
Retro Invaders a space invaders clone by Chris Favreau. Written for the RetroMbuino development board from outrageouscircuits.com for the game programming contest.
LCD_ST7735 Class Reference
LCD_ST7735 is a simple driver for the ST7735 LCD controller. More...
#include <LCD_ST7735.h>
Inherited by display.
Public Types | |
enum | Orientation { Rotate0, Rotate90, Rotate180, Rotate270 } |
Orientation of the display. More... | |
enum | PanelColorFilter { RGB = 0, BGR = 8 } |
Type of color filter of the panel. More... | |
Public Member Functions | |
LCD_ST7735 (PinName backlightPin, PinName resetPin, PinName dsPin, PinName mosiPin, PinName misoPin, PinName clkPin, PinName csPin, PanelColorFilter colorFilter=BGR) | |
Creates an instance of the LCD_ST7735 driver. | |
void | setOrientation (Orientation orientation, bool flip) |
Set the orientation of the display. | |
int | getWidth () |
Get the width of the display given the current orientation. | |
int | getHeight () |
Get the height of the display given the current orientation. | |
void | setBacklight (bool state) |
Control the display's backlight. | |
void | clearScreen (uint16_t color=0x0000) |
Clear the screen. | |
void | setPixel (int x, int y, uint16_t color) |
Set a pixel on the display to the specified color. | |
void | drawLine (int x1, int y1, int x2, int y2, uint16_t color) |
Draw a line on the display. | |
void | drawRect (int x1, int y1, int x2, int y2, uint16_t color) |
Draw a rectangle on the display. | |
void | drawCircle (int x, int y, int r, uint16_t color) |
Draw a circle on the display. | |
void | drawEllipse (int x, int y, int rx, int ry, uint16_t color) |
Draw an ellipse on the display. | |
void | fillRect (int x1, int y1, int x2, int y2, uint16_t fillColor) |
Draw a filled rectangle on the display. | |
void | fillRect (int x1, int y1, int x2, int y2, uint16_t borderColor, uint16_t fillColor) |
Draw a filled rectangle on the display. | |
void | fillCircle (int x, int y, int r, uint16_t borderColor, uint16_t fillColor) |
Draw a filled circle on the display. | |
void | fillEllipse (int x, int y, int rx, int ry, uint16_t borderColor, uint16_t fillColor) |
Draw a filled ellipse on the display. | |
void | drawBitmap (int x, int y, const uint16_t *pbmp) |
Draw a bitmap on the screen. | |
void | drawBitmap (int x, int y, const uint16_t *pbmp, int srcX, int srcY, int srcWidth, int srcHeight) |
Extracts a portion of a bitmap and draws it on the screen. | |
void | setForegroundColor (uint16_t color) |
Set the foreground color used to render text. | |
void | setBackgroundColor (uint16_t color) |
Set the background color used to render text. | |
void | drawString (const uint8_t *pFont, int x, int y, const char *pString) |
Draw a string to the screen using the currently active foreground and background colors. | |
void | measureString (const uint8_t *pFont, const char *pString, uint8_t &width, uint8_t &height) |
Measure the width and height of the string when rendered with the specified font. | |
void | selectDevice () |
Select the device on the SPI bus. |
Detailed Description
LCD_ST7735 is a simple driver for the ST7735 LCD controller.
It provides basic drawing primitives sa well as text and font capabilities. The driver is currently hardcoded to support 65K colors using a 565 RGB pixel format.
Definition at line 16 of file LCD_ST7735.h.
Member Enumeration Documentation
enum Orientation |
Orientation of the display.
- Enumerator:
Rotate0 No rotation of the display image.
Rotate90 Rotate the display image 90 degrees.
Rotate180 Rotate the display image 180 degrees.
Rotate270 Rotate the display image 270 degrees.
Definition at line 20 of file LCD_ST7735.h.
enum PanelColorFilter |
Type of color filter of the panel.
Definition at line 33 of file LCD_ST7735.h.
Constructor & Destructor Documentation
LCD_ST7735 | ( | PinName | backlightPin, |
PinName | resetPin, | ||
PinName | dsPin, | ||
PinName | mosiPin, | ||
PinName | misoPin, | ||
PinName | clkPin, | ||
PinName | csPin, | ||
PanelColorFilter | colorFilter = BGR |
||
) |
Creates an instance of the LCD_ST7735 driver.
