mbed library sources. Supersedes mbed-src.
Dependents: Nucleo_Hello_Encoder BLE_iBeaconScan AM1805_DEMO DISCO-F429ZI_ExportTemplate1 ... more
Span class
[Platform]
Data Structures | |
struct | Span< ElementType, Extent > |
Nonowning view to a sequence of contiguous elements. More... | |
struct | Span< ElementType, SPAN_DYNAMIC_EXTENT > |
Span specialization that handle dynamic size. More... | |
Functions | |
template<typename T , ptrdiff_t LhsExtent, ptrdiff_t RhsExtent> | |
bool | operator== (const Span< T, LhsExtent > &lhs, T(&rhs)[RhsExtent]) |
Equality operation between a Span and a reference to a C++ array. | |
template<typename T , ptrdiff_t LhsExtent, ptrdiff_t RhsExtent> | |
bool | operator== (T(&lhs)[LhsExtent], const Span< T, RhsExtent > &rhs) |
Equality operation between a Span and a reference to a C++ array. | |
template<typename T , ptrdiff_t LhsExtent, ptrdiff_t RhsExtent> | |
bool | operator!= (const Span< T, LhsExtent > &lhs, T(&rhs)[RhsExtent]) |
Not Equal operation between a Span and a reference to a C++ array. | |
template<typename T , ptrdiff_t LhsExtent, ptrdiff_t RhsExtent> | |
bool | operator!= (T(&lhs)[LhsExtent], const Span< T, RhsExtent > &rhs) |
Not Equal operation between a Span and a reference to a C++ array. | |
template<ptrdiff_t Extent, typename T > | |
Span< T, Extent > | make_Span (T *elements) |
Generate a Span from a pointer to a C/C++ array. | |
template<typename T , size_t Extent> | |
Span< const T, Extent > | make_const_Span (const T(&elements)[Extent]) |
Generate a Span to a const content from a reference to a C/C++ array. | |
template<typename T , typename U , ptrdiff_t LhsExtent, ptrdiff_t RhsExtent> | |
bool | operator== (const Span< T, LhsExtent > &lhs, const Span< U, RhsExtent > &rhs) |
Equality operator between two Span objects. | |
template<typename T , typename U , ptrdiff_t LhsExtent, ptrdiff_t RhsExtent> | |
bool | operator!= (const Span< T, LhsExtent > &lhs, const Span< U, RhsExtent > &rhs) |
Not equal operator. | |
template<typename T , size_t Size> | |
Span< T, Size > | make_Span (T(&elements)[Size]) |
Generate a Span from a reference to a C/C++ array. | |
template<typename T > | |
Span< T > | make_Span (T *array_ptr, ptrdiff_t array_size) |
Generate a Span from a C/C++ pointer and the size of the array. | |
template<size_t Extent, typename T > | |
Span< const T, Extent > | make_const_Span (const T *elements) |
Generate a Span to a const content from a pointer to a C/C++ array. | |
template<typename T > | |
Span< const T > | make_const_Span (T *array_ptr, size_t array_size) |
Generate a Span to a const content from a C/C++ pointer and the size of the array. | |
const ptrdiff_t | SPAN_DYNAMIC_EXTENT |
Special value for the Extent parameter of Span. | |
Variables | |
const ptrdiff_t | SPAN_DYNAMIC_EXTENT = -1 |
Special value for the Extent parameter of Span. |
Function Documentation
Span< const T, Extent > make_const_Span | ( | const T(&) | elements[Extent] ) |
Generate a Span to a const content from a reference to a C/C++ array.
- Template Parameters:
-
T Type of elements held in elements. Extent Number of items held in elements.
- Parameters:
-
elements The array viewed.
- Returns:
- The Span to elements.
- Note:
- This helper avoids the typing of template parameter when Span is created 'inline'.
Definition at line 971 of file cmsis/BUILD/mbed/platform/Span.h.
Span< const T, Extent > make_const_Span | ( | const T * | elements ) | [related, inherited] |
Generate a Span to a const content from a pointer to a C/C++ array.
- Template Parameters:
-
Extent Number of items held in elements. T Type of elements held in elements.
- Parameters:
-
elements The reference to the array viewed.
- Returns:
- The Span to elements.
- Note:
- This helper avoids the typing of template parameter when Span is created 'inline'.
Definition at line 992 of file cmsis/BUILD/mbed/platform/Span.h.
Span< const T > make_const_Span | ( | T * | array_ptr, |
size_t | array_size | ||
) | [related, inherited] |
Generate a Span to a const content from a C/C++ pointer and the size of the array.
- Template Parameters:
-
T Type of elements held in array_ptr.
- Parameters:
-
array_ptr The pointer to the array to viewed. array_size The number of T elements in the array.
- Returns:
- The Span to array_ptr with a size of array_size.
- Note:
- This helper avoids the typing of template parameter when Span is created 'inline'.
