1-Wire® library for mbed. Complete 1-Wire library that supports our silicon masters along with a bit-bang master on the MAX32600MBED platform with one common interface for mbed. Slave support has also been included and more slaves will be added as time permits.
Dependents: MAXREFDES131_Qt_Demo MAX32630FTHR_iButton_uSD_Logger MAX32630FTHR_DS18B20_uSD_Logger MAXREFDES130_131_Demo ... more
OneWireSlave.h
00001 /******************************************************************//** 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 #ifndef OneWire_OneWireSlave 00034 #define OneWire_OneWireSlave 00035 00036 #include <stddef.h> 00037 #include "RomId/RomId.h" 00038 #include "RomId/RomIterator.h" 00039 00040 namespace OneWire 00041 { 00042 class OneWireMaster; 00043 00044 /// Base class for all 1-Wire Slaves. 00045 class OneWireSlave 00046 { 00047 public: 00048 enum CmdResult 00049 { 00050 Success, 00051 CommunicationError, 00052 CrcError, 00053 TimeoutError, 00054 OperationFailure 00055 }; 00056 00057 /// @{ 00058 /// 1-Wire ROM ID for this slave device. 00059 RomId romId () const { return m_romId; } 00060 void setRomId(const RomId & romId ) { m_romId = romId ; } 00061 /// @} 00062 00063 private: 00064 RomId m_romId; 00065 RandomAccessRomIterator & m_selector; 00066 00067 protected: 00068 /// @param selector Provides 1-Wire ROM selection and bus access. 00069 OneWireSlave (RandomAccessRomIterator & selector) : m_selector(selector) { } 00070 00071 ~OneWireSlave() { } 00072 00073 /// Select this slave device by ROM ID. 00074 OneWireMaster::CmdResult selectDevice() const { return m_selector.selectDevice(m_romId); } 00075 00076 /// The 1-Wire master for this slave device. 00077 OneWireMaster & master() const { return m_selector.master(); } 00078 }; 00079 } 00080 00081 #endif
Generated on Tue Jul 12 2022 15:46:21 by
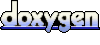