
An application to log WiFi SSIDs for position lookup testing
Dependencies: C027_Support SWO mbed-rtos mbed picojson
Fork of lpc4088_ebb_ublox_Cellular_PubNubDemo_rtos by
DeviceConfiguration.cpp
00001 #include "DeviceConfiguration.h" 00002 #include <stdlib.h> 00003 #include <stdio.h> 00004 #include <string.h> 00005 00006 DeviceConfiguration::DeviceConfiguration() 00007 { 00008 size_t i; 00009 00010 for (i = 0; i < DEVICE_CONFIGURATION_SIZE; i++) { 00011 _items[i].key = NULL; 00012 _items[i].value = NULL; 00013 } 00014 } 00015 00016 DeviceConfiguration::~DeviceConfiguration() 00017 { 00018 clear(); 00019 } 00020 00021 bool DeviceConfiguration::read(const char *str) 00022 { 00023 const char *ptr, *ptr2, *ptr3; size_t i, j, len1, len2; 00024 DeviceConfiguration::KeyValue items[DEVICE_CONFIGURATION_SIZE]; 00025 00026 for (i = 0; i < DEVICE_CONFIGURATION_SIZE; i++) { 00027 items[i].key = NULL; 00028 items[i].value = NULL; 00029 } 00030 00031 ptr = str; 00032 i = 0; 00033 while ((*ptr != '\0') && (i < DEVICE_CONFIGURATION_SIZE)) { 00034 if (((ptr2 = strchr(ptr, '=')) == NULL) || 00035 ((ptr3 = strchr(ptr2+1, ';')) == NULL)) 00036 goto failure; 00037 00038 len1 = ptr2-ptr; 00039 len2 = ptr3-ptr2 - 1; 00040 00041 if ((memchr(ptr, ';', len1) != NULL) || 00042 (memchr(ptr2+1, '=', len2) != NULL)) 00043 goto failure; 00044 00045 for (j = 0; j < DEVICE_CONFIGURATION_SIZE; j++) { 00046 if ((items[j].key != NULL) && (strlen(items[j].key) == len1) && (strncmp(items[j].key, ptr, len1) == 0)) 00047 goto failure; 00048 } 00049 00050 if ((items[i].key = (char*)malloc(len1+1)) == NULL) 00051 goto failure; 00052 if ((items[i].value = (char*)malloc(len2+1)) == NULL) { 00053 free(items[i].key); 00054 items[i].key = NULL; 00055 goto failure; 00056 } 00057 00058 strncpy(items[i].key, ptr, len1); 00059 strncpy(items[i].value, ptr2+1, len2); 00060 items[i].key[len1] = '\0'; 00061 items[i].value[len2] = '\0'; 00062 00063 i++; 00064 ptr = ptr3+1; 00065 } 00066 00067 if (*ptr != '\0') 00068 goto failure; 00069 00070 clear(); 00071 memcpy(_items, items, sizeof(DeviceConfiguration::KeyValue)*DEVICE_CONFIGURATION_SIZE); 00072 return true; 00073 00074 failure: 00075 for (i = 0; i < DEVICE_CONFIGURATION_SIZE; i++) { 00076 if (items[i].key != NULL) { 00077 free(items[i].key); 00078 free(items[i].value); 00079 } 00080 } 00081 00082 return false; 00083 } 00084 00085 bool DeviceConfiguration::write(char *buf, size_t len) 00086 { 00087 char *ptr; size_t i; int ret, ln; 00088 00089 ptr = buf; 00090 for (i = 0; i < DEVICE_CONFIGURATION_SIZE; i++) { 00091 if (_items[i].key == NULL) 00092 continue; 00093 00094 ret = snprintf(ptr, len, "%s=%s;%n", _items[i].key, _items[i].value, &ln); 00095 if ((ret < 0) || (ret >= len)) 00096 return false; 00097 00098 ptr += ln; 00099 len -= ln; 00100 } 00101 00102 return true; 00103 } 00104 00105 bool DeviceConfiguration::set(const char *key, const char *value) 00106 { 00107 KeyValue *item; size_t i; 00108 00109 if ((item = search(key)) == NULL) { 00110 for (i = 0; (i < DEVICE_CONFIGURATION_SIZE) && (item == NULL); i++) { 00111 if (_items[i].key == NULL) 00112 item = &_items[i]; 00113 } 00114 } 00115 00116 if (item == NULL) 00117 return false; 00118 00119 if ((item->key = (char*)malloc(strlen(key)+1)) == NULL) 00120 return false; 00121 if ((item->value = (char*)malloc(strlen(value)+1)) == NULL) { 00122 free(item->key); 00123 item->key = NULL; 00124 return false; 00125 } 00126 00127 strcpy(item->key, key); 00128 strcpy(item->value, value); 00129 return true; 00130 } 00131 00132 const char * DeviceConfiguration::get(const char *key) 00133 { 00134 KeyValue *item; 00135 00136 if ((item = search(key)) == NULL) 00137 return NULL; 00138 00139 return item->value; 00140 } 00141 00142 bool DeviceConfiguration::unset(const char *key) 00143 { 00144 KeyValue *item; 00145 00146 if ((item = search(key)) == NULL) 00147 return false; 00148 00149 free(item->key); 00150 free(item->value); 00151 item->key = NULL; 00152 item->value = NULL; 00153 return true; 00154 } 00155 00156 bool DeviceConfiguration::has(const char *key) 00157 { 00158 return (search(key) != NULL); 00159 } 00160 00161 void DeviceConfiguration::clear() 00162 { 00163 size_t i; 00164 00165 for (i = 0; i < DEVICE_CONFIGURATION_SIZE; i++) { 00166 if (_items[i].key != NULL) { 00167 free(_items[i].key); 00168 free(_items[i].value); 00169 } 00170 } 00171 } 00172 00173 DeviceConfiguration::KeyValue * DeviceConfiguration::search(const char *key) 00174 { 00175 size_t i; 00176 00177 for (i = 0; i < DEVICE_CONFIGURATION_SIZE; i++) { 00178 if (strcmp(key, _items[i].key) == 0) 00179 return &_items[i]; 00180 } 00181 00182 return NULL; 00183 }
Generated on Sun Jul 17 2022 02:39:36 by
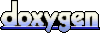