1-Wire® library for mbed. Complete 1-Wire library that supports our silicon masters along with a bit-bang master on the MAX32600MBED platform with one common interface for mbed. Slave support has also been included and more slaves will be added as time permits.
Dependents: MAXREFDES131_Qt_Demo MAX32630FTHR_iButton_uSD_Logger MAX32630FTHR_DS18B20_uSD_Logger MAXREFDES130_131_Demo ... more
DS2431.cpp
00001 /******************************************************************//** 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 **********************************************************************/ 00032 00033 #include "wait_api.h" 00034 #include "Slaves/Memory/DS2431/DS2431.h" 00035 00036 using namespace OneWire; 00037 using namespace OneWire::crc; 00038 00039 enum Command 00040 { 00041 WriteScratchpad = 0x0F, 00042 ReadScratchpad = 0xAA, 00043 CopyScratchpad = 0x55, 00044 ReadMemory = 0xF0 00045 }; 00046 00047 static const DS2431::Address beginReservedAddress = 0x0088; 00048 00049 //********************************************************************* 00050 DS2431::DS2431(RandomAccessRomIterator & selector) : OneWireSlave(selector) 00051 { 00052 } 00053 00054 //********************************************************************* 00055 OneWireSlave::CmdResult DS2431::writeMemory(Address targetAddress, const Scratchpad & data) 00056 { 00057 if (((targetAddress & 0x7) != 0x0) || ((targetAddress + data.size()) > beginReservedAddress)) 00058 { 00059 return OneWireSlave::OperationFailure; 00060 } 00061 OneWireSlave::CmdResult result = writeScratchpad(targetAddress, data); 00062 if (result != OneWireSlave::Success) 00063 { 00064 return result; 00065 } 00066 Scratchpad readData; 00067 uint8_t esByte; 00068 result = readScratchpad(readData, esByte); 00069 if (result != OneWireSlave::Success) 00070 { 00071 return result; 00072 } 00073 result = copyScratchpad(targetAddress, esByte); 00074 return result; 00075 } 00076 00077 //********************************************************************* 00078 OneWireSlave::CmdResult DS2431::readMemory(Address targetAddress, uint8_t numBytes, uint8_t * data) 00079 { 00080 if ((targetAddress + numBytes) > beginReservedAddress) 00081 { 00082 return OneWireSlave::OperationFailure; 00083 } 00084 OneWireMaster::CmdResult owmResult = selectDevice(); 00085 if (owmResult != OneWireMaster::Success) 00086 { 00087 return OneWireSlave::CommunicationError; 00088 } 00089 uint8_t sendBlock[] = { ReadMemory, static_cast<uint8_t>(targetAddress), static_cast<uint8_t>(targetAddress >> 8) }; 00090 owmResult = master().OWWriteBlock(sendBlock, sizeof(sendBlock) / sizeof(sendBlock[0])); 00091 if (owmResult != OneWireMaster::Success) 00092 { 00093 return OneWireSlave::CommunicationError; 00094 } 00095 owmResult = master().OWReadBlock(data, numBytes); 00096 if (owmResult != OneWireMaster::Success) 00097 { 00098 return OneWireSlave::CommunicationError; 00099 } 00100 // else 00101 return OneWireSlave::Success; 00102 } 00103 00104 //********************************************************************* 00105 OneWireSlave::CmdResult DS2431::writeScratchpad(Address targetAddress, const Scratchpad & data) 00106 { 00107 OneWireMaster::CmdResult owmResult = selectDevice(); 00108 if(owmResult != OneWireMaster::Success) 00109 { 00110 return OneWireSlave::CommunicationError; 00111 } 00112 uint8_t sendBlock[3 + Scratchpad::csize] = { WriteScratchpad, static_cast<uint8_t>(targetAddress), static_cast<uint8_t>(targetAddress >> 8) }; 00113 std::memcpy((sendBlock + 3), data.data(), data.size()); 00114 owmResult = master().OWWriteBlock(sendBlock, sizeof(sendBlock) / sizeof(sendBlock[0])); 00115 if (owmResult != OneWireMaster::Success) 