EthernetInterface Libraryを使ったSimpleな SMTP ClientLibraryです. LOGIN認証を追加しました.(2014.4 Update) It is SMTPClient Library which is Simple using EthernetInterface Library.
Dependents: SimpleSMTPClient_HelloWorld USBHost-MSD_Sensors_1 IOT-GPS-SMS IOT_HW_5_websockets ... more
SimpleSMTPClient.h
00001 /* 00002 * 00003 * mbed Simple SMTP Client 00004 * Copyright (c) 2012 Tadao Iida 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 */ 00007 00008 /** @file 00009 * @brief Simple SMTP Client 00010 */ 00011 00012 #ifndef SimpleSMTPC_h 00013 #define SimpleSMTPC_h 00014 00015 #include "EthernetInterface.h" 00016 00017 ///SMTP authentication 00018 enum SMTPAuth { 00019 SMTP_AUTH_NONE, ///<No authentication 00020 SMTP_AUTH_PLAIN, ///<AUTH PLAIN authentication 00021 SMTP_AUTH_LOGIN 00022 }; 00023 00024 ///SimpleSMTP client results 00025 enum SMTPResult 00026 { 00027 SMTP_AUTH_OK = 235, ///<Authentication successful 00028 SMTP_OK = 250, ///<Requested mail action okay, completed 00029 SMTP_INPUT = 354 ///<Start mail input 00030 }; 00031 #define DEBUG 00032 00033 #define SMTP_TIMEOUT 15000 // ms 00034 00035 class SimpleSMTPClient 00036 { 00037 public: 00038 /** 00039 Instantiate the SimpleSMTP client 00040 */ 00041 SimpleSMTPClient(); 00042 00043 /** send mail 00044 * @param host mail server 00045 * @param data mail body 00046 * @param user auth username 00047 * @param pwd auth password 00048 * @param domain 00049 * @param port mail port 00050 * @param auth SMTP auth 00051 * @return 0:success, -1:failue 00052 */ 00053 int sendmail (char *host, char *user, char *pwd,char *domain,char *port,SMTPAuth auth); 00054 00055 /** setMessage 00056 * @param sub Subject <The Subject are less than 64 characters.> 00057 * @param msg Message 00058 * @return 0:success, -1:failue 00059 */ 00060 int setMessage(char *sub,char *msg); 00061 00062 /** addMessage 00063 * @param msg Message 00064 * @return 0:success, -1:failue 00065 */ 00066 int addMessage(char *msg); 00067 00068 /** setFromAddress 00069 * @param from mail address 00070 * @return character count, -1:failue 00071 */ 00072 int setFromAddress(char *from); 00073 00074 /** setToAddress <The ToAddress are less than 128 characters.> 00075 * @param to mail address 00076 * @return character count, -1:failue 00077 */ 00078 int setToAddress(char *to); 00079 00080 /** clearMessage 00081 * 00082 */ 00083 void clearMessage(void); 00084 00085 /** msgLength 00086 * 00087 */ 00088 int msgLength(void); 00089 00090 00091 private: 00092 int base64enc(const char *input, unsigned int length, char *output, int outputlen); 00093 int receiveMessage(int code); 00094 char* getFromAddress(void); 00095 char* getToAddress(void); 00096 char* getSubject(); 00097 char* getHeader(); 00098 char* getMessage(); 00099 int makeHeader(void); 00100 00101 TCPSocketConnection smtp; 00102 char header[256]; 00103 char body[1500]; 00104 char from[64]; 00105 char to[128]; 00106 char subject[64]; 00107 char message[1244]; 00108 }; 00109 00110 #endif
Generated on Sat Jul 16 2022 18:21:37 by
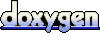