- Parameters:
-
backlightPin pin used to control the backlight resetPin pin used to reset the display controller dsPin pin used to put the display controller into data mode mosiPin SPI channel MOSI pin misoPin SPI channel MISO pin clkPin SPI channel clock pin csPin SPI chip select pin
Definition at line 4 of file LCD_ST7735.cpp.
Member Function Documentation
void clearScreen | ( | uint16_t | color = 0x0000 ) |
Clear the screen.
- Parameters:
-
color The color used to clear the screen. Defaults to black if not passed.
Definition at line 82 of file LCD_ST7735.cpp.
void drawBitmap | ( | int | x, |
int | y, | ||
const uint16_t * | pbmp, | ||
int | srcX, | ||
int | srcY, | ||
int | srcWidth, | ||
int | srcHeight | ||
) |
Extracts a portion of a bitmap and draws it on the screen.
- Parameters:
-
x The X coordinate location to draw the bitmap. y The Y coordinate location to draw the bitmap. pbmp Pointer to the bitmap. srcX X offset into the source bitmap of the portion to extract srcY Y offset into the source bitmap of the portion to extract srcWidth Width of the bitmap portion to draw srcHeight Height of the bitmap portion to draw
- Note:
- The bitmap is an single dimensional uint8_t (unsigned short) array. The first to elements of the array indicate the width and height of the bitmap repectively. The rest of the entries int the array make up the pixel data for the array.
Definition at line 357 of file LCD_ST7735.cpp.
void drawBitmap | ( | int | x, |
int | y, | ||
const uint16_t * | pbmp | ||
) |
Draw a bitmap on the screen.
- Parameters:
-
x The X coordinate location to draw the bitmap. y The Y coordinate location to draw the bitmap. pbmp Pointer to the bitmap.
- Note:
- The bitmap is an single dimensional uint8_t (unsigned short) array. The first to elements of the array indicate the width and height of the bitmap repectively. The rest of the entries int the array make up the pixel data for the array.
Definition at line 342 of file LCD_ST7735.cpp.
void drawCircle | ( | int | x, |
int | y, | ||
int | r, | ||
uint16_t | color | ||
) |
Draw a circle on the display.
- Parameters:
-
x The X coordinate of the center of the circle y The Y coordinate of the center of the circle r The radius of the circle color The color used to draw the circle
Definition at line 157 of file LCD_ST7735.cpp.
void drawEllipse | ( | int | x, |
int | y, | ||
int | rx, | ||
int | ry, | ||
uint16_t | color | ||
) |
Draw an ellipse on the display.
- Parameters:
-
x The X coordinate of the center of the ellipse y The Y coordinate of the center of the ellipse rx The X radius of the ellipse ry The X radius of the ellipse color The color used to draw the ellipse
Definition at line 186 of file LCD_ST7735.cpp.
void drawLine | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint16_t | color | ||
) |
Draw a line on the display.
- Parameters:
-
x1 The X coordinate of the starting point on the line y1 The Y coordinate of the starting point on the line x2 The X coordinate of the end point on the line y2 The Y coordinate of the end point on the line color The color used to draw the pixel
Definition at line 102 of file LCD_ST7735.cpp.
void drawRect | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint16_t | color | ||
) |
Draw a rectangle on the display.
- Parameters:
-
x1 The X coordinate of the upper left corner y1 The Y coordinate of the upper left corner x2 The X coordinate of the lower right corner y2 The Y coordinate of the lower right corner color The color used to draw the rectangle
Definition at line 146 of file LCD_ST7735.cpp.
void drawString | ( | const uint8_t * | pFont, |
int | x, | ||
int | y, | ||
const char * | pString | ||
) |
Draw a string to the screen using the currently active foreground and background colors.
- Parameters:
-
pFont Pointer to the font used to render the string to the display x The X coordinate location to draw the string. y The Y coordinate location to draw the string. pString ASCIIZ string to draw to the display.
