
microServiceBus.com is an integration platform for IoT and enterprise applications. This platform lets you expose microservices from small devices and large systems using a hosting infrastructure. These host can run on both Linux and Windows using components built either natively or using node.js.
Dependencies: C12832 EthernetInterface MbedJSONValue WebSocketClient mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "Websocket.h" 00004 #include "MbedJSONValue.h" 00005 #include "C12832.h" 00006 #include <string> 00007 #include "bootloader.h" 00008 00009 00010 C12832 lcd(D11, D13, D12, D7, D10); 00011 Serial pc1(USBTX, USBRX); // tx, rx 00012 00013 string _organizationId = "74ff8902-2057-499a-bca8-36a699c2458f"; 00014 00015 int main() { 00016 00017 lcd.cls(); 00018 pc1.printf("\r\nStarting up\r\n"); 00019 EthernetInterface eth; 00020 eth.init(); //Use DHCP 00021 eth.connect(); 00022 pc1.printf("IP Address is %s\r\n", eth.getIPAddress()); 00023 00024 //Websocket ws("ws://microservicebus-northeurope-stage.azurewebsites.net/Services/WsHandler.ashx?id=42"); 00025 Websocket ws("ws://192.168.1.64/Services/WsHandler.ashx?id=42"); 00026 bool connected; 00027 connected = ws.connect(); 00028 00029 if(connected) 00030 { 00031 pc1.printf("Connected successfully\r\n"); 00032 } 00033 else 00034 { 00035 pc1.printf("Unable to connect\r\n"); 00036 ws.close(); 00037 exit(0); 00038 } 00039 00040 //while (!ws.connect()); 00041 char str[10000]; 00042 00043 MbedJSONValue signInJson; 00044 signInJson["nodeName"] = eth.getMACAddress(); 00045 signInJson["organizationId"] = _organizationId; 00046 signInJson["machineName"] = eth.getMACAddress(); 00047 signInJson["ip"] = eth.getIPAddress(); 00048 00049 char buf[256]; 00050 snprintf(buf, sizeof buf, "signIn::%s", signInJson.serialize()); 00051 ws.send(buf); 00052 00053 while(1){ 00054 memset(str, 0, 10000); 00055 wait(1.0f); 00056 ///pc1.printf("."); 00057 00058 if (ws.read(str)) { 00059 00060 string msg(str); 00061 00062 if (msg.find("broadcast::") == 0) 00063 { 00064 pc1.printf("Broadcast: "); 00065 pc1.printf(str); 00066 pc1.printf("\r\n"); 00067 } 00068 else if (msg.find("signInMessage::") == 0) 00069 { 00070 pc1.printf("Sign In successfully\r\n"); 00071 } 00072 else if (msg.find("reboot::") == 0) 00073 { 00074 pc1.printf("Disconnecting...\r\n"); 00075 eth.disconnect(); 00076 pc1.printf("\r\nCalling bootloader...\r\n"); 00077 write_flash(); 00078 } 00079 else if (msg.find("errorMessage::") == 0) 00080 { 00081 pc1.printf("ERROR...\r\n"); 00082 pc1.printf(str); 00083 pc1.printf("\r\n"); 00084 } 00085 else if (msg.find("ping::") == 0) 00086 { 00087 msg.replace(0,6,""); 00088 pc1.printf("ping from:"); 00089 pc1.printf(msg.c_str()); 00090 pc1.printf("\r\n"); 00091 00092 MbedJSONValue pingRequestJson; 00093 const char * json = msg.c_str(); 00094 parse(pingRequestJson, json); 00095 std::string connectionid; 00096 connectionid = pingRequestJson["connectionid"].get<std::string>(); 00097 00098 MbedJSONValue pingResponseJson; 00099 pingResponseJson["nodeName"] = eth.getMACAddress(); 00100 pingResponseJson["organizationId"] = _organizationId; 00101 pingResponseJson["connectionid"] = connectionid; 00102 pingResponseJson["status"] = "online"; 00103 00104 snprintf(buf, sizeof buf, "pingResponse::%s", pingResponseJson.serialize()); 00105 ws.send(buf); 00106 } 00107 } 00108 00109 } 00110 ws.close(); 00111 00112 // lcd.printf("Signing out..."); 00113 } 00114 char* appendCharToCharArray(char* array, char a) 00115 { 00116 size_t len = strlen(array); 00117 00118 char* ret = new char[len+2]; 00119 00120 strcpy(ret, array); 00121 ret[len] = a; 00122 ret[len+1] = '\0'; 00123 00124 return ret; 00125 }
Generated on Fri Jul 15 2022 01:35:33 by
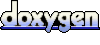