Library for H-Bridge Motor Driver Using Bipolar Transistors
Embed:
(wiki syntax)
Show/hide line numbers
motorlib.h
00001 /*mbed motorlib Library for H-Bridge Motor Driver Using Bipolar Transistors 00002 * 00003 * mbed simple H-bridge motor controller 00004 * Copyright (c) 2007-2012, Prabhu Desai 00005 * 00006 00007 */ 00008 00009 #ifndef MBED_MOTOR_H 00010 #define MBED_MOTOR_H 00011 00012 #include "mbed.h" 00013 00014 #define LM293D_MOTOR_CTRL 00015 /** Interface to control a standard DC motor 00016 * 00017 */ 00018 class Motor { 00019 public: 00020 /** Motor Control Type */ 00021 enum MCtrlType { 00022 L293D_DISCRETE /**< 0 or 100% duty cycle with L293D */ 00023 , L293D_PWM /**< PWM based voltage control with L293D */ 00024 , COMPS_DISCRETE /**< 0 or 100% duty cycle with transistor circuit*/ 00025 , COMPS_PWM /**< PWM based voltage control with transistor circuit*/ 00026 00027 }; 00028 00029 /** Create a motor control interface 00030 * 00031 * @param r1 DigitalOut, driving H-bridge NPN transistor on the BOTTOm right 00032 * @param r2 DigitalOut, driving H-bridge PNP transistor on the TOP right 00033 * @param r3 DigitalOut, driving H-bridge NPN transistor on the BOTTOm left. 00034 * @param r4 DigitalOut, driving H-bridge PNP transistor on the TOP left 00035 * @param mctype DigitalOut, driving H-bridge PNP transistor on the TOP left 00036 */ 00037 Motor(PinName r1, PinName r4,MCtrlType mctype =L293D_PWM); 00038 Motor(PinName r1, PinName r2, PinName r3, PinName r4,MCtrlType mctype =L293D_PWM); 00039 00040 /** Set the motor to coast/roll/off 00041 * 00042 * @param void 00043 * @return void 00044 */ 00045 void coast(void); 00046 00047 /** Rotate motor in clockwise direction. 00048 * 00049 * @param void 00050 * @return void 00051 */ 00052 void forward(void); 00053 00054 /** /** Rotate motor in Anti-clockwise direction. 00055 * 00056 * @param void 00057 * @return void 00058 */ 00059 00060 void backward(void); 00061 00062 /** Stop the motor. 00063 * 00064 * @param void 00065 * @return void 00066 */ 00067 00068 void stop(void); 00069 00070 protected: 00071 #ifdef LM293D_MOTOR_CTRL 00072 PwmOut _r1; 00073 PwmOut _r4; 00074 #else 00075 DigitalOut _r1; 00076 DigitalOut _r2; 00077 DigitalOut _r3; 00078 DigitalOut _r4; 00079 #endif 00080 MCtrlType _mctype; 00081 00082 }; 00083 00084 #endif
Generated on Tue Jul 12 2022 20:46:04 by
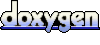