Library for controlling a strip of Adafruit NeoPixels with WS2812 drivers. Because of the strict timing requirements of the self-clocking data signal, the critical parts of code are written in ARM assembly. Currently, only the NXP LPC1768 platform is supported. More information about NeoPixels can be found at http://learn.adafruit.com/adafruit-neopixel-uberguide/overview
Dependents: NeoPixels ECE4180_Werable_LCD_DEMO neopixel_spi NeoPixels ... more
NeoStrip Class Reference
NeoStrip objects manage the buffering and assigning of addressable NeoPixels. More...
#include <NeoStrip.h>
Public Member Functions | |
NeoStrip (PinName pin, int N) | |
Create a NeoStrip object. | |
void | setBrightness (float bright) |
Set an overall brightness scale for the entire strip. | |
void | setPixel (int p, int color) |
Set a single pixel to the specified color. | |
void | setPixel (int p, uint8_t red, uint8_t green, uint8_t blue) |
Set a single pixel to the specified color, with red, green, and blue values in separate arguments. | |
void | setPixels (int p, int n, const int *colors) |
Set n pixels starting at pixel p. | |
void | clear () |
Reset all pixels in the strip to be of (0x000000) | |
void | write () |
Write the colors out to the strip; this method must be called to see any hardware effect. |
Detailed Description
NeoStrip objects manage the buffering and assigning of addressable NeoPixels.
Definition at line 31 of file NeoStrip.h.
Constructor & Destructor Documentation
NeoStrip | ( | PinName | pin, |
int | N | ||
) |
Create a NeoStrip object.
- Parameters:
-
pin The mbed data pin name N The number of pixels in the strip
Definition at line 25 of file NeoStrip.cpp.
Member Function Documentation
void clear | ( | ) |
Reset all pixels in the strip to be of (0x000000)
Definition at line 75 of file NeoStrip.cpp.
void setBrightness | ( | float | bright ) |
Set an overall brightness scale for the entire strip.
When colors are set using setPixel(), they are scaled according to this brightness.
Because NeoPixels are very bright and draw a lot of current, the brightness is set to 0.5 by default.
- Parameters:
-
bright The brightness scale between 0 and 1.0
Definition at line 41 of file NeoStrip.cpp.
void setPixel | ( | int | p, |
int | color | ||
) |
Set a single pixel to the specified color.
This method (and all other setPixel methods) uses modulo-N arithmetic when addressing pixels. If p >= N, the pixel address will wrap around.
- Parameters:
-
p The pixel number (starting at 0) to set color A 24-bit color packed into a single int, using standard hex color codes (e.g. 0xFF0000 is red)
Definition at line 46 of file NeoStrip.cpp.
void setPixel | ( | int | p, |
uint8_t | red, | ||
uint8_t | green, | ||
uint8_t | blue | ||
) |
Set a single pixel to the specified color, with red, green, and blue values in separate arguments.
Definition at line 54 of file NeoStrip.cpp.
void setPixels | ( | int | p, |
int | n, | ||
const int * | colors | ||
) |
Set n pixels starting at pixel p.
- Parameters:
-
p The first pixel in the strip to set. n The number of pixels to set. colors An array of length n containing the 24-bit colors for each pixel
Definition at line 63 of file NeoStrip.cpp.
void write | ( | ) |
Write the colors out to the strip; this method must be called to see any hardware effect.
This function disables interrupts while the strip data is being sent, each pixel takes approximately 30us to send, plus a 50us reset pulse at the end.
Definition at line 85 of file NeoStrip.cpp.
Generated on Tue Jul 12 2022 18:57:23 by
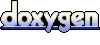