00116 { 00117 return OneWireSlave::CommunicationError; 00118 } 00119 uint8_t recvbyte; 00120 owmResult = master().OWReadByte(recvbyte); 00121 if (owmResult != OneWireMaster::Success) 00122 { 00123 return OneWireSlave::CommunicationError; 00124 } 00125 uint16_t invCRC16 = recvbyte; 00126 owmResult = master().OWReadByte(recvbyte); 00127 if (owmResult != OneWireMaster::Success) 00128 { 00129 return OneWireSlave::CommunicationError; 00130 } 00131 invCRC16 |= (recvbyte << 8); 00132 //calc our own inverted CRC16 to compare with one returned 00133 uint16_t calculatedInvCRC16 = ~calculateCrc16(sendBlock, sizeof(sendBlock) / sizeof(sendBlock[0])); 00134 if (invCRC16 != calculatedInvCRC16) 00135 { 00136 return OneWireSlave::CrcError; 00137 } 00138 // else 00139 return OneWireSlave::Success; 00140 } 00141 00142 //********************************************************************* 00143 OneWireSlave::CmdResult DS2431::readScratchpad(Scratchpad & data, uint8_t & esByte) 00144 { 00145 OneWireMaster::CmdResult owmResult = selectDevice(); 00146 if (owmResult != OneWireMaster::Success) 00147 { 00148 return OneWireSlave::CommunicationError; 00149 } 00150 owmResult = master().OWWriteByte(ReadScratchpad); 00151 if (owmResult != OneWireMaster::Success) 00152 { 00153 return OneWireSlave::CommunicationError; 00154 } 00155 uint8_t recvBlock[13]; 00156 const size_t recvBlockSize = sizeof(recvBlock) / sizeof(recvBlock[0]); 00157 owmResult = master().OWReadBlock(recvBlock, recvBlockSize); 00158 if (owmResult != OneWireMaster::Success) 00159 { 00160 return OneWireSlave::CommunicationError; 00161 } 00162 uint16_t invCRC16 = ((recvBlock[recvBlockSize - 1] << 8) | recvBlock[recvBlockSize - 2]); 00163 // Shift contents down 00164 std::memmove(recvBlock + 1, recvBlock, recvBlockSize - 1); 00165 recvBlock[0] = ReadScratchpad; 00166 //calc our own inverted CRC16 to compare with one returned 00167 uint16_t calculatedInvCRC16 = ~calculateCrc16(recvBlock, recvBlockSize - 1); 00168 if (invCRC16 != calculatedInvCRC16) 00169 { 00170 return OneWireSlave::CrcError; 00171 } 00172 esByte = recvBlock[3]; 00173 std::memcpy(data.data(), (recvBlock + 4), data.size()); 00174 return OneWireSlave::Success; 00175 } 00176 00177 //********************************************************************* 00178 OneWireSlave::CmdResult DS2431::copyScratchpad(Address targetAddress, uint8_t esByte) 00179 { 00180 OneWireMaster::CmdResult owmResult = selectDevice(); 00181 if(owmResult != OneWireMaster::Success) 00182 { 00183 return OneWireSlave::CommunicationError; 00184 } 00185 uint8_t sendBlock[] = { CopyScratchpad, static_cast<uint8_t>(targetAddress), static_cast<uint8_t>(targetAddress >> 8) }; 00186 owmResult = master().OWWriteBlock(sendBlock, sizeof(sendBlock) / sizeof(sendBlock[0])); 00187 if (owmResult != OneWireMaster::Success) 00188 { 00189 return OneWireSlave::CommunicationError; 00190 } 00191 owmResult = master().OWWriteByteSetLevel(esByte, OneWireMaster::StrongLevel); 00192 if (owmResult != OneWireMaster::Success) 00193 { 00194 return OneWireSlave::CommunicationError; 00195 } 00196 wait_ms(10); 00197 owmResult = master().OWSetLevel(OneWireMaster::NormalLevel); 00198 if (owmResult != OneWireMaster::Success) 00199 { 00200 return OneWireSlave::CommunicationError; 00201 } 00202 uint8_t check; 00203 owmResult = master().OWReadByte(check); 00204 if (owmResult != OneWireMaster::Success) 00205 { 00206 return OneWireSlave::CommunicationError; 00207 } 00208 if (check != 0xAA) 00209 { 00210 return OneWireSlave::OperationFailure; 00211 } 00212 // else 00213 return OneWireSlave::Success; 00214 }
Generated on Tue Jul 12 2022 15:46:20 by
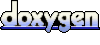