fork
Fork of cpputest by
Embed:
(wiki syntax)
Show/hide line numbers
MemoryLeakDetectorNewMacros.h
00001 00002 /* 00003 * This file can be used to get extra debugging information about memory leaks in your production code. 00004 * It defines a preprocessor macro for operator new. This will pass additional information to the 00005 * operator new and this will give the line/file information of the memory leaks in your code. 00006 * 00007 * You can use this by including this file to all your production code. When using gcc, you can use 00008 * the -include file to do this for you. 00009 * 00010 * Warning: Using the new macro can cause a conflict with newly declared operator news. This can be 00011 * resolved by: 00012 * 1. #undef operator new before including this file 00013 * 2. Including the files that override operator new before this file. 00014 * This can be done by creating your own NewMacros.h file that includes your operator new overrides 00015 * and THEN this file. 00016 * 00017 * STL (or StdC++ lib) also does overrides for operator new. Therefore, you'd need to include the STL 00018 * files *before* this file too. 00019 * 00020 */ 00021 00022 #include "CppUTestConfig.h" 00023 00024 /* Make sure that mem leak detection is on and that this is being included from a C++ file */ 00025 #if CPPUTEST_USE_MEM_LEAK_DETECTION && defined(__cplusplus) 00026 00027 /* This #ifndef prevents <new> from being included twice and enables the file to be included anywhere */ 00028 #ifndef CPPUTEST_USE_NEW_MACROS 00029 00030 #if CPPUTEST_USE_STD_CPP_LIB 00031 #include <new> 00032 #include <memory> 00033 #include <string> 00034 #endif 00035 00036 void* operator new(size_t size, const char* file, int line) UT_THROW (std::bad_alloc); 00037 void* operator new[](size_t size, const char* file, int line) UT_THROW (std::bad_alloc); 00038 void* operator new(size_t size) UT_THROW(std::bad_alloc); 00039 void* operator new[](size_t size) UT_THROW(std::bad_alloc); 00040 00041 void operator delete(void* mem) UT_NOTHROW; 00042 void operator delete[](void* mem) UT_NOTHROW; 00043 void operator delete(void* mem, const char* file, int line) UT_NOTHROW; 00044 void operator delete[](void* mem, const char* file, int line) UT_NOTHROW; 00045 00046 #endif 00047 00048 #define new new(__FILE__, __LINE__) 00049 00050 #define CPPUTEST_USE_NEW_MACROS 1 00051 00052 #endif
Generated on Tue Jul 12 2022 21:37:56 by
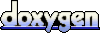