
A test program for the TLV320, Generates some sine waves.
Dependencies: I2S TLV320 mbed sinelookup
main.cpp
00001 #include "mbed.h" 00002 #include "sinelookup.h" 00003 #include "TLV320.h" 00004 #include "I2S.h" 00005 00006 #define SAMPLERATE 48000 00007 00008 00009 TLV320 codec(p9, p10); 00010 I2S i2s(I2S_TRANSMIT, p5, p6, p7); 00011 00012 AnalogIn aIn(p19); 00013 AnalogIn vol(p20); 00014 00015 float phase_l; 00016 float phase_r; 00017 int minibuf[16]; 00018 float skipper; 00019 float volume; 00020 float phase_kill; 00021 00022 00023 extern "C" void HardFault_Handler() 00024 { 00025 error("Hard Fault!\n"); 00026 } 00027 00028 00029 void play(void) 00030 { 00031 int to_write = i2s.max_fifo_points() - (i2s.fifo_points()); 00032 for(int i = 0; i < to_write; i+=2) { 00033 minibuf[i] = int(float(sine16lookup[int(phase_l)])*volume); 00034 //minibuf[i] = int(sine16lookup[int(phase_l)]); 00035 minibuf[i+1] = int(float(sine16lookup[int(phase_r)])*volume); 00036 phase_l+=skipper; 00037 phase_r = phase_l + phase_kill; 00038 while(phase_l >= SINE16LENGTH) { 00039 phase_l -= SINE16LENGTH; 00040 } 00041 00042 while(phase_r >= SINE16LENGTH) { 00043 phase_r -= SINE16LENGTH; 00044 } 00045 while(phase_r< 0) phase_r += SINE16LENGTH; 00046 } 00047 i2s.write(minibuf, to_write); 00048 } 00049 00050 /* main */ 00051 int main() 00052 { 00053 skipper = (aIn*49)+1; 00054 00055 codec.power(true); 00056 codec.frequency(SAMPLERATE); 00057 codec.wordsize(16); 00058 codec.master(false); 00059 codec.headphone_volume(0.5); 00060 codec.start(); 00061 00062 i2s.frequency(SAMPLERATE); 00063 i2s.wordsize(16); 00064 i2s.stereomono(I2S_STEREO); 00065 i2s.masterslave(I2S_MASTER); 00066 i2s.attach(&play); 00067 i2s.start(); 00068 00069 while(1) 00070 { 00071 skipper = (aIn*30)+1; 00072 00073 volume = 1; 00074 phase_kill = (vol-0.5)*400; 00075 00076 //printf("Skipper:%f, %i, %i\n\r",skipper, to_write,i2s.fifo_level()); 00077 00078 /*if(flag) 00079 { 00080 printf("."); 00081 flag = false; 00082 }*/ 00083 } 00084 00085 }
Generated on Fri Jul 15 2022 12:05:36 by
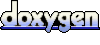