
Retro Invaders a space invaders clone by Chris Favreau. Written for the RetroMbuino development board from outrageouscircuits.com for the game programming contest.
LCD_ST7735.cpp
00001 #include "mbed.h" 00002 #include "LCD_ST7735.h" 00003 00004 LCD_ST7735::LCD_ST7735( 00005 PinName backlightPin, 00006 PinName resetPin, 00007 PinName dsPin, 00008 PinName mosiPin, 00009 PinName misoPin, 00010 PinName clkPin, 00011 PinName csPin, 00012 PanelColorFilter colorFilter 00013 ) : 00014 _colorFilter(colorFilter), 00015 _backlight(backlightPin, 0), 00016 _reset(resetPin, 1), 00017 _ds(dsPin, 0), 00018 _cs(csPin, 1), 00019 _spi(mosiPin, misoPin, clkPin) 00020 { 00021 _spi.format(8, 3); 00022 _spi.frequency(15000000); 00023 00024 initDisplay(); 00025 setOrientation(Rotate270, false); 00026 clearScreen(); 00027 setForegroundColor(0xffff); 00028 setBackgroundColor(0x0000); 00029 } 00030 00031 void LCD_ST7735::setOrientation(Orientation orientation, bool flip) 00032 { 00033 const static uint8_t my = 0x80; 00034 const static uint8_t mx = 0x40; 00035 const static uint8_t mv = 0x20; 00036 00037 uint8_t madctlData = _colorFilter; 00038 switch(orientation) 00039 { 00040 case Rotate0: 00041 _width = 128; 00042 _height = 160; 00043 madctlData |= flip ? mx : 0; 00044 break; 00045 00046 case Rotate90: 00047 _width = 160; 00048 _height = 128; 00049 madctlData |= flip ? my | mv | mx : mv | mx; 00050 break; 00051 00052 case Rotate180: 00053 _width = 128; 00054 _height = 160; 00055 madctlData |= flip ? my : mx | my; 00056 break; 00057 00058 case Rotate270: 00059 _width = 160; 00060 _height = 128; 00061 madctlData |= flip ? mv : mv | my; 00062 break; 00063 } 00064 write(CMD_MADCTL, (uint8_t[]){madctlData}, 1); 00065 } 00066 00067 int LCD_ST7735::getWidth() 00068 { 00069 return _width; 00070 } 00071 00072 int LCD_ST7735::getHeight() 00073 { 00074 return _height; 00075 } 00076 00077 void LCD_ST7735::setBacklight(bool state) 00078 { 00079 _backlight = state ? 1 : 0; 00080 } 00081 00082 void LCD_ST7735::clearScreen(uint16_t color) 00083 { 00084 clipRect(0, 0, _width - 1, _height - 1); 00085 beginBatchCommand(CMD_RAMWR); 00086 uint8_t colorHigh = color >> 8; 00087 uint8_t colorLow = color; 00088 for(int i = 0; i < 128 * 160 * 2; ++i) 00089 { 00090 writeBatchData(colorHigh, colorLow); 00091 } 00092 endBatchCommand(); 00093 } 00094 00095 void LCD_ST7735::setPixel(int x, int y, uint16_t color) 00096 { 00097 write(CMD_CASET, (uint8_t[]){0, x, 0, x}, 4); 00098 write(CMD_RASET, (uint8_t[]){0, y, 0, y}, 4); 00099 write(CMD_RAMWR, color); 00100 } 00101 00102 void LCD_ST7735::drawLine(int x1, int y1, int x2, int y2, uint16_t color) 00103 { 00104 int dx = abs(x2 - x1); 00105 int dy = abs(y2 - y1); 00106 00107 if (dx == 0) 00108 { 00109 drawVertLine(x1, y1, y2, color); 00110 return; 00111 } 00112 else if(dy == 0) 00113 { 00114 drawHorizLine(x1, y1, x2, color); 00115 return; 00116 } 00117 00118 int sx = (x1 < x2) ? 1 : -1; 00119 int sy = (y1 < y2) ? 