Interface for the encoder of the red fischertechnik motors
FtEncoder Class Reference
Simple Interface for the simple encoder of the red fischertechnik motors. More...
#include <FtEncoder.h>
Public Member Functions | |
FtEncoder (PinName pwm, unsigned int standStillTimeout=50000) | |
create a simple interface to the encoder connected to the given pin | |
unsigned int | getCounter () const |
get number of falling and rising encoder edges | |
void | resetCounter (unsigned int cnt=0) |
reset the encoder edge counter to the given value (default is 0) | |
unsigned int | getLastPeriod () const |
get period [µs] between the two latest edges of same direction (rising or falling) | |
unsigned int | getTimeStampOfLastUpdate () const |
get time stamp [µs] of last update i.e. encoder edge or timeout | |
unsigned int | getCurrentTimeStamp () const |
returns current time stamp [µs] | |
float | getSpeed () const |
get speed in revolutions per second (Hz) | |
void | setCallBack (FunctionPointer callBack) |
hook in a call back to the encoder ISR e.g. for sending a RTOS signal |
Detailed Description
Simple Interface for the simple encoder of the red fischertechnik motors.
The interface counts both, rising and falling edges, resulting in 150 counts per revolution. The interface also provides a speed measurement updated with each edge.
- Note:
- Connect the green wire to GND, the red one to +3.3V and the black signal line to any of mbed numbered pins. Additionally connect the signal line via a pullup resitor to +3.3V. A 10K resistor works fine.
Definition at line 18 of file FtEncoder.h.
Constructor & Destructor Documentation
FtEncoder | ( | PinName | pwm, |
unsigned int | standStillTimeout = 50000 |
||
) |
create a simple interface to the encoder connected to the given pin
- Parameters:
-
name of the pin the black encoder signal wire is connected to standStillTimeout After this time [µs] without any encoder edge getSpeed() returns zero
Definition at line 3 of file FtEncoder.cpp.
Member Function Documentation
unsigned int getCounter | ( | ) | const |
get number of falling and rising encoder edges
Definition at line 46 of file FtEncoder.h.
unsigned int getCurrentTimeStamp | ( | ) | const |
returns current time stamp [µs]
- Note:
- simply calls us_ticker_read() from mbed's C-API (capi/us_ticker_api.h)
Definition at line 66 of file FtEncoder.h.
unsigned int getLastPeriod | ( | ) | const |
get period [µs] between the two latest edges of same direction (rising or falling)
- Note:
- Returns standStillTimeout if motor stands still or no edges have been detected yet
Definition at line 21 of file FtEncoder.cpp.
float getSpeed | ( | ) | const |
get speed in revolutions per second (Hz)
Definition at line 33 of file FtEncoder.cpp.
unsigned int getTimeStampOfLastUpdate | ( | ) | const |
get time stamp [µs] of last update i.e. encoder edge or timeout
Definition at line 60 of file FtEncoder.h.
void resetCounter | ( | unsigned int | cnt = 0 ) |
reset the encoder edge counter to the given value (default is 0)
Definition at line 51 of file FtEncoder.h.
void setCallBack | ( | FunctionPointer | callBack ) |
hook in a call back to the encoder ISR e.g. for sending a RTOS signal
Definition at line 74 of file FtEncoder.h.
Generated on Thu Jul 14 2022 22:48:54 by
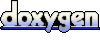