
Test program for TM1640 LED controller library. Initial version
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed TM1640 Test program, for TM1640 LED controller 00002 * Copyright (c) 2016, v01: WH, Initial version 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 #include "mbed.h" 00023 #include "TM1640.h" 00024 00025 #if (LM1640_TEST == 1) 00026 // LM1640 TM1640 Test 00027 #include "Font_7Seg.h" 00028 00029 Serial pc(USBTX, USBRX); 00030 DigitalOut myled(LED1); 00031 00032 // DisplayData_t size is 16 bytes (16 Grids @ 8 Segments) 00033 TM1640::DisplayData_t all_str = {0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF}; 00034 TM1640::DisplayData_t cls_str = {0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00}; 00035 TM1640::DisplayData_t hello_str = {C7_H,C7_E, C7_L,C7_L, C7_O,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00}; 00036 TM1640::DisplayData_t bye_str = {C7_B,C7_Y, C7_E,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00}; 00037 00038 //TM1640_LM1640 declaration 00039 TM1640_LM1640 LM1640(p5,p7); 00040 00041 00042 void show_menu() { 00043 // pc.printf("0: Exit\n\r"); 00044 pc.printf("1: All\n\r"); 00045 pc.printf("2: Show all segs\r\n"); 00046 pc.printf("3: Show all chars\n\r"); 00047 pc.printf("4: Show all digits\n\r"); 00048 pc.printf("5: Show all icons\n\r"); 00049 pc.printf("6: Counter\n\r"); 00050 pc.printf("7: Bye\n\r"); 00051 pc.printf("8: Floats\n\r"); 00052 } 00053 00054 00055 char cmd, bits; 00056 int main() { 00057 00058 pc.printf("Hello World\r\n"); // 00059 00060 LM1640.cls(); 00061 LM1640.writeData(all_str); 00062 wait(2); 00063 LM1640.setBrightness(TM1640_BRT3); 00064 wait(1); 00065 LM1640.setBrightness(TM1640_BRT0); 00066 wait(1); 00067 LM1640.setBrightness(TM1640_BRT4); 00068 00069 wait(1); 00070 LM1640.cls(true); 00071 LM1640.writeData(hello_str); 00072 00073 char cmd2 = '0'; 00074 while (1) { 00075 00076 show_menu(); 00077 cmd2 = pc.getc(); 00078 00079 switch (cmd2) { 00080 case '1' : { 00081 pc.printf("all\r\n"); 00082 LM1640.cls(); 00083 LM1640.writeData(all_str); 00084 break; 00085 } 00086 00087 case '2' : { 00088 #if(1) 00089 //test to show all segs 00090 pc.printf("Show all segs\r\n"); 00091 wait(1); 00092 LM1640.cls(); 00093 00094 for (int i=0; i<TM1640_DISPLAY_MEM; i++) { 00095 for (int bit=0; bit<8; bit++) { 00096 LM1640.cls(); 00097 00098 bits = 0x01 << bit; 00099 LM1640.writeData(bits, i); 00100 00101 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00102 // wait(0.5); 00103 cmd = pc.getc(); // wait for key 00104 } 00105 } 00106 pc.printf("\r\nShow all segs done\r\n"); 00107 #endif 00108 break; 00109 } 00110 00111 case '3' : { 00112 00113 #if(1) 00114 //test to show all chars 00115 pc.printf("Show all NATO Alpha chars\r\n"); 00116 00117 // 01234567 00118 const char NATO[][9] = { {"Alpha "}, 00119 {"Bravo "}, 00120 {"Charlie "}, 00121 {"Delta "}, 00122 {"Echo "}, 00123 {"Foxtrot "}, 00124 {"Golf "}, 00125 {"Hotel "}, 00126 {"India "}, 00127 {"Juliet "}, 00128 {"Kilo "}, 00129 {"Lima "}, 00130 {"Mike "}, 00131 {"November"}, 00132 {"Oscar "}, 00133 {"Papa "}, 00134 {"Quebec "}, 00135 {"Romeo "}, 00136 {"Sierra "}, 00137 {"Tango "}, 00138 {"Uniform "}, 00139 {"Victor "}, 00140 {"Whiskey "}, 00141 {"X-ray "}, 00142 {"Yankee "}, 00143 {"Zulu "} 00144 }; 00145 00146 LM1640.cls(); // clear all, preserve Icons 00147 for (int i=0; i<26; i++) { 00148 LM1640.locate(0); 00149 LM1640.printf("%s", NATO[i]); 00150 wait(0.5); 00151 } 00152 pc.printf("Show all