1 : -1; 00120 int err = dx - dy; 00121 while(x1 != x2 || y1 != y2) 00122 { 00123 setPixel(x1, y1, color); 00124 int e2 = err << 1; 00125 if (e2 > -dy) 00126 { 00127 err -= dy; 00128 x1 += sx; 00129 } 00130 if (e2 < dx) 00131 { 00132 err += dx; 00133 y1 += sy; 00134 } 00135 } 00136 setPixel(x2, y2, color); 00137 } 00138 00139 void LCD_ST7735::swap(int &a, int &b) 00140 { 00141 int t = a; 00142 a = b; 00143 b = t; 00144 } 00145 00146 void LCD_ST7735::drawRect(int x1, int y1, int x2, int y2, uint16_t color) 00147 { 00148 if (x1 > x2) swap(x1, x2); 00149 if (y1 > y2) swap(y1, y2); 00150 00151 drawHorizLine(x1, y1, x2, color); 00152 drawHorizLine(x1, y2, x2, color); 00153 drawVertLine(x1, y1, y2, color); 00154 drawVertLine(x2, y1, y2, color); 00155 } 00156 00157 void LCD_ST7735::drawCircle(int x, int y, int r, uint16_t color) 00158 { 00159 int ix = r; 00160 int iy = 0; 00161 int err = 1 - r; 00162 00163 while(ix >= iy) 00164 { 00165 setPixel(x + ix, y + iy, color); 00166 setPixel(x + iy, y + ix, color); 00167 setPixel(x - ix, y + iy, color); 00168 setPixel(x - iy, y + ix, color); 00169 setPixel(x - ix, y - iy, color); 00170 setPixel(x - iy, y - ix, color); 00171 setPixel(x + ix, y - iy, color); 00172 setPixel(x + iy, y - ix, color); 00173 iy++; 00174 if (err < 0) 00175 { 00176 err += 2 * iy + 1; 00177 } 00178 else 00179 { 00180 ix--; 00181 err += 2 * (iy - ix + 1); 00182 } 00183 } 00184 } 00185 00186 void LCD_ST7735::drawEllipse(int x, int y, int rx, int ry, uint16_t color) 00187 { 00188 int a2 = rx * rx; 00189 int b2 = ry * ry; 00190 int fa2 = 4 * a2; 00191 int fb2 = 4 * b2; 00192 00193 int ix, iy, sigma; 00194 for (ix = 0, iy = ry, sigma = 2 * b2 + a2 * (1 - 2 * ry); b2 * ix <= a2 * iy; ix++) 00195 { 00196 setPixel(x + ix, y + iy, color); 00197 setPixel(x - ix, y + iy, color); 00198 setPixel(x + ix, y - iy, color); 00199 setPixel(x - ix, y - iy, color); 00200 if (sigma >= 0) 00201 { 00202 sigma+= fa2 * (1 - iy); 00203 iy--; 00204 } 00205 sigma += b2 * ((4 * ix) + 6); 00206 } 00207 00208 for (ix = rx, iy = 0, sigma = 2 * a2 + b2 * (1 - 2 * rx); a2 * iy <= b2 * ix; iy++) 00209 { 00210 setPixel(x + ix, y + iy, color); 00211 setPixel(x - ix, y + iy, color); 00212 setPixel(x + ix, y - iy, color); 00213 setPixel(x - ix, y - iy, color); 00214 if (sigma >= 0) 00215 { 00216 sigma+= fb2 * (1 - ix); 00217 ix--; 00218 } 00219 sigma += a2 * ((4 * iy) + 6); 00220 } 00221 } 00222 void LCD_ST7735::fillRect(int x1, int y1, int x2, int y2, uint16_t fillColor) 00223 { 00224 if (x1 > x2) swap(x1, x2); 00225 if (y1 > y2) swap(y1, y2); 00226 00227 clipRect(x1, y1, x2, y2); 00228 int c = ((x2-x1) * (y2-y1)) << 1; 00229 uint8_t colorHigh = fillColor >> 8; 00230 uint8_t colorLow = fillColor; 00231 beginBatchCommand(CMD_RAMWR); 00232 while(c--) 00233 { 00234 writeBatchData(colorHigh, colorLow); 00235 } 00236 endBatchCommand(); 00237 } 00238 00239 void LCD_ST7735::fillRect(int x1, int y1, int x2, int y2, uint16_t borderColor, uint16_t