The modified AndroidAccessory Library
Dependents: ADKTerm droidcycle uva_nc androidservo ... more
AndroidAccessory.h
00001 /* mbed AndroidAccessory Library 00002 * Created by p07gbar from work by Makoto Abe 00003 * 00004 */ 00005 #ifndef ADK_H_INCLUDED 00006 #define ADK_H_INCLUDED 00007 00008 #include "mbed.h" 00009 #include "USBHost.h" 00010 00011 00012 //#define ADKLOG 1 00013 #if ADKLOG 00014 #define LOG(...) printf(__VA_ARGS__) 00015 #define Log(...) printf(__VA_ARGS__) 00016 #define log(...) printf(__VA_ARGS__) 00017 00018 #else 00019 #define LOG(...) do {} while(0) 00020 #define Log(...) do {} while(0) 00021 #define log(...) do {} while(0) 00022 00023 #endif 00024 00025 #define ACCESSORY_STRING_MANUFACTURER 0 00026 #define ACCESSORY_STRING_MODEL 1 00027 #define ACCESSORY_STRING_DESCRIPTION 2 00028 #define ACCESSORY_STRING_VERSION 3 00029 #define ACCESSORY_STRING_URI 4 00030 #define ACCESSORY_STRING_SERIAL 5 00031 00032 #define ACCESSORY_GET_PROTOCOL 51 00033 #define ACCESSORY_SEND_STRING 52 00034 #define ACCESSORY_START 53 00035 00036 00037 00038 /** An AndroidAccessory control class 00039 * 00040 * It allows easy creation of a mbed android ADK accessory, with minimal low level fussing. 00041 * Base code should have methods resetDevice(), setupDevice(), callbackRead(u8 *buff, int len) and callBackWrite() functions 00042 * 00043 */ 00044 00045 class AndroidAccessory { 00046 public: 00047 00048 00049 00050 /** Create a AndroidAccessory object 00051 * 00052 * Create a AndroidAccessoryobject with specified buffer sizes and infomation 00053 * 00054 * @param rbuffsize The size of the read buffer 00055 * @param wbuffsize The size of the write buffer 00056 * @param manufacturer The manufacturer of the accessory 00057 * @param model The model of the accessory 00058 * @param description A short description of the accessory 00059 * @param version The current version of the accessory 00060 * @param uri Some data to go with the accessory (URL or more description) 00061 * @param serial The serial number of the accessory 00062 */ 00063 AndroidAccessory(int rbuffsize,int wbuffsize, 00064 const char* manufacturer, 00065 const char *model, 00066 const char *description, 00067 const char *version, 00068 const char *uri, 00069 const char *serial 00070 ); 00071 00072 /** Init the device 00073 * This is meant to be implimented by the user of the class 00074 * 00075 * @param device Device number 00076 * @param configuration Configuration 00077 * @param interfaceNumber Inteface number 00078 */ 00079 virtual void init(int device, int configuration, int interfaceNumber); 00080 00081 /** Reset the device 00082 * This is meant to be implimented by the user of the class 00083 * 00084 */ 00085 virtual void resetDevice()=0; 00086 00087 /** Setup the device 00088 * This is meant to be implimented by the user of the class. Called when the device is first intialised 00089 * 00090 */ 00091 virtual void setupDevice()=0; 00092 00093 /** Callback on Read 00094 * This is meant to be implimented by the user of the class. Called when some data has been read in. 00095 * 00096 * @param buff The buffered read in data 00097 * @param len The length of the packet recived 00098 * 00099 */ 00100 virtual int callbackRead(u8 *buff, int len)=0; 00101 00102 /** Callback after Write 00103 * This is meant to be implimented by the user of the class. Called when the write has been finished. 00104 * 00105 */ 00106 virtual int callbackWrite()=0; 00107 00108 /** Write over USB 00109 * This sends the data in the buffer over USB in a packet 00110 * 00111 * @param buff The buffer to write out 00112 * @param len The length of the packet to send 00113 * 00114 */ 00115 int write(u8 *buff, int len); 00116 00117 /** Write over USB 00118 * This sends the data in the buffer over USB in a packet, sends _writebuff and _writebuffsize 00119 * 00120 */ 00121 int write() { 00122 return write(_writebuff,_writebuffsize); 00123 } 00124 00125 /** Write over USB with no callback 00126 * This sends the data in the buffer over USB in a packet, waits until the packet is sent, rather than doing a callback 00127 * 00128 * @param buff The buffer to write out 00129 * @param len The length of the packet to send 00130 * 00131 */ 00132 int writeNC(u8 *buff, int len); 00133 00134 /** Write over USB 00135 * This sends the data in the buffer over USB in a packet, waits until the packet is sent, rather than doing a callback, sends _writebuff and _writebuffsize 00136 * 00137 */ 00138 int writeNC() { 00139 return writeNC(_writebuff,_writebuffsize); 00140 } 00141 00142 /** Read the buffer USB 00143 * This sends the data in the buffer over USB in a packet, waits until the packet is sent, rather than doing a callback 00144 * 00145 * @param buff The buffer to read into 00146 * @param len The length of the packet to read in 00147 * 00148 * @param returns The number of bytes read 00149 * 00150 */ 00151 int read(u8 *buff, int len); 00152 00153 00154 void adkEnd() { 00155 // _initok=false; 00156 resetDevice(); 00157 }; //if connection close 00158 bool switchDevice(int device); 00159 00160 //buffer 00161 u8* _readbuff; 00162 int _readbuffsize; 00163 u8* _writebuff; 00164 int _writebuffsize; 00165 u8* _strbuff;//255bytes; 00166 void sendString(const char *str); 00167 00168 private: 00169 00170 void sendString(int device, int index, const char *str); 00171 int getProtocol(int device); 00172 00173 const char *manufacturer; 00174 const char *model; 00175 const char *description; 00176 const char *version; 00177 const char *uri; 00178 const char *serial; 00179 00180 //endpoints 00181 int input_ep; 00182 int output_ep; 00183 00184 int _device; 00185 int _configuration; 00186 int _interfaceNumber; 00187 00188 //bool _initok; 00189 00190 }; 00191 00192 extern AndroidAccessory* _adk; 00193 00194 00195 #endif
Generated on Tue Jul 12 2022 15:55:45 by
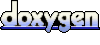