fork
Fork of cpputest by
Embed:
(wiki syntax)
Show/hide line numbers
TestFailure.h
00001 /* 00002 * Copyright (c) 2007, Michael Feathers, James Grenning and Bas Vodde 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions are met: 00007 * * Redistributions of source code must retain the above copyright 00008 * notice, this list of conditions and the following disclaimer. 00009 * * Redistributions in binary form must reproduce the above copyright 00010 * notice, this list of conditions and the following disclaimer in the 00011 * documentation and/or other materials provided with the distribution. 00012 * * Neither the name of the <organization> nor the 00013 * names of its contributors may be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE EARLIER MENTIONED AUTHORS ``AS IS'' AND ANY 00017 * EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00019 * DISCLAIMED. IN NO EVENT SHALL <copyright holder> BE LIABLE FOR ANY 00020 * DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00021 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00022 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00023 * ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00024 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00025 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00026 */ 00027 00028 /////////////////////////////////////////////////////////////////////////////// 00029 // 00030 // Failure is a class which holds information for a specific 00031 // test failure. It can be overriden for more complex failure messages 00032 // 00033 /////////////////////////////////////////////////////////////////////////////// 00034 00035 00036 #ifndef D_TestFailure_H 00037 #define D_TestFailure_H 00038 00039 #include "SimpleString.h" 00040 00041 class UtestShell; 00042 class TestOutput; 00043 00044 class TestFailure 00045 { 00046 00047 public: 00048 TestFailure(UtestShell*, const char* fileName, int lineNumber, 00049 const SimpleString& theMessage); 00050 TestFailure(UtestShell*, const SimpleString& theMessage); 00051 TestFailure(UtestShell*, const char* fileName, int lineNumber); 00052 TestFailure(const TestFailure&); 00053 virtual ~TestFailure(); 00054 00055 virtual SimpleString getFileName() const; 00056 virtual SimpleString getTestName() const; 00057 virtual int getFailureLineNumber() const; 00058 virtual SimpleString getMessage() const; 00059 virtual SimpleString getTestFileName() const; 00060 virtual int getTestLineNumber() const; 00061 bool isOutsideTestFile() const; 00062 bool isInHelperFunction() const; 00063 00064 00065 protected: 00066 00067 SimpleString createButWasString(const SimpleString& expected, const SimpleString& actual); 00068 SimpleString createDifferenceAtPosString(const SimpleString& actual, size_t position); 00069 00070 SimpleString testName_; 00071 SimpleString fileName_; 00072 int lineNumber_; 00073 SimpleString testFileName_; 00074 int testLineNumber_; 00075 SimpleString message_; 00076 00077 TestFailure& operator=(const TestFailure&); 00078 00079 }; 00080 00081 class EqualsFailure: public TestFailure 00082 { 00083 public: 00084 EqualsFailure(UtestShell*, const char* fileName, int lineNumber, const char* expected, const char* actual); 00085 EqualsFailure(UtestShell*, const char* fileName, int lineNumber, const SimpleString& expected, const SimpleString& actual); 00086 }; 00087 00088 class DoublesEqualFailure: public TestFailure 00089 { 00090 public: 00091 DoublesEqualFailure(UtestShell*, const char* fileName, int lineNumber, double expected, double actual, double threshold); 00092 }; 00093 00094 class CheckEqualFailure : public TestFailure 00095 { 00096 public: 00097 CheckEqualFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& expected, const SimpleString& actual); 00098 }; 00099 00100 class ContainsFailure: public TestFailure 00101 { 00102 public: 00103 ContainsFailure(UtestShell*, const char* fileName, int lineNumber, const SimpleString& expected, const SimpleString& actual); 00104 00105 }; 00106 00107 class CheckFailure : public TestFailure 00108 { 00109 public: 00110 CheckFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& checkString, const SimpleString& conditionString, const SimpleString& textString = ""); 00111 }; 00112 00113 class FailFailure : public TestFailure 00114 { 00115 public: 00116 FailFailure(UtestShell* test, const char* fileName, int lineNumber, const SimpleString& message); 00117 }; 00118 00119 class LongsEqualFailure : public TestFailure 00120 { 00121 public: 00122 LongsEqualFailure(UtestShell* test, const char* fileName, int lineNumber, long expected, long actual); 00123 }; 00124 00125 class UnsignedLongsEqualFailure : public TestFailure 00126 { 00127 public: 00128 UnsignedLongsEqualFailure(UtestShell* test, const char* fileName, int lineNumber, unsigned long expected, unsigned long actual); 00129 }; 00130 00131 class StringEqualFailure : public TestFailure 00132 { 00133 public: 00134 StringEqualFailure(UtestShell* test, const char* fileName, int lineNumber, const char* expected, const char* actual); 00135 }; 00136 00137 class StringEqualNoCaseFailure : public TestFailure 00138 { 00139 public: 00140 StringEqualNoCaseFailure(UtestShell* test, const char* fileName, int lineNumber, const char* expected, const char* actual); 00141 }; 00142 00143 #endif
Generated on Tue Jul 12 2022 21:37:56 by
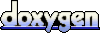