mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
mbed_itm_api.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #if DEVICE_ITM 00019 00020 #include "hal/itm_api.h" 00021 #include "cmsis.h" 00022 00023 #include <stdbool.h> 00024 00025 #ifndef ITM_STIM_FIFOREADY_Msk 00026 #define ITM_STIM_FIFOREADY_Msk 1 00027 #endif 00028 00029 #define ITM_ENABLE_WRITE 0xC5ACCE55 00030 00031 #define SWO_NRZ 0x02 00032 #define SWO_STIMULUS_PORT 0x01 00033 00034 void mbed_itm_init(void) 00035 { 00036 static bool do_init = true; 00037 00038 if (do_init) { 00039 do_init = false; 00040 00041 itm_init(); 00042 00043 /* Enable write access to ITM registers. */ 00044 ITM->LAR = ITM_ENABLE_WRITE; 00045 00046 /* Trace Port Interface Selected Pin Protocol Register. */ 00047 TPI->SPPR = (SWO_NRZ << TPI_SPPR_TXMODE_Pos); 00048 00049 /* Trace Port Interface Formatter and Flush Control Register */ 00050 TPI->FFCR = (1 << TPI_FFCR_TrigIn_Pos); 00051 00052 /* Data Watchpoint and Trace Control Register */ 00053 DWT->CTRL = (1 << DWT_CTRL_CYCTAP_Pos) | 00054 (0xF << DWT_CTRL_POSTINIT_Pos) | 00055 (0xF << DWT_CTRL_POSTPRESET_Pos) | 00056 (1 << DWT_CTRL_CYCCNTENA_Pos); 00057 00058 /* Trace Privilege Register. 00059 * Disable access to trace channel configuration from non-privileged mode. 00060 */ 00061 ITM->TPR = 0x0; 00062 00063 /* Trace Control Register */ 00064 ITM->TCR = (1 << ITM_TCR_TraceBusID_Pos) | 00065 (1 << ITM_TCR_DWTENA_Pos) | 00066 (1 << ITM_TCR_SYNCENA_Pos) | 00067 (1 << ITM_TCR_ITMENA_Pos); 00068 00069 /* Trace Enable Register */ 00070 ITM->TER = SWO_STIMULUS_PORT; 00071 } 00072 } 00073 00074 static void itm_out8(uint32_t port, uint8_t data) 00075 { 00076 /* Wait until port is available */ 00077 while ((ITM->PORT[port].u32 & ITM_STIM_FIFOREADY_Msk) == 0) { 00078 __NOP(); 00079 } 00080 00081 /* write data to port */ 00082 ITM->PORT[port].u8 = data; 00083 } 00084 00085 static void itm_out32(uint32_t port, uint32_t data) 00086 { 00087 /* Wait until port is available */ 00088 while ((ITM->PORT[port].u32 & ITM_STIM_FIFOREADY_Msk) == 0) { 00089 __NOP(); 00090 } 00091 00092 /* write data to port */ 00093 ITM->PORT[port].u32 = data; 00094 } 00095 00096 uint32_t mbed_itm_send(uint32_t port, uint32_t data) 00097 { 00098 /* Check if ITM and port is enabled */ 00099 if (((ITM->TCR & ITM_TCR_ITMENA_Msk) != 0UL) && /* ITM enabled */ 00100 ((ITM->TER & (1UL << port)) != 0UL)) { /* ITM Port enabled */ 00101 itm_out32(port, data); 00102 } 00103 00104 return data; 00105 } 00106 00107 void mbed_itm_send_block(uint32_t port, const void *data, size_t len) 00108 { 00109 const char *ptr = data; 00110 00111 /* Check if ITM and port is enabled */ 00112 if (((ITM->TCR & ITM_TCR_ITMENA_Msk) != 0UL) && /* ITM enabled */ 00113 ((ITM->TER & (1UL << port)) != 0UL)) { /* ITM Port enabled */ 00114 /* Output single byte at a time until data is aligned */ 00115 while ((((uintptr_t) ptr) & 3) && len != 0) { 00116 itm_out8(port, *ptr++); 00117 len--; 00118 } 00119 00120 /* Output bulk of data one word at a time */ 00121 while (len >= 4) { 00122 itm_out32(port, *(const uint32_t *) ptr); 00123 ptr += 4; 00124 len -= 4; 00125 } 00126 00127 /* Output any trailing bytes */ 00128 while (len != 0) { 00129 itm_out8(port, *ptr++); 00130 len--; 00131 } 00132 } 00133 } 00134 #endif // DEVICE_ITM
Generated on Tue Jul 12 2022 20:41:15 by
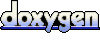