Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Dependents: testUniGraphic_150217 maze_TFT_MMA8451Q TFT_test_frdm-kl25z TFT_test_NUCLEO-F411RE ... more
I2C_bus Class Reference
I2C interface. More...
#include <I2C_bus.h>
Inherits Protocols.
Public Member Functions | |
I2C_bus (int Hz, int address, PinName sda, PinName scl) | |
Create an I2C display interface. | |
Protected Member Functions | |
virtual void | wr_cmd8 (unsigned char cmd) |
Send 8bit command to display controller. | |
virtual void | wr_data8 (unsigned char data) |
Send 8bit data to display controller. | |
virtual void | wr_cmd16 (unsigned short cmd) |
Send 2x8bit command to display controller. | |
virtual void | wr_data16 (unsigned short data) |
Send 2x8bit data to display controller. | |
virtual void | wr_gram (unsigned short data) |
Send 16bit pixeldata to display controller. | |
virtual void | wr_gram (unsigned short data, unsigned int count) |
Send same 16bit pixeldata to display controller multiple times. | |
virtual void | wr_grambuf (unsigned short *data, unsigned int lenght) |
Send array of pixeldata shorts to display controller. | |
virtual unsigned short | rd_gram (bool convert) |
Read 16bit pixeldata from display controller (with dummy cycle) | |
virtual unsigned int | rd_reg_data32 (unsigned char reg) |
Read 4x8bit register data ( reading from display ia I2C is not implemented in most controllers ! | |
virtual unsigned int | rd_extcreg_data32 (unsigned char reg, unsigned char SPIreadenablecmd) |
Read 3x8bit ExtendedCommands register data reading from display ia I2C is not implemented in most controllers ! | |
virtual void | dummyread () |
ILI932x specific, does a dummy read cycle, number of bits is protocol dependent reading from display ia I2C is not implemented in most controllers ! | |
virtual void | reg_select (unsigned char reg, bool forread=false) |
ILI932x specific, select register for a successive write or read. | |
virtual void | reg_write (unsigned char reg, unsigned short data) |
ILI932x specific, write register with data. | |
virtual unsigned short | reg_read (unsigned char reg) |
ILI932x specific, read register. | |
virtual void | hw_reset () |
HW reset sequence (without display init commands) most I2C displays have no reset signal ! | |
virtual void | BusEnable (bool enable) |
Set ChipSelect high or low. |
Detailed Description
I2C interface.
Definition at line 9 of file I2C_bus.h.
Constructor & Destructor Documentation
I2C_bus | ( | int | Hz, |
int | address, | ||
PinName | sda, | ||
PinName | scl | ||
) |
Create an I2C display interface.
- Parameters:
-
I2C frquency I2C address I2C pin sda I2C pin scl
Definition at line 16 of file I2C_bus.cpp.
Member Function Documentation
void BusEnable | ( | bool | enable ) | [protected, virtual] |
Set ChipSelect high or low.
- Parameters:
-
enable 0/1 not implemented for I2C !
Implements Protocols.
Definition at line 98 of file I2C_bus.cpp.
void dummyread | ( | ) | [protected, virtual] |
ILI932x specific, does a dummy read cycle, number of bits is protocol dependent reading from display ia I2C is not implemented in most controllers !
Implements Protocols.
Definition at line 116 of file I2C_bus.cpp.
void hw_reset | ( | ) | [protected, virtual] |
HW reset sequence (without display init commands) most I2C displays have no reset signal !
Implements Protocols.
Definition at line 94 of file I2C_bus.cpp.
unsigned int rd_extcreg_data32 | ( | unsigned char | reg, |
unsigned char | SPIreadenablecmd | ||
) | [protected, virtual] |
Read 3x8bit ExtendedCommands register data reading from display ia I2C is not implemented in most controllers !
Implements Protocols.
Definition at line 111 of file I2C_bus.cpp.
unsigned short rd_gram | ( | bool | convert ) | [protected, virtual] |
Read 16bit pixeldata from display controller (with dummy cycle)
- Parameters:
-
convert true/false. Convert 18bit to 16bit, some controllers returns 18bit
- Returns:
- 16bit color
Implements Protocols.
Definition at line 120 of file I2C_bus.cpp.
unsigned int rd_reg_data32 | ( | unsigned char | reg ) | [protected, virtual] |
Read 4x8bit register data ( reading from display ia I2C is not implemented in most controllers !
Implements Protocols.
Definition at line 106 of file I2C_bus.cpp.
unsigned short reg_read | ( | unsigned char | reg ) | [protected, virtual] |
ILI932x specific, read register.
- Parameters:
-
reg register to be read
- Returns:
- 16bit register value not implemented for I2C !
Implements Protocols.
Definition at line 125 of file I2C_bus.cpp.
void reg_select | ( | unsigned char | reg, |
bool | forread = false |
||
) | [protected, virtual] |
ILI932x specific, select register for a successive write or read.
reading from display ia I2C is not implemented in most controllers !
Implements Protocols.
Definition at line 102 of file I2C_bus.cpp.
void reg_write | ( | unsigned char | reg, |
unsigned short | data | ||
) | [protected, virtual] |
ILI932x specific, write register with data.
- Parameters:
-
reg register to write data 16bit data not implemented for I2C !
Implements Protocols.
Definition at line 130 of file I2C_bus.cpp.
void wr_cmd16 | ( | unsigned short | cmd ) | [protected, virtual] |
Send 2x8bit command to display controller.
- Parameters:
-
cmd,: halfword to send
- Note:
- in SPI_16 mode a single 16bit transfer will be done
Implements Protocols.
Definition at line 35 of file I2C_bus.cpp.
void wr_cmd8 | ( | unsigned char | cmd ) | [protected, virtual] |
Send 8bit command to display controller.
- Parameters:
-
cmd,: byte to send
Implements Protocols.
Definition at line 24 of file I2C_bus.cpp.
void wr_data16 | ( | unsigned short | data ) | [protected, virtual] |
Send 2x8bit data to display controller.
- Parameters:
-
data,: halfword to send
- Note:
- in SPI_16 mode a single 16bit transfer will be done
Implements Protocols.
Definition at line 44 of file I2C_bus.cpp.
void wr_data8 | ( | unsigned char | data ) | [protected, virtual] |
Send 8bit data to display controller.
- Parameters:
-
data,: byte to send
Implements Protocols.
Definition at line 31 of file I2C_bus.cpp.
void wr_gram | ( | unsigned short | data ) | [protected, virtual] |
Send 16bit pixeldata to display controller.
- Parameters:
-
data,: halfword to send
Implements Protocols.
Definition at line 49 of file I2C_bus.cpp.
void wr_gram | ( | unsigned short | data, |
unsigned int | count | ||
) | [protected, virtual] |
Send same 16bit pixeldata to display controller multiple times.
- Parameters:
-
data,: halfword to send count,: how many
Implements Protocols.
Definition at line 54 of file I2C_bus.cpp.
void wr_grambuf | ( | unsigned short * | data, |
unsigned int | lenght | ||
) | [protected, virtual] |
Send array of pixeldata shorts to display controller.
- Parameters:
-
data,: unsigned short pixeldata array lenght,: lenght (in shorts)
Implements Protocols.
Definition at line 79 of file I2C_bus.cpp.
Generated on Tue Jul 12 2022 13:49:07 by
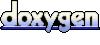