
This example establishes a transparent link between the mbed seial port and the gps on the C027. You can use it to use the standard u-blox tools such as u-center. These tools can then connect to the serial port and talk directly to the GPS receiver. Baudrate should be set to 9600 baud and is fixed. u-center can be downloaded from u-blox website following this link: http://www.u-blox.com/en/evaluation-tools-a-software/u-center/u-center.html
Dependencies: mbed
main.cpp
00001 #include "mbed.h" 00002 #ifdef TARGET_UBLOX_C027 00003 #include "C027_api.h" 00004 #else 00005 #error "This example is targeted for the C027 platform" 00006 #endif 00007 00008 /* This example is establishing a transparent link between 00009 the mbed serial port and the serial communication interface 00010 of the GPS. 00011 00012 For a more advanced driver for the GPS or Modem(MDM) please 00013 look at the follwing library and example: 00014 C027_Support Library 00015 http://mbed.org/teams/ublox/code/C027_Support/ 00016 C027_Support Example 00017 http://mbed.org/teams/ublox/code/C027_SupportTest/ 00018 */ 00019 int main() 00020 { 00021 c027_gps_powerOn(); 00022 int baud = GPSBAUD; 00023 00024 // open the gps serial port 00025 Serial gps(GPSTXD, GPSRXD); 00026 gps.baud(baud); 00027 00028 // open the PC serial port and (use the same baudrate) 00029 Serial pc(USBTX, USBRX); 00030 pc.baud(baud); 00031 00032 while (1) 00033 { 00034 // transfer data from pc to gps 00035 if (pc.readable() && gps.writeable()) 00036 gps.putc(pc.getc()); 00037 // transfer data from gps to pc 00038 if (gps.readable() && pc.writeable()) 00039 pc.putc(gps.getc()); 00040 } 00041 }
Generated on Thu Jul 14 2022 08:45:02 by
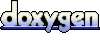