fillColor) 00240 { 00241 if (x1 > x2) swap(x1, x2); 00242 if (y1 > y2) swap(y1, y2); 00243 00244 drawRect(x1, y1, x2, y2, borderColor); 00245 ++x1; ++y1; --x2; --y2; 00246 if (x2 >= x1 && y2 >= y1) 00247 { 00248 int c = ((x2 + 1 - x1) * (y2 + 1 - y1)) << 1; 00249 00250 clipRect(x1, y1, x2, y2); 00251 uint8_t colorHigh = fillColor >> 8; 00252 uint8_t colorLow = fillColor; 00253 beginBatchCommand(CMD_RAMWR); 00254 while(c--) 00255 { 00256 writeBatchData(colorHigh, colorLow); 00257 } 00258 endBatchCommand(); 00259 } 00260 } 00261 00262 void LCD_ST7735::fillCircle(int x, int y, int r, uint16_t borderColor, uint16_t fillColor) 00263 { 00264 int ix = r; 00265 int iy = 0; 00266 int err = 1 - r; 00267 00268 while(ix >= iy) 00269 { 00270 setPixel(x - ix, y + iy, borderColor); 00271 setPixel(x + ix, y + iy, borderColor); 00272 drawHorizLine(x - ix + 1, y + iy, x + ix - 1, fillColor); 00273 00274 setPixel(x - iy, y + ix, borderColor); 00275 setPixel(x + iy, y + ix, borderColor); 00276 drawHorizLine(x - iy + 1, y + ix, x + iy - 1, fillColor); 00277 00278 setPixel(x - ix, y - iy, borderColor); 00279 setPixel(x + ix, y - iy, borderColor); 00280 drawHorizLine(x - ix + 1, y - iy, x + ix - 1, fillColor); 00281 00282 setPixel(x - iy, y - ix, borderColor); 00283 setPixel(x + iy, y - ix, borderColor); 00284 drawHorizLine(x - iy + 1, y - ix, x + iy - 1, fillColor); 00285 iy++; 00286 if (err < 0) 00287 { 00288 err += 2 * iy + 1; 00289 } 00290 else 00291 { 00292 ix--; 00293 err += 2 * (iy - ix + 1); 00294 } 00295 } 00296 } 00297 00298 void LCD_ST7735::fillEllipse(int x, int y, int rx, int ry, uint16_t borderColor, uint16_t fillColor) 00299 { 00300 int a2 = rx * rx; 00301 int b2 = ry * ry; 00302 int fa2 = 4 * a2; 00303 int fb2 = 4 * b2; 00304 00305 int ix, iy, sigma; 00306 for (ix = 0, iy = ry, sigma = 2 * b2 + a2 * (1 - 2 * ry); b2 * ix <= a2 * iy; ix++) 00307 { 00308 setPixel(x + ix, y + iy, borderColor); 00309 setPixel(x - ix, y + iy, borderColor); 00310 drawHorizLine(x - ix + 1, y + iy, x + ix - 1, fillColor); 00311 00312 setPixel(x + ix, y - iy, borderColor); 00313 setPixel(x - ix, y - iy, borderColor); 00314 drawHorizLine(x - ix + 1, y - iy, x + ix - 1, fillColor); 00315 00316 if (sigma >= 0) 00317 { 00318 sigma+= fa2 * (1 - iy); 00319 iy--; 00320 } 00321 sigma += b2 * ((4 * ix) + 6); 00322 } 00323 00324 for (ix = rx, iy = 0, sigma = 2 * a2 + b2 * (1 - 2 * rx); a2 * iy <= b2 * ix; iy++) 00325 { 00326 setPixel(x + ix, y + iy, borderColor); 00327 setPixel(x - ix, y + iy, borderColor); 00328 drawHorizLine(x - ix + 1, y + iy, x + ix - 1, fillColor); 00329 00330 setPixel(x + ix, y - iy, borderColor); 00331 setPixel(x - ix, y - iy, borderColor); 00332 drawHorizLine(x - ix + 1, y - iy, x + ix - 1, fillColor); 00333 if (sigma >= 0) 00334 { 00335 sigma+= fb2 * (1 - ix); 00336 ix--; 00337 } 00338 sigma += a2 * ((4 * iy) + 6); 00339 } 00340 } 00341 00342 void LCD_ST7735::drawBitmap(int x, int y, const uint16_t *pbmp) 00343 { 00344 int w = *pbmp++; 00345 int h = *pbmp++; 00346 00347 clip(x, y, w, h); 00348 int c = w * h; 00349 beginBatchCommand(CMD_RAMWR); 00350 while(c--) 00351 { 00352 writeBatchData(*pbmp++); 00353 } 00354 endBatchCommand(); 00355 } 00356 00357 void LCD_ST7735::drawBitmap(int x, int y, const uint16_t *pbmp, int srcX, int srcY, int srcWidth, int srcHeight) 00358 { 00359 int w = *pbmp++; 00360 int h = *pbmp++; 00361 00362 clip(x, y, srcWidth, srcHeight); 00363 beginBatchCommand(CMD_RAMWR); 00364 const uint16_t *p = pbmp + srcX + (srcY * w); 00365 for(int iy = 0; iy < srcHeight; ++iy) 00366 { 00367 for(int ix = 0; ix < srcWidth; ++ix) 00368 { 00369 writeBatchData(*(p + ix)); 00370 } 00371 p += w; 00372 } 00373 endBatchCommand(); 00374 } 00375 00376 void LCD_ST7735::setForegroundColor(uint16_t color) 00377 { 00378 _foregroundColorHigh = color >> 8; 00379 _foregroundColorLow = color; 00380 } 00381 00382 void LCD_ST7735::setBackgroundColor(uint16_t color) 00383 { 00384 _backgroundColorHigh = color >> 8; 00385 _backgroundColorLow = color; 00386 } 00387 00388 void LCD_ST7735::drawString(const uint8_t *pFont, int x, int y, const char *pString) 00389 { 00390 uint8_t w = *pFont; 00391 uint8_t h = *(pFont + 1); 00392 uint8_t offset = *(pFont + 2); 00393 uint8_t leftPad = *(pFont + 3); 00394 uint8_t rightPad = *(pFont + 4); 00395 uint8_t topPad = *(pFont + 5); 00396 uint8_t bottomPad = *(pFont + 6); 00397 00398 if (y + topPad + h + bottomPad < 0) return; 00399 if (y >= _height) return; 00400 if (x + leftPad + w + rightPad < 0) return; 00401 00402 char *p = (char*)pString; 00403 while(*p != 0) 00404 { 00405 if (x >= _width) return; 00406 drawChar(pFont, x, y, *p++, w, h, offset, leftPad, rightPad, topPad, bottomPad); 00407 x += (w + leftPad + rightPad); 00408 } 00409 } 00410 00411 void LCD_ST7735::measureString(const uint8_t *pFont, const char *pString, uint8_t &width, uint8_t &height) 00412 { 00413 uint8_t w = *pFont; 00414 uint8_t h = *(pFont + 1); 00415 uint8_t leftPad = *(pFont + 3); 00416 uint8_t rightPad = *(pFont + 4); 00417 uint8_t topPad = *(pFont + 5); 00418 uint8_t bottomPad = *(pFont + 6); 00419 00420 width = (w + leftPad + rightPad) * strlen(pString); 00421 height = (h + topPad + bottomPad); 00422 } 00423 00424 void LCD_ST7735::selectDevice() 00425 { 00426 _spi.prepareFastSPI(); 00427 } 00428 00429 void LCD_ST7735::drawVertLine(int x1, int y1, int y2, uint16_t color) 00430 { 00431 clipRect(x1, y1, x1, y2); 00432 beginBatchCommand(CMD_RAMWR); 00433 int c = (y2 - y1) << 1; 00434 uint8_t colorHigh = color >> 8; 00435 uint8_t colorLow = color; 00436 for (int i = 0; i < c; ++i) 00437 { 00438 writeBatchData(colorHigh, colorLow); 00439 } 00440 endBatchCommand(); 00441 } 00442 00443 void LCD_ST7735::drawHorizLine(int x1, int y1, int x2, uint16_t color) 00444 { 00445 clipRect(x1, y1, x2, y1); 00446 beginBatchCommand(CMD_RAMWR); 00447 int c = (x2 - x1) << 1; 00448 uint8_t colorHigh = color >> 8; 00449 uint8_t colorLow = color; 00450 