mbed library sources. Supersedes mbed-src.
Fork of mbed-dev by
Embed:
(wiki syntax)
Show/hide line numbers
i2c_api.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_I2C_API_H 00017 #define MBED_I2C_API_H 00018 00019 #include "device.h" 00020 #include "buffer.h" 00021 #include "dma_api.h" 00022 00023 #if DEVICE_I2C 00024 00025 /** 00026 * @defgroup I2CEvents I2C Events Macros 00027 * 00028 * @{ 00029 */ 00030 #define I2C_EVENT_ERROR (1 << 1) 00031 #define I2C_EVENT_ERROR_NO_SLAVE (1 << 2) 00032 #define I2C_EVENT_TRANSFER_COMPLETE (1 << 3) 00033 #define I2C_EVENT_TRANSFER_EARLY_NACK (1 << 4) 00034 #define I2C_EVENT_ALL (I2C_EVENT_ERROR | I2C_EVENT_TRANSFER_COMPLETE | I2C_EVENT_ERROR_NO_SLAVE | I2C_EVENT_TRANSFER_EARLY_NACK) 00035 00036 /**@}*/ 00037 00038 #if DEVICE_I2C_ASYNCH 00039 /** Asynch i2c hal structure 00040 */ 00041 typedef struct { 00042 struct i2c_s i2c; /**< Target specific i2c structure */ 00043 struct buffer_s tx_buff; /**< Tx buffer */ 00044 struct buffer_s rx_buff; /**< Rx buffer */ 00045 } i2c_t; 00046 00047 #else 00048 /** Non-asynch i2c hal structure 00049 */ 00050 typedef struct i2c_s i2c_t; 00051 00052 #endif 00053 00054 enum { 00055 I2C_ERROR_NO_SLAVE = -1, 00056 I2C_ERROR_BUS_BUSY = -2 00057 }; 00058 00059 #ifdef __cplusplus 00060 extern "C" { 00061 #endif 00062 00063 /** 00064 * \defgroup GeneralI2C I2C Configuration Functions 00065 * @{ 00066 */ 00067 00068 /** Initialize the I2C peripheral. It sets the default parameters for I2C 00069 * peripheral, and configure its specifieds pins. 00070 * @param obj The i2c object 00071 * @param sda The sda pin 00072 * @param scl The scl pin 00073 */ 00074 void i2c_init(i2c_t *obj, PinName sda, PinName scl); 00075 00076 /** Configure the I2C frequency. 00077 * @param obj The i2c object 00078 * @param hz Frequency in Hz 00079 */ 00080 void i2c_frequency(i2c_t *obj, int hz); 00081 00082 /** Send START command. 00083 * @param obj The i2c object 00084 */ 00085 int i2c_start(i2c_t *obj); 00086 00087 /** Send STOP command. 00088 * @param obj The i2c object 00089 */ 00090 int i2c_stop(i2c_t *obj); 00091 00092 /** Blocking reading data. 00093 * @param obj The i2c object 00094 * @param address 7-bit address (last bit is 1) 00095 * @param data The buffer for receiving 00096 * @param length Number of bytes to read 00097 * @param stop Stop to be generated after the transfer is done 00098 * @return Number of read bytes 00099 */ 00100 int i2c_read(i2c_t *obj, int address, char *data, int length, int stop); 00101 00102 /** Blocking sending data. 00103 * @param obj The i2c object 00104 * @param address 7-bit address (last bit is 0) 00105 * @param data The buffer for sending 00106 * @param length Number of bytes to wrte 00107 * @param stop Stop to be generated after the transfer is done 00108 * @return Number of written bytes 00109 */ 00110 int i2c_write(i2c_t *obj, int address, const char *data, int length, int stop); 00111 00112 /** Reset I2C peripheral. TODO: The action here. Most of the implementation sends stop(). 00113 * @param obj The i2c object 00114 */ 00115 void i2c_reset(i2c_t *obj); 00116 00117 /** Read one byte. 00118 * @param obj The i2c object 00119 * @param last Acknoledge 00120 * @return The read byte 00121 */ 00122 int i2c_byte_read(i2c_t *obj, int last); 00123 00124 /** Write one byte. 00125 * @param obj The i2c object 00126 * @param data Byte to be written 00127 * @return 0 if NAK was received, 1 if ACK was received, 2 for timeout. 