NATO Alpha chars done\r\n"); 00153 #endif 00154 00155 #if(0) 00156 //test to show all chars 00157 pc.printf("Show all alpha chars\r\n"); 00158 wait(1); 00159 LM1640.cls(); 00160 00161 for (int i=0; i<26; i++) { 00162 LM1640.printf("%c", char(i + 'A')); 00163 // LM1640.printf("%c", char(i + 'a')); 00164 wait(0.25); 00165 } 00166 pc.printf("Show all alpha chars done\r\n"); 00167 #endif 00168 00169 #if(0) 00170 //test to show all chars 00171 pc.printf("Show all chars\r\n"); 00172 wait(1); 00173 LM1640.cls(); 00174 00175 for (int i=FONT_7S_START; i<FONT_7S_END; i++) { 00176 LM1640.printf("%c", char(i)); 00177 // wait(0.25); 00178 cmd = pc.getc(); // wait for key 00179 } 00180 pc.printf("Show all chars done\r\n"); 00181 #endif 00182 break; 00183 } 00184 case '4': { 00185 #if(0) 00186 //test to show all digits (base is 10) 00187 pc.printf("Show all digits\r\n"); 00188 wait(1); 00189 LM1640.cls(); 00190 00191 for (int i=0; i<LM1640_NR_DIGITS; i++) { 00192 00193 for (int cnt=0; cnt<10; cnt++) { 00194 LM1640.locate(i); 00195 LM1640.printf("%0d", cnt); 00196 00197 // wait(0.5); 00198 cmd = pc.getc(); // wait for key 00199 } 00200 } 00201 pc.printf("\r\nShow all digits done\r\n"); 00202 #endif 00203 00204 #if(1) 00205 //test to show all digits (base is 0x10) 00206 pc.printf("Show all hex digits\r\n"); 00207 wait(1); 00208 LM1640.cls(); 00209 00210 LM1640.printf("%08x", 0x01234567); 00211 LM1640.printf("%08x", 0x89ABCDEF); 00212 cmd = pc.getc(); // wait for key 00213 LM1640.printf("%016x", 0x0); 00214 00215 for (int i=0; i<LM1640_NR_DIGITS; i++) { 00216 00217 for (int cnt=0; cnt<0x10; cnt++) { 00218 LM1640.locate(i); 00219 LM1640.printf("%0x", cnt); 00220 00221 // wait(0.5); 00222 cmd = pc.getc(); // wait for key 00223 } 00224 } 00225 pc.printf("\r\nShow all hex digits done\r\n"); 00226 #endif 00227 break; 00228 } 00229 case '5': { 00230 #if(1) 00231 //test to show all icons 00232 pc.printf("Show all icons\r\n"); 00233 LM1640.cls(true); // Also clear all Icons 00234 00235 float delay=0.1; 00236 // Icons on 00237 LM1640.setIcon(TM1640_LM1640::DP1); wait(delay); 00238 LM1640.setIcon(TM1640_LM1640::DP2); wait(delay); 00239 LM1640.setIcon(TM1640_LM1640::DP3); wait(delay); 00240 LM1640.setIcon(TM1640_LM1640::DP4); wait(delay); 00241 LM1640.setIcon(TM1640_LM1640::DP5); wait(delay); 00242 LM1640.setIcon(TM1640_LM1640::DP6); wait(delay); 00243 LM1640.setIcon(TM1640_LM1640::DP7); wait(delay); 00244 LM1640.setIcon(TM1640_LM1640::DP8); wait(delay); 00245 LM1640.setIcon(TM1640_LM1640::DP9); wait(delay); 00246 LM1640.setIcon(TM1640_LM1640::DP10); wait(delay); 00247 LM1640.setIcon(TM1640_LM1640::DP11); wait(delay); 00248 LM1640.setIcon(TM1640_LM1640::DP12); wait(delay); 00249 LM1640.setIcon(TM1640_LM1640::DP13); wait(delay); 00250 LM1640.setIcon(TM1640_LM1640::DP14); wait(delay); 00251 LM1640.setIcon(TM1640_LM1640::DP15); wait(delay); 00252 LM1640.setIcon(TM1640_LM1640::DP16); wait(delay); 00253 00254 wait(delay); 00255 00256 // Icons off 00257 LM1640.clrIcon(TM1640_LM1640::DP1); wait(delay); 00258 LM1640.clrIcon(TM1640_LM1640::DP2); wait(delay); 00259 LM1640.clrIcon(TM1640_LM1640::DP3); wait(delay); 00260 LM1640.clrIcon(TM1640_LM1640::DP4); wait(delay); 00261 LM1640.clrIcon(TM1640_LM1640::DP5); wait(delay); 00262 LM1640.clrIcon(TM1640_LM1640::DP6); wait(delay); 00263 LM1640.clrIcon(TM1640_LM1640::DP7); wait(delay); 00264 LM1640.clrIcon(TM1640_LM1640::DP8); wait(delay); 00265 LM1640.clrIcon(TM1640_LM1640::DP9); wait(delay); 00266 