for (int i = 0; i < c; ++i) 00451 { 00452 writeBatchData(colorHigh, colorLow); 00453 } 00454 endBatchCommand(); 00455 } 00456 00457 void LCD_ST7735::drawChar(const uint8_t *pFont, int x, int y, char c, uint8_t w, uint8_t h, uint8_t offset, uint8_t leftPad, uint8_t rightPad, uint8_t topPad, uint8_t bottomPad) 00458 { 00459 const uint8_t *pChar = (pFont + 7) + ((c - offset) * h); 00460 00461 clip(x, y, w + leftPad + rightPad, h + topPad + bottomPad); 00462 00463 beginBatchCommand(CMD_RAMWR); 00464 00465 // Render top spacing 00466 for (int r = 0; r < topPad; ++r) 00467 { 00468 for (int c = 0; c < w + leftPad + rightPad; ++c) 00469 { 00470 writeBatchData(_backgroundColorHigh); 00471 writeBatchData(_backgroundColorLow); 00472 } 00473 } 00474 00475 // Render character 00476 for(int r = 0; r < h; ++r) 00477 { 00478 uint8_t b = pChar[r]; 00479 00480 // Render left spacing 00481 for (int c = 0; c < leftPad; ++c) 00482 { 00483 writeBatchData(_backgroundColorHigh); 00484 writeBatchData(_backgroundColorLow); 00485 } 00486 for(int c = 0; c < w; ++c) 00487 { 00488 if (b & 0x80) 00489 { 00490 writeBatchData(_foregroundColorHigh); 00491 writeBatchData(_foregroundColorLow); 00492 } 00493 else 00494 { 00495 writeBatchData(_backgroundColorHigh); 00496 writeBatchData(_backgroundColorLow); 00497 } 00498 00499 b <<= 1; 00500 } 00501 00502 for (int c = 0; c < rightPad; ++c) 00503 { 00504 writeBatchData(_backgroundColorHigh); 00505 writeBatchData(_backgroundColorLow); 00506 } 00507 } 00508 00509 // Render bottom spacing 00510 for (int r = 0; r < bottomPad; ++r) 00511 { 00512 for (int c = 0; c < w + leftPad + rightPad; ++c) 00513 { 00514 writeBatchData(_backgroundColorHigh); 00515 writeBatchData(_backgroundColorLow); 00516 } 00517 } 00518 endBatchCommand(); 00519 } 00520 00521 void LCD_ST7735::initDisplay() 00522 { 00523 selectDevice(); 00524 reset(); 00525 00526 writeCommand(CMD_SLPOUT); 00527 00528 write(CMD_FRMCTR1, (uint8_t[]){0x01, 0x2c, 0x2d}, 3); 00529 write(CMD_FRMCTR2, (uint8_t[]){0x01, 0x2c, 0x2d}, 3); 00530 write(CMD_FRMCTR3, (uint8_t[]){0x01, 0x2c, 0x2d, 0x01, 0x2c, 0x2d}, 6); 00531 00532 write(CMD_INVCTR, (uint8_t[]){0x07}, 1); 00533 00534 write(CMD_PWCTR1, (uint8_t[]){0xa2, 0x02, 0x84}, 3); 00535 write(CMD_PWCTR2, (uint8_t[]){0xc5}, 1); 00536 write(CMD_PWCTR3, (uint8_t[]){0x0a, 0x00}, 2); 00537 write(CMD_PWCTR4, (uint8_t[]){0x8a, 0x2a}, 2); 00538 write(CMD_PWCTR5, (uint8_t[]){0x8a, 0xee}, 2); 00539 00540 write(CMD_VMCTR1, (uint8_t[]){0x0e}, 1); 00541 00542 write(CMD_MADCTL, (uint8_t[]){0xc0 | _colorFilter}, 1); 00543 00544 // Gama sequence 00545 write(CMD_GAMCTRP1, (uint8_t[]) 00546 { 00547 0x0f, 0x1a, 00548 0x0f, 0x18, 00549 0x2f, 0x28, 00550 0x20, 0x22, 00551 0x1f, 0x1b, 00552 0x23, 0x37, 00553 0x00, 0x07, 00554 0x02, 0x10 00555 }, 16); 00556 00557 write(CMD_GAMCTRN1, (uint8_t[]) 00558 { 00559 0x0f, 0x1b, 00560 0x0f, 0x17, 00561 0x33, 0x2c, 00562 0x29, 0x2e, 00563 0x30, 0x30, 00564 0x39, 0x3f, 00565 0x00, 0x07, 00566 