMaster.h.
Member Function Documentation
virtual CmdResult OWInitMaster | ( | ) | [pure virtual] |
Receive one bit of communication and set a new level on the 1-Wire bus.
- Parameters:
-
[out] sendRecvBit Read data from 1-Wire bus will be returned in lsb. afterLevel Level to set the 1-Wire bus to after communication.
Definition at line 143 of file OneWireMaster.h.
OneWireMaster::CmdResult OWReadBlock | ( | uint8_t * | recvBuf, |
size_t | recvLen | ||
) | [virtual] |
Receive a block of communication on the 1-Wire bus.
- Parameters:
-
[out] recvBuf Buffer to receive the data from the 1-Wire bus. recvLen Length of the buffer to receive.
Reimplemented in DS2465.
Definition at line 53 of file OneWireMaster.cpp.
virtual CmdResult OWReset | ( | ) | [pure virtual] |
Send and receive one bit of communication and set a new level on the 1-Wire bus.
- Parameters:
-
[in,out] sendRecvBit Buffer containing the bit to send on 1-Wire bus in lsb. Read data from 1-Wire bus will be returned in lsb. afterLevel Level to set the 1-Wire bus to after communication.
OneWireMaster::CmdResult OWTriplet | ( | SearchDirection & | searchDirection, |
uint8_t & | sbr, | ||
uint8_t & | tsb | ||
) | [virtual] |
1-Wire Triplet operation.
Perform one bit of a 1-Wire search. This command does two read bits and one write bit. The write bit is either the default direction (all device have same bit) or in case of a discrepancy, the 'searchDirection' parameter is used.
- Parameters:
-
[in,out] searchDirection Input with desired direction in case both read bits are zero. Output with direction taken based on read bits. [out] sbr Bit result of first read operation. [out] tsb Bit result of second read operation.
Reimplemented in DS2465, and DS248x.
Definition at line 69 of file OneWireMaster.cpp.
Send one bit of communication and set a new level on the 1-Wire bus.
- Parameters:
-
sendBit Buffer containing the bit to send on 1-Wire bus in lsb. afterLevel Level to set the 1-Wire bus to after communication.
Definition at line 138 of file OneWireMaster.h.
OneWireMaster::CmdResult OWWriteBlock | ( | const uint8_t * | sendBuf, |
size_t | sendLen | ||
) | [virtual] |
Send a block of communication on the 1-Wire bus.
- Parameters:
-
[in] sendBuf Buffer to send on the 1-Wire bus. sendLen Length of the buffer to send.
Reimplemented in DS2465.
Definition at line 37 of file OneWireMaster.cpp.
Generated on Tue Jul 12 2022 15:46:21 by
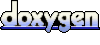