
Setup and test the ESP8266 Wi Fi SOC from Sparkfun. Sets SSID and PASSWORD and prints status messages. For use on mbed LPC1768. Also reports IP and MAC address. See https://developer.mbed.org/users/4180_1/notebook/using-the-esp8266-with-the-mbed-lpc1768/
Fork of ESP8266-configuaration-baudrate by
main.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); 00004 Serial esp(p28, p27); // tx, rx 00005 DigitalOut reset(p26); 00006 Timer t; 00007 00008 int count,ended,timeout; 00009 char buf[1024]; 00010 char snd[255]; 00011 00012 char ssid[32] = "mySSID"; // enter WiFi router ssid inside the quotes 00013 char pwd [32] = "myPASSWORD"; // enter WiFi router password inside the quotes 00014 00015 void SendCMD(),getreply(),ESPconfig(),ESPsetbaudrate(); 00016 00017 00018 int main() 00019 { 00020 reset=0; //hardware reset for 8266 00021 pc.baud(115200); // set what you want here depending on your terminal program speed 00022 pc.printf("\f\n\r-------------ESP8266 Hardware Reset-------------\n\r"); 00023 wait(0.5); 00024 reset=1; 00025 timeout=2; 00026 getreply(); 00027 00028 esp.baud(115200); // change this to the new ESP8266 baudrate if it is changed at any time. 00029 00030 //ESPsetbaudrate(); //****************** include this routine to set a different ESP8266 baudrate ****************** 00031 00032 ESPconfig(); //****************** include Config to set the ESP8266 configuration *********************** 00033 00034 00035 00036 // continuosly get AP list and IP 00037 while(1) { 00038 pc.printf("\n---------- Listing Acces Points ----------\r\n"); 00039 strcpy(snd, "AT+CWLAP\r\n"); 00040 SendCMD(); 00041 timeout=15; 00042 getreply(); 00043 pc.printf(buf); 00044 wait(2); 00045 pc.printf("\n---------- Get IP and MAC ----------\r\n"); 00046 strcpy(snd, "AT+CIFSR\r\n"); 00047 SendCMD(); 00048 timeout=10; 00049 getreply(); 00050 pc.printf(buf); 00051 wait(2); 00052 } 00053 00054 } 00055 00056 // Sets new ESP8266 baurate, change the esp.baud(xxxxx) to match your new setting once this has been executed 00057 void ESPsetbaudrate() 00058 { 00059 strcpy(snd, "AT+CIOBAUD=115200\r\n"); // change the numeric value to the required baudrate 00060 SendCMD(); 00061 } 00062 00063 // +++++++++++++++++++++++++++++++++ This is for ESP8266 config only, run this once to set up the ESP8266 +++++++++++++++ 00064 void ESPconfig() 00065 { 00066 wait(5); 00067 strcpy(snd,"AT\r\n"); 00068 SendCMD(); 00069 wait(1); 00070 strcpy(snd,"AT\r\n"); 00071 SendCMD(); 00072 wait(1); 00073 strcpy(snd,"AT\r\n"); 00074 SendCMD(); 00075 timeout=1; 00076 getreply(); 00077 wait(1); 00078 pc.printf("\f---------- Starting ESP Config ----------\r\n\n"); 00079 00080 pc.printf("---------- Reset & get Firmware ----------\r\n"); 00081 strcpy(snd,"AT+RST\r\n"); 00082 SendCMD(); 00083 timeout=5; 00084 getreply(); 00085 pc.printf(buf); 00086 00087 wait(2); 00088 00089 pc.printf("\n---------- Get Version ----------\r\n"); 00090 strcpy(snd,"AT+GMR\r\n"); 00091 SendCMD(); 00092 timeout=4; 00093 getreply(); 00094 pc.printf(buf); 00095 00096 wait(3); 00097 00098 // set CWMODE to 1=Station,2=AP,3=BOTH, default mode 1 (Station) 00099 pc.printf("\n---------- Setting Mode ----------\r\n"); 00100 strcpy(snd, "AT+CWMODE=1\r\n"); 00101 SendCMD(); 00102 timeout=4; 00103 getreply(); 00104 pc.printf(buf); 00105 00106 wait(2); 00107 00108 // set CIPMUX to 0=Single,1=Multi 00109 pc.printf("\n---------- Setting Connection Mode ----------\r\n"); 00110 strcpy(snd, "AT+CIPMUX=1\r\n"); 00111 SendCMD(); 00112 timeout=4; 00113 getreply(); 00114 pc.printf(buf); 00115 00116 wait(2); 00117 00118 pc.printf("\n---------- Listing Access Points ----------\r\n"); 00119 strcpy(snd, "AT+CWLAP\r\n"); 00120 SendCMD(); 00121 timeout=15; 00122 getreply(); 00123 pc.printf(buf); 00124 00125 wait(2); 00126 00127 pc.printf("\n---------- Connecting to AP ----------\r\n"); 00128 pc.printf("ssid = %s pwd = %s\r\n",ssid,pwd); 00129 strcpy(snd, "AT+CWJAP=\""); 00130 strcat(snd, ssid); 00131 strcat(snd, "\",\""); 00132 strcat(snd, pwd); 00133 strcat(snd, "\"\r\n"); 00134 SendCMD(); 00135 timeout=10; 00136 getreply(); 00137 pc.printf(buf); 00138 00139 wait(5); 00140 00141 pc.printf("\n---------- Get IP's ----------\r\n"); 00142 strcpy(snd, "AT+CIFSR\r\n"); 00143 SendCMD(); 00144 timeout=3; 00145 getreply(); 00146 pc.printf(buf); 00147 00148 wait(1); 00149 00150 pc.printf("\n---------- Get Connection Status ----------\r\n"); 00151 strcpy(snd, "AT+CIPSTATUS\r\n"); 00152 SendCMD(); 00153 timeout=5; 00154 getreply(); 00155 pc.printf(buf); 00156 00157 pc.printf("\n\n\n If you get a valid (non zero) IP, ESP8266 has been set up.\r\n"); 00158 pc.printf(" Run this if you want to reconfig the ESP8266 at any time.\r\n"); 00159 pc.printf(" It saves the SSID and password settings internally\r\n"); 00160 wait(10); 00161 } 00162 00163 void SendCMD() 00164 { 00165 esp.printf("%s", snd); 00166 } 00167 00168 void getreply() 00169 { 00170 memset(buf, '\0', sizeof(buf)); 00171 t.start(); 00172 ended=0; 00173 count=0; 00174 while(!ended) { 00175 if(esp.readable()) { 00176 buf[count] = esp.getc(); 00177 count++; 00178 } 00179 if(t.read() > timeout) { 00180 ended = 1; 00181 t.stop(); 00182 t.reset(); 00183 } 00184 } 00185 }
Generated on Sun Jul 17 2022 06:43:38 by
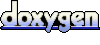