Definition at line 1014 of file cmsis/BUILD/mbed/platform/Span.h.
Span< T, Size > make_Span | ( | T(&) | elements[Size] ) | [related, inherited] |
Generate a Span from a reference to a C/C++ array.
- Template Parameters:
-
T Type of elements held in elements. Extent Number of items held in elements.
- Parameters:
-
elements The reference to the array viewed.
- Returns:
- The Span to elements.
- Note:
- This helper avoids the typing of template parameter when Span is created 'inline'.
Definition at line 913 of file cmsis/BUILD/mbed/platform/Span.h.
Span< T > make_Span | ( | T * | array_ptr, |
ptrdiff_t | array_size | ||
) | [related, inherited] |
Generate a Span from a C/C++ pointer and the size of the array.
- Template Parameters:
-
T Type of elements held in array_ptr.
- Parameters:
-
array_ptr The pointer to the array viewed. array_size The number of T elements in the array.
- Returns:
- The Span to array_ptr with a size of array_size.
- Note:
- This helper avoids the typing of template parameter when Span is created 'inline'.
Definition at line 953 of file cmsis/BUILD/mbed/platform/Span.h.
Span< T, Extent > make_Span | ( | T * | elements ) |
Generate a Span from a pointer to a C/C++ array.
- Template Parameters:
-
Extent Number of items held in elements. T Type of elements held in elements.
- Parameters:
-
elements The reference to the array viewed.
- Returns:
- The Span to elements.
- Note:
- This helper avoids the typing of template parameter when Span is created 'inline'.
Definition at line 932 of file cmsis/BUILD/mbed/platform/Span.h.
bool operator!= | ( | const Span< T, LhsExtent > & | lhs, |
T(&) | rhs[RhsExtent] | ||
) |
Not Equal operation between a Span and a reference to a C++ array.
- Parameters:
-
lhs Left side of the binary operation. rhs Right side of the binary operation.
- Returns:
- True if elements in input have the same size and the same content and false otherwise.
Definition at line 877 of file cmsis/BUILD/mbed/platform/Span.h.
bool operator!= | ( | const Span< T, LhsExtent > & | lhs, |
const Span< U, RhsExtent > & | rhs | ||
) | [related, inherited] |
Not equal operator.
- Parameters:
-
lhs Left side of the binary operation. rhs Right side of the binary operation.
- Returns:
- True if arrays in input do not have the same size or the same content and false otherwise.
Definition at line 862 of file cmsis/BUILD/mbed/platform/Span.h.
bool operator!= | ( | T(&) | lhs[LhsExtent], |
const Span< T, RhsExtent > & | rhs | ||
) |
Not Equal operation between a Span and a reference to a C++ array.
- Parameters:
-
lhs Left side of the binary operation. rhs Right side of the binary operation.
- Returns:
- True if elements in input have the same size and the same content and false otherwise.
Definition at line 892 of file cmsis/BUILD/mbed/platform/Span.h.
bool operator== | ( | const Span< T, LhsExtent > & | lhs, |
const Span< U, RhsExtent > & | rhs | ||
) | [related, inherited] |
Equality operator between two Span objects.
- Parameters:
-
lhs Left side of the binary operation. rhs Right side of the binary operation.
- Returns:
- True if Spans in input have the same size and the same content and false otherwise.
Definition at line 805 of file cmsis/BUILD/mbed/platform/Span.h.
bool operator== | ( | T(&) | lhs[LhsExtent], |
const Span< T, RhsExtent > & | rhs | ||
) |
Equality operation between a Span and a reference to a C++ array.
- Parameters:
-
lhs Left side of the binary operation. rhs Right side of the binary operation.
- Returns:
- True if elements in input have the same size and the same content and false otherwise.
Definition at line 845 of file cmsis/BUILD/mbed/platform/Span.h.
bool operator== | ( | const Span< T, LhsExtent > & | lhs, |
T(&) | rhs[RhsExtent] | ||
) |
Equality operation between a Span and a reference to a C++ array.
- Parameters:
-
lhs Left side of the binary operation. rhs Right side of the binary operation.
- Returns:
- True if elements in input have the same size and the same content and false otherwise.
Definition at line 830 of file cmsis/BUILD/mbed/platform/Span.h.
const ptrdiff_t SPAN_DYNAMIC_EXTENT [related, inherited] |
Special value for the Extent parameter of Span.
If the type uses this value, then the size of the array is stored in the object at runtime.
Definition at line 67 of file platform/Span.h.
Variable Documentation
const ptrdiff_t SPAN_DYNAMIC_EXTENT = -1 [related, inherited] |
Special value for the Extent parameter of Span.
If the type uses this value, then the size of the array is stored in the object at runtime.
Definition at line 67 of file cmsis/BUILD/mbed/platform/Span.h.
Generated on Tue Jul 12 2022 20:41:16 by
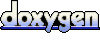