LM1640.clrIcon(TM1640_LM1640::DP10); wait(delay); 00267 LM1640.clrIcon(TM1640_LM1640::DP11); wait(delay); 00268 LM1640.clrIcon(TM1640_LM1640::DP12); wait(delay); 00269 LM1640.clrIcon(TM1640_LM1640::DP13); wait(delay); 00270 LM1640.clrIcon(TM1640_LM1640::DP14); wait(delay); 00271 LM1640.clrIcon(TM1640_LM1640::DP15); wait(delay); 00272 LM1640.clrIcon(TM1640_LM1640::DP16); wait(delay); 00273 // wait(1); 00274 // LM1640.cls(); // clear all, preserve Icons 00275 pc.printf("Show all icons done\r\n"); 00276 #endif 00277 break; 00278 } 00279 00280 case '6': { 00281 LM1640.cls(); // clear all, preserve Icons 00282 00283 #if(1) 00284 LM1640.locate(0); 00285 LM1640.printf("Counters "); 00286 00287 for (int cnt=0; cnt<=0xFF; cnt++) { 00288 LM1640.locate(10); 00289 LM1640.printf("%02x", cnt); 00290 LM1640.locate(14); 00291 LM1640.printf("%02x", 0xFF - cnt); 00292 00293 wait(0.2); 00294 } 00295 #endif 00296 00297 // LM1640.writeData(hello_str); 00298 // LM1640.printf("hello"); 00299 00300 break; 00301 } 00302 case '7': { 00303 00304 LM1640.cls(); // clear all, preserve Icons 00305 // LM1640.writeData(bye_str); 00306 LM1640.printf("Bye"); 00307 00308 break; 00309 } 00310 00311 case '8': { 00312 LM1640.cls(); // clear all, preserve Icons 00313 LM1640.printf("%2.3f", -0.1234); // test decimal point display 00314 wait(0.5); 00315 LM1640.cls(); // clear all, preserve Icons 00316 LM1640.printf("%2.3f", -012.345); // test decimal point display 00317 break; 00318 } 00319 00320 default : { 00321 break; 00322 } 00323 00324 } //switch cmd 00325 00326 myled = !myled; 00327 wait(0.3); 00328 } //while 00329 } 00330 #endif 00331 00332 00333 00334 #if (TM1640_TEST == 1) 00335 // Direct TM1640 Test 00336 00337 Serial pc(USBTX, USBRX); 00338 DigitalOut myled(LED1); 00339 00340 // DisplayData_t size is 16 bytes (16 Grids @ 8 Segments) 00341 TM1640::DisplayData_t all_str = {0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF, 0xFF,0xFF}; 00342 TM1640::DisplayData_t cls_str = {0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00, 0x00,0x00}; 00343 00344 TM1640 TM1640(p5,p7); 00345 00346 char cmd, bits; 00347 int main() { 00348 00349 pc.printf("Hello World\r\n"); // 00350 00351 TM1640.cls(); 00352 TM1640.writeData(all_str); 00353 wait(2); 00354 TM1640.setBrightness(TM1640_BRT3); 00355 wait(1); 00356 TM1640.setBrightness(TM1640_BRT0); 00357 wait(1); 00358 TM1640.setBrightness(TM1640_BRT4); 00359 00360 while (1) { 00361 00362 cmd = pc.getc(); 00363 00364 switch cmd { 00365 case '1' : 00366 TM1640.cls(); 00367 TM1640.writeData(all_str); 00368 break; 00369 00370 case '2' : 00371 TM1640.cls(); 00372 TM1640.writeData(cls_str); 00373 break; 00374 00375 case '3' : 00376 00377 #if(1) 00378 //test to show all segs 00379 pc.printf("Show all segs\r\n"); 00380 wait(1); 00381 TM1640.cls(); 00382 00383 for (int i=0; i<TM1640_DISPLAY_MEM; i++) { 00384 for (int bit=0; bit<8; bit++) { 00385 TM1640.cls(); 00386 00387 bits = 0x01 << bit; 00388 TM1640.writeData(bits, i); 00389 00390 pc.printf("Idx = %d, Bits = 0x%02x\r\n", i, bits); 00391 // wait(0.5); 00392 cmd = pc.getc(); // wait for key 00393 } 00394 } 00395 pc.printf("Show all segs done\r\n"); 00396 #endif 00397 break; 00398 00399 case '4' : 00400 TM1640.cls(); 00401 // TM1640.writeData(hello_str); 00402 break; 00403 00404 default : 00405 break; 00406 00407 } //switch cmd 00408 00409 myled = !myled; 00410 wait(0.3); 00411 } //while 00412 } 00413 #endif
Generated on Wed Jul 20 2022 02:48:31 by
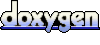