LM61 and TMP36 Analog Temperature Sensors
The LM61 is a low cost analog temperature sensor. In addition to surface mount, it is available in a TO-92 3 pin package that will plug directly into a breadboard. Cost for the LM61 can run well under $1 US. Accuracy runs around 2C or 1C for TMP36.
Hello World
Import programLM61
Analog Temperature Sensor Demo. See http://mbed.org/users/4180_1/notebook/lm61-analog-temperature-sensor/
Library
Import programLM61
Analog Temperature Sensor Demo. See http://mbed.org/users/4180_1/notebook/lm61-analog-temperature-sensor/
Pinout
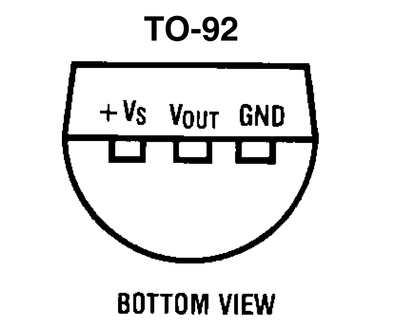
Datasheet
http://www.ti.com/lit/ds/symlink/lm61.pdfNotes
mbed | LM61 (or TMP36) | |
GND | GND | |
Vout(3.3V) | Vs | |
p15 | Vout |
Warning
Pay attention to the pinout show above. If you plug in the sensor backwards, it becomes a space heater for a few seconds and dies, or if you are lucky mbed's overcurrent circuit shuts things down. Keep the leads short to reduce analog noise on a breadboard. If a design turns on and off other high current external I/O devices, a (1-10uf?) decoupling capacitor across the analog sensor's power leads (located right at the sensor) can help filter out the transient switching noise on the power supply and produce more accurate and consistent readings.
Here is a simple code example showing how to setup and use a new C++ class for the LM61
LM61
//Print temperature from LM61 analog temperature sensor //Setup a new class for LM61 sensor class LM61 { public: LM61(PinName pin); LM61(); operator float (); float read(); private: //sets up the AnalogIn pin AnalogIn _pin; }; LM61::LM61(PinName pin) : _pin(pin) { // _pin(pin) means pass pin to the AnalogIn constructor } float LM61::read() { //convert sensor reading to temperature in degrees C return ((_pin.read()*3.3)-0.600)*100.0; } //overload of float conversion (avoids needing to type .read() in equations) LM61::operator float () { //convert sensor reading to temperature in degrees C return ((_pin.read()*3.3)-0.600)*100.0; } //use the new class to set p15 to analog input to read and convert LM61 sensor's voltage output LM61 mylm61(p15); //also setting unused analog input pins to digital outputs reduces A/D noise a bit //see http://mbed.org/users/chris/notebook/Getting-best-ADC-performance/ DigitalOut P16(p16); DigitalOut P17(p17); DigitalOut P18(p18); DigitalOut P19(p19); DigitalOut P20(p20); int main() { float tempC; while(1) { tempC = mylm61; printf("Float operator overloading avoids .read() above: T=%5.2F C \n\r", tempC); printf("As a printf arg it needs a float type convert: T=%5.2F C \n\r", float(mylm61)); printf("Or Use .read() instead: T=%5.2F C \n\r\n\r", mylm61.read()); wait(.5); } }
Code modified for TMP36
TMP36
#include "mbed.h" //Print temperature from TMP36 analog temperature sensor //Setup a new class for TMP36 sensor class TMP36 { public: TMP36(PinName pin); TMP36(); operator float (); float read(); private: //class sets up the AnalogIn pin AnalogIn _pin; }; TMP36::TMP36(PinName pin) : _pin(pin) { // _pin(pin) means pass pin to the AnalogIn constructor } float TMP36::read() { //convert sensor reading to temperature in degrees C return ((_pin.read()*3.3)-0.500)*100.0; } //overload of float conversion (avoids needing to type .read() in equations) TMP36::operator float () { //convert sensor reading to temperature in degrees C return ((_pin.read()*3.3)-0.500)*100.0; } //use the new class to set p15 to analog input to read and convert TMP36 sensor's voltage output TMP36 myTMP36(p15); //also setting unused analog input pins to digital outputs reduces A/D noise a bit //see http://mbed.org/users/chris/notebook/Getting-best-ADC-performance/ DigitalOut P16(p16); DigitalOut P17(p17); DigitalOut P18(p18); DigitalOut P19(p19); DigitalOut P20(p20); int main() { float tempC; while(1) { tempC = myTMP36; printf("Float operator overloading avoids .read(): T=%5.2F C \n\r", tempC); printf("A Printf arg needs a float type convert: T=%5.2F C \n\r", float(myTMP36)); printf("Or Use .read() instead: T=%5.2F C \n\r\n\r", myTMP36.read()); wait(.5); } }
Import programTemp36_HelloWorld_WIZwiki-W7500
Temp36 HelloWorld Example for WIZwiki-W7500
Temp36
/* Analog Input "Temp36" Temperature Sensor Example Program */ #include "mbed.h" // Initialize a pins to perform analog input fucntions AnalogIn ain(A0); // connect A0(WIZwiki-W7500) to Vout(Temp36) int main(void) { while (1) { float V = ain.read() * 3.3; // connect Vs(Temp36) to 3.3V(WIZwiki-W7500) //float V = ain.read() * 5; // connect Vs(Temp36) to 5V(WIZwiki-W7500) float tempC = (V-0.5) * 100; // calculate temperature C float tempF = (tempC * 9 / 5) + 32.0; // calculate temperature F printf("tempC value : %5.2f C \r\n", tempC); printf("tempF value : %5.2f F \r\n", tempF); wait(1.0); } }
The example code above displays the temperature from the sensor. From the datasheet, the output is assumed linear with Vout = 0.6 + 0.01*Tc. Since the analog input is scaled between 0 to 1.0 by AnalogIn(), it is multiplied by 3.3 to convert it back to a voltage reading, 0.6 is subtracted and it is multiplied by 100 to obtain the temperature in degrees C. A printf() then displays the temperature in degrees C and F on the PC. For the TMP36, change the voltage offset from 0.600 to 0.500 in the code example.
In a final product, additional steps can be taken to reduce and filter the noise. See http://mbed.org/users/chris/notebook/Getting-best-ADC-performance/ for some suggestions. Analog noise also tends to be a bit higher on some processors when they are running in debug mode according to cautionary notes in data sheets. Many mbed modules are in debug mode when operating over the USB cable. Some PCs also have more noise present on USB power pins.