Bluetooth JPEG camera library with test program build over the the original library for LS-Y201 LinkSprite JPEG Camera
Dependents: JPEGCameraTest JPEGCamera_SIM808_STM32F401RE JPEGCamera_SIM808_MPU9150_STM32F401RE
JPEGCamera.h
00001 /* Arduino JPEGCamera Library 00002 * Copyright 2010 SparkFun Electronics 00003 * Written by Ryan Owens 00004 * Modified by arms22 00005 * Ported to mbed by yamaguch 00006 * Modified by thesane 00007 */ 00008 00009 #ifndef JPEG_CAMERA_H 00010 #define JPEG_CAMERA_H 00011 00012 #include "mbed.h" 00013 00014 /** 00015 * Interface for LinkSprite JPEG Camera module LS-Y201 00016 */ 00017 class JPEGCamera : public Serial { 00018 public: 00019 /***/ 00020 enum PictureSize { 00021 SIZE160x120 = 0x22, 00022 SIZE320x240 = 0x11, 00023 SIZE640x480 = 0x00, 00024 }; 00025 00026 /** 00027 * Create JPEG Camera 00028 * 00029 * @param tx tx pin 00030 * @param rx rx pin 00031 */ 00032 JPEGCamera(PinName tx, PinName rx); 00033 00034 /** 00035 * Set picture size 00036 * 00037 * @param size picture size (available sizes are SIZE160x120, SIZE320x240, SIZE640x480) 00038 * @param doReset flag to perform reset operation after changing size 00039 * 00040 * @returns true if succeeded, false otherwise 00041 */ 00042 bool setPictureSize(JPEGCamera::PictureSize size, bool doReset = true); 00043 00044 /** 00045 * Return whether camera is ready or not 00046 * 00047 * @returns true if ready, false otherwise 00048 */ 00049 bool isReady(); 00050 00051 /** 00052 * Return whether camera is processing the taken picture or not 00053 * 00054 * @returns true if the camera is in processing, false otherwise 00055 */ 00056 bool isProcessing(); 00057 00058 /** 00059 * Take a picture 00060 * 00061 * @param filename filename to store the picture data 00062 * @returns true if succeeded, false otherwise 00063 */ 00064 bool takePicture(); 00065 /** 00066 * Process picture (writing the file) 00067 * 00068 * @param address of serial port used to transmit the image 00069 * @returns true if no error in processing, false otherwise 00070 */ 00071 bool processPicture(Serial &control); 00072 00073 /** 00074 * Perform reset oepration (it takes 4 seconds) 00075 * 00076 * @returns true if succeeded, false otherwise 00077 */ 00078 bool reset(); 00079 00080 /** 00081 * Send a picture command to the camera module 00082 * 00083 * @returns true if succeeded, false otherwise 00084 */ 00085 bool takePicture_int(void); 00086 00087 /** 00088 * Send a stop pictures command to the camera module 00089 * 00090 * @returns true if succeeded, false otherwise 00091 */ 00092 bool stopPictures(void); 00093 00094 /** 00095 * Get the picture image size 00096 * 00097 * @returns the actual image size in bytes 00098 */ 00099 int getImageSize(); 00100 00101 /** 00102 * Read the picture data to the buffer 00103 * 00104 * @param dataBuf data buffer address to store the received data 00105 * @param size data size to read 00106 * @param address the address of the picture data to read 00107 * 00108 * @returns the size of the data read 00109 */ 00110 int readData(char *dataBuf, int size, int address); 00111 00112 //private: 00113 Timer timer; 00114 FILE *fp; 00115 int imageSize; 00116 int address; 00117 enum State {UNKNOWN, READY, PROCESSING, ERROR = -1} state; 00118 00119 int sendReceive(char *buf, int sendSize, int receiveSize); 00120 int receive(char *buf, int size, int timeout); 00121 }; 00122 00123 #endif
Generated on Mon Jul 18 2022 19:13:10 by
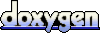