
Websocket Hello World over an ethernet network
Dependencies: EthernetNetIf mbed DNSResolver
main.cpp
00001 #include "mbed.h" 00002 #include "Websocket.h" 00003 00004 Serial pc(USBTX, USBRX); 00005 Timer tmr; 00006 00007 //Here, we create a Websocket instance in 'rw' (write) mode 00008 //on the 'samux' channel 00009 Websocket ws("ws://sockets.mbed.org/ws/samux/rw"); 00010 00011 int main() { 00012 char recv[128]; 00013 while (1) { 00014 00015 while (!ws.connect()) 00016 pc.printf("cannot connect websocket, retrying\r\n"); 00017 00018 tmr.start(); 00019 while (1) { 00020 if (tmr.read() > 0.5) { 00021 ws.send("Hello World! over Ethernet"); 00022 if (ws.read(recv)) { 00023 pc.printf("recv: %s\r\n", recv); 00024 } 00025 tmr.start(); 00026 } 00027 Net::poll(); 00028 } 00029 } 00030 }
Generated on Mon Aug 1 2022 07:57:28 by
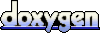