mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
critical_section_api.h
00001 /** \addtogroup hal */ 00002 /** @{*/ 00003 /* mbed Microcontroller Library 00004 * Copyright (c) 2006-2017 ARM Limited 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 */ 00019 #ifndef MBED_CRITICAL_SECTION_API_H 00020 #define MBED_CRITICAL_SECTION_API_H 00021 00022 #include <stdbool.h> 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /** 00029 * \defgroup hal_critical Critical Section HAL functions 00030 * @{ 00031 */ 00032 00033 /** 00034 * Mark the start of a critical section 00035 * 00036 * This function will be called by core_util_critical_section_enter() each time 00037 * the application requests to enter a critical section. The purpose of the 00038 * critical section is to ensure mutual-exclusion synchronisation of the 00039 * processor by preventing any change in processor control, the default 00040 * behaviour requires storing the state of interrupts in the system before 00041 * disabling them. 00042 * 00043 * The critical section code supports nesting. When a thread has entered a 00044 * critical section it can make additional calls to 00045 * core_util_critical_section_enter() without deadlocking itself. The critical 00046 * section driver API tracks the number of nested calls to the critical section. 00047 * The critical section will only be exited when 00048 * core_util_critical_section_exit() has been called once for each time it 00049 * entered the critical section. 00050 * 00051 * On the first call to enter a critical section this function MUST store the 00052 * state of any interrupts or other application settings it will modify to 00053 * facilitate the critical section. 00054 * 00055 * Each successive call to enter the critical section MUST ignore storing or 00056 * modifying any application state. 00057 * 00058 * The default implementation of this function which will save the current state 00059 * of interrupts before disabling them. This implementation can be found in 00060 * mbed_critical_section_api.c. This behaviour is can be overridden on a per 00061 * platform basis by providing a different implementation within the correct 00062 * targets directory. 00063 */ 00064 void hal_critical_section_enter(void); 00065 00066 00067 /** Mark the end of a critical section. 00068 * 00069 * The purpose of this function is to restore any state that was modified upon 00070 * entering the critical section, allowing other threads or interrupts to change 00071 * the processor control. 00072 * 00073 * This function will be called once by core_util_critical_section_exit() per 00074 * critical section on last call to exit. When called, the application MUST 00075 * restore the saved interrupt/application state that was saved when entering 00076 * the critical section. 00077 * 00078 * There is a default implementation of this function, it will restore the state 00079 * of interrupts that were previously saved when hal_critical_section_enter was 00080 * first called, this implementation can be found in 00081 * mbed_critical_section_api.c. This behaviour is overridable by providing a 00082 * different function implementation within the correct targets directory. 00083 */ 00084 void hal_critical_section_exit(void); 00085 00086 00087 /** Determine if the application is currently running in a critical section 00088 * 00089 * The purpose of this function is to inform the caller whether or not the 00090 * application is running in a critical section. This is done by checking if 00091 * the current interrupt state has been saved in the underlying implementation, 00092 * this could also be done by checking the state of the interrupts at the time 00093 * of calling. 00094 * 00095 * @return True if running in a critical section, false if not. 00096 */ 00097 bool hal_in_critical_section(void); 00098 00099 00100 /**@}*/ 00101 00102 #ifdef __cplusplus 00103 } 00104 #endif 00105 00106 #endif // MBED_CRITICAL_SECTION_API_H 00107 00108 /** @}*/
Generated on Tue Jul 12 2022 20:41:14 by
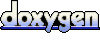