Definition at line 388 of file LCD_ST7735.cpp.
void fillCircle | ( | int | x, |
int | y, | ||
int | r, | ||
uint16_t | borderColor, | ||
uint16_t | fillColor | ||
) |
Draw a filled circle on the display.
- Parameters:
-
x The X coordinate of the center of the circle y The Y coordinate of the center of the circle borderColor The color used to draw the circumference of the circle fillColor The color used to fill the circle
Definition at line 262 of file LCD_ST7735.cpp.
void fillEllipse | ( | int | x, |
int | y, | ||
int | rx, | ||
int | ry, | ||
uint16_t | borderColor, | ||
uint16_t | fillColor | ||
) |
Draw a filled ellipse on the display.
- Parameters:
-
x The X coordinate of the center of the ellipse y The Y coordinate of the center of the ellipse rx The X radius of the ellipse ry The X radius of the ellipse borderColor The color used to draw the circumference of the circle fillColor The color used to fill the circle
Definition at line 298 of file LCD_ST7735.cpp.
void fillRect | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint16_t | fillColor | ||
) |
Draw a filled rectangle on the display.
- Parameters:
-
x1 The X coordinate of the upper left corner y1 The Y coordinate of the upper left corner x2 The X coordinate of the lower right corner y2 The Y coordinate of the lower right corner fillColor The color used to fill the rectangle
Definition at line 222 of file LCD_ST7735.cpp.
void fillRect | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
uint16_t | borderColor, | ||
uint16_t | fillColor | ||
) |
Draw a filled rectangle on the display.
- Parameters:
-
x1 The X coordinate of the upper left corner y1 The Y coordinate of the upper left corner x2 The X coordinate of the lower right corner y2 The Y coordinate of the lower right corner borderColor The color used to draw the rectangle frame fillColor The color used to fill the rectangle
Definition at line 239 of file LCD_ST7735.cpp.
int getHeight | ( | ) |
Get the height of the display given the current orientation.
Definition at line 72 of file LCD_ST7735.cpp.
int getWidth | ( | ) |
Get the width of the display given the current orientation.
Definition at line 67 of file LCD_ST7735.cpp.
void measureString | ( | const uint8_t * | pFont, |
const char * | pString, | ||
uint8_t & | width, | ||
uint8_t & | height | ||
) |
Measure the width and height of the string when rendered with the specified font.
- Parameters:
-
pFont Pointer to the font used to measure the string pString ASCIIZ string to measure. width Reference to the variable that will contain the width height Reference to the variable that will contain the height
Definition at line 411 of file LCD_ST7735.cpp.
void selectDevice | ( | ) |
Select the device on the SPI bus.
selectDevice needs to be called before accessing the screen if there are multiple devices on the SPI bus.
Definition at line 424 of file LCD_ST7735.cpp.
void setBackgroundColor | ( | uint16_t | color ) |
Set the background color used to render text.
- Parameters:
-
color Color used when drawing background portions of the text
- Note:
- The color can be changed multiple times to render text with various background colors on the display
Definition at line 382 of file LCD_ST7735.cpp.
void setBacklight | ( | bool | state ) |
Control the display's backlight.
- Parameters:
-
state true to turn the backlight on, false to turn it off
Definition at line 77 of file LCD_ST7735.cpp.
void setForegroundColor | ( | uint16_t | color ) |
Set the foreground color used to render text.
- Parameters:
-
color Color used when drawing text to the display
- Note:
- The color can be changed multiple times to render text in various colors on the display
Definition at line 376 of file LCD_ST7735.cpp.
void setOrientation | ( | Orientation | orientation, |
bool | flip | ||
) |
Set the orientation of the display.
- Parameters:
-
orientation Orientation of the display. flip Flips the display direction
Definition at line 31 of file LCD_ST7735.cpp.
void setPixel | ( | int | x, |
int | y, | ||
uint16_t | color | ||
) |
Set a pixel on the display to the specified color.
- Parameters:
-
x The X coordinate of the pixel (0..127) y The Y coordinate of the pixel (0..159) color Color to set the pixel to.
Definition at line 95 of file LCD_ST7735.cpp.
Generated on Thu Jul 14 2022 20:06:44 by
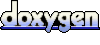