barometric pressure sensor BMP085 http://mbed.org/users/okini3939/notebook/barometric-pressure-sensor-bmp085/ http://mbed.org/users/okini3939/notebook/weatherduino-on-mbed/
Fork of BMP085 by
BMP085.h
00001 /* 00002 * mbed library to use a Bosch Sensortec BMP085/BMP180 sensor 00003 * Copyright (c) 2010 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 */ 00006 00007 /** @file BMP085.h 00008 * @brief mbed library to use a Bosch Sensortec BMP085/BMP180 sensor 00009 * barometric pressure sensor BMP085/BMP180 (Bosch Sensortec) 00010 * interface: I2C digital 00011 */ 00012 00013 #ifndef BMP085_H 00014 #define BMP085_H 00015 00016 #include "mbed.h" 00017 00018 /* 00019 * @brief Pressure at sea level 00020 */ 00021 #define SEA_PRES 1013.25f 00022 00023 /** 00024 * @brief over sampling setting 00025 */ 00026 enum BMP085_oss { 00027 BMP085_oss1 = 0, ///< ultra low power (1 time) 00028 BMP085_oss2 = 1, ///< standard (2 times) 00029 BMP085_oss4 = 2, ///< high resolution (4 times) 00030 BMP085_oss8 = 3 ///< ultra high resolution (8 times) 00031 }; 00032 00033 /** 00034 * @brief BMP085 class 00035 */ 00036 class BMP085 { 00037 public: 00038 BMP085(PinName p_sda, PinName p_scl, BMP085_oss p_oss = BMP085_oss1); 00039 BMP085(I2C& p_i2c, BMP085_oss p_oss = BMP085_oss1); 00040 00041 float get_temperature(); 00042 float get_pressure(); 00043 void update(); 00044 00045 protected: 00046 void init(BMP085_oss); 00047 unsigned short twi_readshort (int, int); 00048 unsigned long twi_readlong (int, int); 00049 void twi_writechar (int, int, int); 00050 00051 I2C i2c; 00052 float temperature; 00053 float pressure; 00054 00055 private: 00056 00057 short ac1, ac2, ac3, b1, b2, mb, mc, md, oss; 00058 unsigned short ac4, ac5, ac6; 00059 }; 00060 00061 #endif
Generated on Wed Jul 13 2022 10:05:58 by
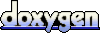