
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "Motor.h" 00003 00004 /* 00005 * This code reads command files to control the motors. 00006 * The file name it reads is command.txt 00007 * 00008 * The file format is : 00009 * 00010 * <command> <data> 00011 * 00012 * The commands are : 00013 * 00014 * F - Forward, both motor on full speed forwards 00015 * B - Backwards, both motors on full speed in reverse 00016 * L - Left, left motor full speed backwards, right motor full speed forward 00017 * R - Right, right motor full speed backwards, left motor full speed forward 00018 * W - Wait, both motors stop 00019 * 00020 * Data : 00021 * 00022 * Data is a time in milliseconds to carry out the command. 00023 * 00024 * Example : 00025 * 00026 * F 1000 00027 * W 200 00028 * L 1000 00029 * W 200 00030 * R 1000 00031 * W 200 00032 * B 1000 00033 * W 200 00034 */ 00035 00036 00037 LocalFileSystem local("local"); 00038 00039 Serial pc(USBTX, USBRX); 00040 00041 BusOut leds(LED1,LED2,LED3,LED4); 00042 00043 Motor left(p23, p6, p5); 00044 Motor right(p24, p8, p7); 00045 00046 00047 int main() { 00048 00049 leds = 0x0; 00050 00051 // flash the LED 3 time before starting 00052 for (int i=0; i < 3 ; i++) { 00053 leds = 0x1; 00054 wait (0.5); 00055 leds = 0x0; 00056 wait (0.5); 00057 } 00058 00059 // Open the command.txt file 00060 FILE *commandfile = fopen("/local/command.txt", "r"); 00061 00062 // If there is no file, sit flashing the LEDs 00063 if (commandfile == NULL) { 00064 while(1) { 00065 leds = 0xf; 00066 wait(0.5); 00067 leds = 0x0; 00068 wait(0.5); 00069 } 00070 } 00071 00072 // process each of the commands in the file 00073 while (!feof(commandfile)) { 00074 00075 char command = 0; 00076 int data = 0.0; 00077 00078 // Read from the command file 00079 fscanf(commandfile, "%c %d\n", &command, &data); 00080 00081 if((command=='f') || (command=='F')){ 00082 left.speed(1); 00083 right.speed(1); 00084 } 00085 else if((command=='b') || (command=='B')){ 00086 left.speed(-1); 00087 right.speed(-1); 00088 } 00089 else if((command=='l') || (command=='L')){ 00090 left.speed(-1); 00091 right.speed(1); 00092 } 00093 else if ((command=='r') || (command=='R')){ 00094 left.speed(1); 00095 right.speed(-1); 00096 } 00097 // Wait commands 00098 else if ((command=='w') || (command=='W')){ 00099 } 00100 00101 // wait for the length of the command 00102 wait(data/1000.0); 00103 00104 // Switch the motors off 00105 left.speed(0); 00106 right.speed(0); 00107 00108 00109 } // this is the end of the file while loop 00110 00111 00112 for (int i=0;i<10;i++) { 00113 leds = 0x0; 00114 wait (0.2); 00115 leds = 0xf; 00116 wait (0.2); 00117 } 00118 00119 fclose(commandfile); 00120 exit(0); 00121 00122 } // end of main
Generated on Thu Jul 14 2022 03:22:15 by
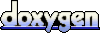