00128 */ 00129 int i2c_byte_write(i2c_t *obj, int data); 00130 00131 /**@}*/ 00132 00133 #if DEVICE_I2CSLAVE 00134 00135 /** 00136 * \defgroup SynchI2C Synchronous I2C Hardware Abstraction Layer for slave 00137 * @{ 00138 */ 00139 00140 /** Configure I2C as slave or master. 00141 * @param obj The I2C object 00142 * @return non-zero if a value is available 00143 */ 00144 void i2c_slave_mode(i2c_t *obj, int enable_slave); 00145 00146 /** Check to see if the I2C slave has been addressed. 00147 * @param obj The I2C object 00148 * @return The status - 1 - read addresses, 2 - write to all slaves, 00149 * 3 write addressed, 0 - the slave has not been addressed 00150 */ 00151 int i2c_slave_receive(i2c_t *obj); 00152 00153 /** Configure I2C as slave or master. 00154 * @param obj The I2C object 00155 * @return non-zero if a value is available 00156 */ 00157 int i2c_slave_read(i2c_t *obj, char *data, int length); 00158 00159 /** Configure I2C as slave or master. 00160 * @param obj The I2C object 00161 * @return non-zero if a value is available 00162 */ 00163 int i2c_slave_write(i2c_t *obj, const char *data, int length); 00164 00165 /** Configure I2C address. 00166 * @param obj The I2C object 00167 * @param idx Currently not used 00168 * @param address The address to be set 00169 * @param mask Currently not used 00170 */ 00171 void i2c_slave_address(i2c_t *obj, int idx, uint32_t address, uint32_t mask); 00172 00173 #endif 00174 00175 /**@}*/ 00176 00177 #if DEVICE_I2C_ASYNCH 00178 00179 /** 00180 * \defgroup AsynchI2C Asynchronous I2C Hardware Abstraction Layer 00181 * @{ 00182 */ 00183 00184 /** Start i2c asynchronous transfer. 00185 * @param obj The I2C object 00186 * @param tx The buffer to send 00187 * @param tx_length The number of words to transmit 00188 * @param rx The buffer to receive 00189 * @param rx_length The number of words to receive 00190 * @param address The address to be set - 7bit or 9 bit 00191 * @param stop If true, stop will be generated after the transfer is done 00192 * @param handler The I2C IRQ handler to be set 00193 * @param hint DMA hint usage 00194 */ 00195 void i2c_transfer_asynch(i2c_t *obj, const void *tx, size_t tx_length, void *rx, size_t rx_length, uint32_t address, uint32_t stop, uint32_t handler, uint32_t event, DMAUsage hint); 00196 00197 /** The asynchronous IRQ handler 00198 * @param obj The I2C object which holds the transfer information 00199 * @return event flags if a transfer termination condition was met or 0 otherwise. 00200 */ 00201 uint32_t i2c_irq_handler_asynch(i2c_t *obj); 00202 00203 /** Attempts to determine if I2C peripheral is already in use. 00204 * @param obj The I2C object 00205 * @return non-zero if the I2C module is active or zero if it is not 00206 */ 00207 uint8_t i2c_active(i2c_t *obj); 00208 00209 /** Abort ongoing asynchronous transaction. 00210 * @param obj The I2C object 00211 */ 00212 void i2c_abort_asynch(i2c_t *obj); 00213 00214 #endif 00215 00216 /**@}*/ 00217 00218 #ifdef __cplusplus 00219 } 00220 #endif 00221 00222 #endif 00223 00224 #endif
Generated on Tue Jul 12 2022 20:02:09 by
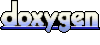