0x03, 0x10 00567 }, 16); 00568 00569 write(CMD_CASET, (uint8_t[]){0x00, 0x00, 0x00, 0x7f}, 4); 00570 write(CMD_RASET, (uint8_t[]){0x00, 0x00, 0x00, 0x9f}, 4); 00571 00572 write(CMD_EXTCTRL, (uint8_t[]){0x01}, 1); 00573 00574 // Disable RAM power save 00575 write(0xf6, (uint8_t[]){0x00}, 1); 00576 00577 // 65k color mode 00578 write(CMD_COLMOD, (uint8_t[]){0x05}, 1); 00579 00580 // Enable display 00581 writeCommand(CMD_DISPON); 00582 00583 setBacklight(true); 00584 } 00585 00586 void LCD_ST7735::reset() 00587 { 00588 _reset = 0; 00589 wait_us(100); 00590 _reset = 1; 00591 wait_us(100); 00592 } 00593 00594 void LCD_ST7735::clip(int x, int y, int w, int h) 00595 { 00596 clipRect(x, y, (x + w) - 1, (y + h) - 1); 00597 } 00598 00599 void LCD_ST7735::clipRect(int x1, int y1, int x2, int y2) 00600 { 00601 uint8_t x1l = (uint8_t)x1; 00602 uint8_t x1h = (uint8_t)(x1 >> 8); 00603 uint8_t x2l = (uint8_t)x2; 00604 uint8_t x2h = (uint8_t)(x2 >> 8); 00605 write(CMD_CASET, (uint8_t[]){x1h, x1l, x2h, x2l}, 4); 00606 00607 uint8_t y1l = (uint8_t)y1; 00608 uint8_t y1h = (uint8_t)(y1 >> 8); 00609 uint8_t y2l = (uint8_t)y2; 00610 uint8_t y2h = (uint8_t)(y2 >> 8); 00611 write(CMD_RASET, (uint8_t[]){y1h, y1l, y2h, y2l}, 4); 00612 } 00613 00614 void LCD_ST7735::writeCommand(uint8_t cmd) 00615 { 00616 _cs = 0; 00617 _ds = 0; 00618 _spi.fastWrite(cmd); 00619 _spi.waitWhileBusy(); 00620 _spi.clearRx(); 00621 _cs = 1; 00622 } 00623 00624 void LCD_ST7735::write(uint8_t cmd, uint8_t data[], int dataLen) 00625 { 00626 _cs = 0; 00627 _ds = 0; 00628 _spi.fastWrite(cmd); 00629 _spi.waitWhileBusy(); 00630 if (data != NULL & dataLen > 0) 00631 { 00632 _ds = 1; 00633 for(int i = 0; i < dataLen; ++i) 00634 { 00635 _spi.fastWrite(data[i]); 00636 } 00637 _spi.waitWhileBusy(); 00638 _ds = 0; 00639 } 00640 _spi.clearRx(); 00641 _cs = 1; 00642 } 00643 00644 void LCD_ST7735::write(uint8_t cmd, uint16_t data) 00645 { 00646 _cs = 0; 00647 _ds = 0; 00648 _spi.fastWrite(cmd); 00649 _spi.waitWhileBusy(); 00650 _ds = 1; 00651 _spi.fastWrite(data >> 8); 00652 _spi.fastWrite(data); 00653 _spi.waitWhileBusy(); 00654 _spi.clearRx(); 00655 _ds = 0; 00656 _cs = 1; 00657 } 00658 00659 void LCD_ST7735::beginBatchCommand(uint8_t cmd) 00660 { 00661 _cs = 0; 00662 _ds = 0; 00663 _spi.fastWrite(cmd); 00664 _spi.waitWhileBusy(); 00665 _ds = 1; 00666 } 00667 00668 void LCD_ST7735::writeBatchData(uint8_t data) 00669 { 00670 _spi.fastWrite(data); 00671 } 00672 00673 void LCD_ST7735::writeBatchData(uint8_t dataHigh, uint8_t dataLow) 00674 { 00675 _spi.fastWrite(dataHigh); 00676 _spi.fastWrite(dataLow); 00677 } 00678 00679 00680 void LCD_ST7735::writeBatchData(uint16_t data) 00681 { 00682 _spi.fastWrite(data >> 8); 00683 _spi.fastWrite(data); 00684 } 00685 00686 void LCD_ST7735::endBatchCommand() 00687 { 00688 _spi.waitWhileBusy(); 00689 _spi.clearRx(); 00690 _ds = 0; 00691 _cs = 1; 00692 }
Generated on Thu Jul 14 2022 20:06:44 by
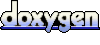