fork
Fork of cpputest by
Embed:
(wiki syntax)
Show/hide line numbers
MemoryLeakDetectorMallocMacros.h
00001 00002 /* 00003 * This file can be used to get extra debugging information about memory leaks in your production code. 00004 * It defines a preprocessor macro for malloc. This will pass additional information to the 00005 * malloc and this will give the line/file information of the memory leaks in your code. 00006 * 00007 * You can use this by including this file to all your production code. When using gcc, you can use 00008 * the -include file to do this for you. 00009 * 00010 */ 00011 00012 #include "CppUTestConfig.h" 00013 00014 #if CPPUTEST_USE_MEM_LEAK_DETECTION 00015 00016 /* This prevents the declaration from done twice and makes sure the file only #defines malloc, so it can be included anywhere */ 00017 #ifndef CPPUTEST_USE_MALLOC_MACROS 00018 00019 #ifdef __cplusplus 00020 extern "C" 00021 { 00022 #endif 00023 00024 extern void* cpputest_malloc_location(size_t size, const char* file, int line); 00025 extern void* cpputest_calloc_location(size_t count, size_t size, const char* file, int line); 00026 extern void* cpputest_realloc_location(void *, size_t, const char* file, int line); 00027 extern void cpputest_free_location(void* buffer, const char* file, int line); 00028 00029 #ifdef __cplusplus 00030 } 00031 #endif 00032 00033 extern void crash_on_allocation_number(unsigned number); 00034 00035 #endif 00036 00037 /* NOTE on strdup! 00038 * 00039 * strdup was implemented earlier, however it is *not* an Standard C function but a POSIX function. 00040 * Because of that, it can lead to portability issues by providing more than is available on the local platform. 00041 * For that reason, strdup is *not* implemented as a macro. If you still want to use it, an easy implementation would be: 00042 * 00043 * size_t length = 1 + strlen(str); 00044 * char* result = (char*) cpputest_malloc_location(length, file, line); 00045 * memcpy(result, str, length); 00046 * return result; 00047 * 00048 */ 00049 00050 #define malloc(a) cpputest_malloc_location(a, __FILE__, __LINE__) 00051 #define calloc(a, b) cpputest_calloc_location(a, b, __FILE__, __LINE__) 00052 #define realloc(a, b) cpputest_realloc_location(a, b, __FILE__, __LINE__) 00053 #define free(a) cpputest_free_location(a, __FILE__, __LINE__) 00054 00055 #define CPPUTEST_USE_MALLOC_MACROS 1 00056 #endif
Generated on Tue Jul 12 2022 21:37:56 by
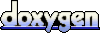