Receiver interface for the fischertechnik IR control set
Embed:
(wiki syntax)
Show/hide line numbers
FtControlSetReceiver.h
00001 #ifndef FTCONTROLSETRECEIVER_H 00002 #define FTCONTROLSETRECEIVER_H 00003 00004 #include "FtControlSetMessage.h" 00005 #include "InterruptIn.h" 00006 #include "FunctionPointer.h" 00007 #include "TimeoutTweaked.h" 00008 00009 using namespace mbed; 00010 00011 /// Receiver interface for the fischertechnik IR control set 00012 /// 00013 /// An mbed port of the code found on this <A HREF="http://www.ftcommunity.de/wiki.php?action=show&topic_id=36">ft community WIKI page</a> 00014 /// The ft IR remote control uses some kind of <A HREF="http://www.sbprojects.com/knowledge/ir/rcmm.php"> RC-MM Protocol </A>. 00015 /// When any of the controls is applied the remote control sends updates at a rate of at 1/120ms or 1/90ms depending on the senders frequency setting. 00016 /// Each message delivers the complete remote control status encoded into 30 bits. 00017 /// The structure of the message can be seen in FtControlSetMessage.h. 00018 /// The protocol uses Pulse Distance Modulation (PDM) with two bits (di-bit) per pulse, hence four different 00019 /// distances. A transmission consists of 16 pulses (15 distances) for a total of 30 bits. 00020 /// 00021 /// I assume that the ft control set works fine with standard 38kHz IR detectors. I have used a CHQ0038 from a broken DVD Player. 00022 /// It can be onnected directly to the mebed: GND, VCC->3.3V and the signal line to any of the numbered mbed gpios. 00023 /// 00024 /// The PDM timing of the ft IR control set isn't that exact. Thus receive errors occur quite frequently. I am observing an error rate somewhere between 00025 /// 3% and 5%. This driver "ignores" up to 3 consecutive receive errors, i.e. it just indicates an error but still provides 00026 /// the last correctly received message, before it resets the message to zero after the fourth error. 00027 /// 00028 /// Some fluorescent energy saving lamp might disturb the transmision quite heavily. 00029 00030 00031 class FtControlSetReceiver 00032 { 00033 InterruptIn m_pulseIRQ; 00034 00035 uint32_t m_rxBuffer; /// receive message buffer 00036 uint32_t m_nPulses; /// receive pulse counter (15 pulses per message) 00037 uint32_t m_timeOfLastEdge; /// signal edge time stamp memory 00038 uint8_t m_nOnes; /// number of received high bits for parity calculation 00039 00040 volatile uint32_t m_errorCount; /// counter of observed receive errors 00041 uint8_t m_nGracefullyIgnoredErrors; /// error counter that reset on successful message reception 00042 00043 volatile uint32_t m_lastMessage; /// last message received correctly 00044 00045 FunctionPointer m_callback; ///callback for user code that is called afetr successful message reception 00046 00047 TimeoutTweaked m_messageTimeout; /// Timeout for message reset when sender is inactive 00048 TimeoutTweaked m_receiveTimeout; /// receive timeout 00049 TimeoutTweaked m_recoverTimeout; /// error recovery timeout 00050 00051 static const uint32_t c_pulseWidthLimits[5]; /// di-bit discrimination times 00052 static const uint32_t c_pulseWidthDelta = 100;//101; /// di-bit discrimination pulse width delta 00053 static const uint32_t c_pulseWidthOffset = 795;//793; /// di-bit common pulse width base 00054 static const uint32_t c_maxPulseTime = c_pulseWidthOffset+7*c_pulseWidthDelta/2; /// reveive timeout 00055 static const uint32_t c_messageTimeout[2]; /// message timeout depends on selected sender frequency 00056 static const uint8_t c_errorsToBeGracefullyIgnored = 3; /// number of consecutive errors after which the message is reset 00057 static const uint32_t c_recoverTimeout = c_maxPulseTime; /// receiver is deactivated for this time after a receive error (IRQ burst suppression) 00058 00059 void pulseISR(); /// pulse handler 00060 void messageTimeoutISR(); /// message time out handler 00061 void recoverISR(); /// reactivates the pulse handler after recover timeout 00062 00063 void handleReceiveError(); 00064 00065 public: 00066 00067 /// Create a receiver 00068 /// @parameter in: The pin the IR detector's message line is connected to 00069 FtControlSetReceiver(PinName in); 00070 00071 /// get the last received message 00072 FtControlSetMessage getMessage()const { 00073 return FtControlSetMessage(m_lastMessage); 00074 }; 00075 00076 /// get number of receive errors 00077 uint32_t getErrorCount()const { 00078 return m_errorCount; 00079 } 00080 00081 /// hook a call back into the receiver ISR e.g. for sending a RTOS message. 00082 /// called on each update or receive error 00083 void setCallback(const FunctionPointer& callback) { 00084 m_callback=callback; 00085 }; 00086 }; 00087 00088 00089 #endif 00090 00091 00092 00093 00094 00095 00096
Generated on Fri Jul 15 2022 03:15:46 by
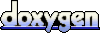