Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Dependents: testUniGraphic_150217 maze_TFT_MMA8451Q TFT_test_frdm-kl25z TFT_test_NUCLEO-F411RE ... more
I2C_bus.cpp
00001 /* mbed UniGraphic library - I2C protocol class 00002 * Copyright (c) 2017 Peter Drescher 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00006 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00007 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00008 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00009 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00010 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00011 * THE SOFTWARE. 00012 */ 00013 00014 #include "I2C_bus.h" 00015 00016 I2C_bus::I2C_bus(int Hz, int address, PinName sda, PinName scl) 00017 : _i2c(sda,scl) 00018 { 00019 _i2c.frequency(Hz); 00020 _address = address; 00021 //hw_reset(); 00022 } 00023 00024 void I2C_bus::wr_cmd8(unsigned char cmd) 00025 { 00026 char tmp[2]; 00027 tmp[0] = 0x00; //command 00028 tmp[1] = cmd; 00029 _i2c.write(_address,tmp,2); 00030 } 00031 void I2C_bus::wr_data8(unsigned char data) 00032 { 00033 _i2c.write(data); // write 8bit 00034 } 00035 void I2C_bus::wr_cmd16(unsigned short cmd) 00036 { 00037 char tmp[3]; 00038 tmp[0] = 00; //command 00039 tmp[1] = cmd>>8; 00040 tmp[2] = cmd&0xFF; 00041 00042 _i2c.write(_address,tmp,3); 00043 } 00044 void I2C_bus::wr_data16(unsigned short data) 00045 { 00046 _i2c.write(data>>8); // write 8bit 00047 _i2c.write(data&0xFF); // write 8bit 00048 } 00049 void I2C_bus::wr_gram(unsigned short data) 00050 { 00051 _i2c.write(data>>8); // write 8bit 00052 _i2c.write(data&0xFF); // write 8bit 00053 } 00054 void I2C_bus::wr_gram(unsigned short data, unsigned int count) 00055 { 00056 _i2c.start(); 00057 _i2c.write(_address); 00058 _i2c.write(0x40); // data continue 00059 if((data>>8)==(data&0xFF)) 00060 { 00061 count<<=1; 00062 while(count) 00063 { 00064 _i2c.write(data); // write 8bit 00065 count--; 00066 } 00067 } 00068 else 00069 { 00070 while(count) 00071 { 00072 _i2c.write(data>>8); // write 8bit 00073 _i2c.write(data&0xFF); // write 8bit 00074 count--; 00075 } 00076 } 00077 _i2c.stop(); 00078 } 00079 void I2C_bus::wr_grambuf(unsigned short* data, unsigned int lenght) 00080 { 00081 _i2c.start(); 00082 _i2c.write(_address); 00083 _i2c.write(0x40); // data continue 00084 while(lenght) 00085 { 00086 _i2c.write((*data)>>8); // write 8bit 00087 _i2c.write((*data)&0xFF); // write 8bit 00088 data++; 00089 lenght--; 00090 } 00091 _i2c.stop(); 00092 } 00093 00094 void I2C_bus::hw_reset() 00095 { 00096 00097 } 00098 void I2C_bus::BusEnable(bool enable) 00099 { 00100 } 00101 00102 void I2C_bus::reg_select(unsigned char reg, bool forread) 00103 { 00104 } 00105 00106 unsigned int I2C_bus::rd_reg_data32(unsigned char reg) 00107 { 00108 return 0; 00109 } 00110 00111 unsigned int I2C_bus::rd_extcreg_data32(unsigned char reg, unsigned char SPIreadenablecmd) 00112 { 00113 return 0; 00114 } 00115 00116 void I2C_bus::dummyread() 00117 { 00118 } 00119 00120 unsigned short I2C_bus::rd_gram(bool convert) 00121 { 00122 return (0); 00123 } 00124 00125 unsigned short I2C_bus::reg_read(unsigned char reg) 00126 { 00127 return (0); 00128 } 00129 00130 void I2C_bus::reg_write(unsigned char reg, unsigned short data) 00131 { 00132 }
Generated on Tue Jul 12 2022 13:49:06 by
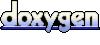