Bluetooth JPEG camera library with test program build over the the original library for LS-Y201 LinkSprite JPEG Camera
Dependents: JPEGCameraTest JPEGCamera_SIM808_STM32F401RE JPEGCamera_SIM808_MPU9150_STM32F401RE
JPEGCamera.cpp
00001 /* Arduino JPEGCamera Library 00002 * Copyright 2010 SparkFun Electronic 00003 * Written by Ryan Owens 00004 * Modified by arms22 00005 * Ported to mbed by yamaguch 00006 * Modified by thesane 00007 */ 00008 00009 #include "JPEGCamera.h" 00010 00011 #define min(x, y) ((x) < (y)) ? (x) : (y) 00012 00013 const int RESPONSE_TIMEOUT = 500; 00014 const int DATA_TIMEOUT = 1000; 00015 00016 JPEGCamera::JPEGCamera(PinName tx, PinName rx) : Serial(tx, rx) { 00017 baud(38400); 00018 state = READY; 00019 } 00020 00021 bool JPEGCamera::setPictureSize(JPEGCamera::PictureSize size, bool doReset) { 00022 char buf[9] = {0x56, 0x00, 0x31, 0x05, 0x04, 0x01, 0x00, 0x19, (char) size}; 00023 int ret = sendReceive(buf, sizeof buf, 5); 00024 00025 if (ret == 5 && buf[0] == 0x76) { 00026 if (doReset) 00027 reset(); 00028 return true; 00029 } else 00030 return false; 00031 } 00032 00033 bool JPEGCamera::isReady() { 00034 return state == READY; 00035 } 00036 00037 bool JPEGCamera::isProcessing() { 00038 return state == PROCESSING; 00039 } 00040 00041 bool JPEGCamera::takePicture() { 00042 if (state == READY) { 00043 if (takePicture_int()) { 00044 imageSize = getImageSize(); 00045 address = 0; 00046 state = PROCESSING; 00047 } else { 00048 state = ERROR; 00049 } 00050 00051 } 00052 return state != ERROR; 00053 } 00054 00055 bool JPEGCamera::processPicture(Serial &control) { 00056 if (state == PROCESSING) { 00057 if (address < imageSize) { 00058 char data[1024]; 00059 int size = readData(data, min(sizeof(data), imageSize - address), address); 00060 for(int i = 0;i<size;i++) 00061 control.putc(data[i]); 00062 address += size; 00063 if (address >= imageSize) { 00064 stopPictures(); 00065 wait(0.1); 00066 state = READY; 00067 } 00068 } 00069 } 00070 00071 return state == PROCESSING || state == READY; 00072 } 00073 00074 bool JPEGCamera::reset() { 00075 char buf[4] = {0x56, 0x00, 0x26, 0x00}; 00076 int ret = sendReceive(buf, sizeof buf, 4); 00077 if (ret == 4 && buf[0] == 0x76) { 00078 wait(4.0); 00079 state = READY; 00080 } else { 00081 state = ERROR; 00082 } 00083 return state == READY; 00084 } 00085 00086 bool JPEGCamera::takePicture_int() { 00087 char buf[5] = {0x56, 0x00, 0x36, 0x01, 0x00}; 00088 int ret = sendReceive(buf, sizeof buf, 5); 00089 00090 return ret == 5 && buf[0] == 0x76; 00091 } 00092 00093 bool JPEGCamera::stopPictures() { 00094 char buf[5] = {0x56, 0x00, 0x36, 0x01, 0x03}; 00095 int ret = sendReceive(buf, sizeof buf, 5); 00096 00097 return ret == 4 && buf[0] == 0x76; 00098 } 00099 00100 int JPEGCamera::getImageSize() { 00101 char buf[9] = {0x56, 0x00, 0x34, 0x01, 0x00}; 00102 int ret = sendReceive(buf, sizeof buf, 9); 00103 00104 //The size is in the last 2 characters of the response. 00105 return (ret == 9 && buf[0] == 0x76) ? (buf[7] << 8 | buf[8]) : 0; 00106 } 00107 00108 int JPEGCamera::readData(char *dataBuf, int size, int address) { 00109 char buf[16] = {0x56, 0x00, 0x32, 0x0C, 0x00, 0x0A, 0x00, 0x00, 00110 address >> 8, address & 255, 0x00, 0x00, size >> 8, size & 255, 0x00, 0x0A 00111 }; 00112 int ret = sendReceive(buf, sizeof buf, 5); 00113 00114 return (ret == 5 && buf[0] == 0x76) ? receive(dataBuf, size, DATA_TIMEOUT) : 0; 00115 } 00116 00117 int JPEGCamera::sendReceive(char *buf, int sendSize, int receiveSize) { 00118 while (readable()) getc(); 00119 00120 for (int i = 0; i < sendSize; i++) putc(buf[i]); 00121 00122 return receive(buf, receiveSize, RESPONSE_TIMEOUT); 00123 } 00124 00125 int JPEGCamera::receive(char *buf, int size, int timeout) { 00126 timer.start(); 00127 timer.reset(); 00128 00129 int i = 0; 00130 while (i < size && timer.read_ms() < timeout) { 00131 if (readable()) 00132 buf[i++] = getc(); 00133 } 00134 00135 return i; 00136 }
Generated on Mon Jul 18 2022 19:13:10 by
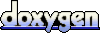