
MP3 Player. You can change fwd/rev speed and skip. see: http://mbed.org/users/okini3939/notebook/lpc4088_madplayer/
Dependencies: I2SSlave SDFileSystem TLV320 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "player.h" 00003 00004 Serial pc(USBTX, USBRX); 00005 DigitalOut led1(LED1), led2(LED2), led3(LED3), led4(LED4); 00006 00007 Ticker ticker; 00008 00009 extern int dac_step; 00010 00011 00012 extern "C" void HardFault_Handler() { 00013 printf("Hard Fault!\r\n"); 00014 exit(-1); 00015 } 00016 00017 void isr_ticker () 00018 { 00019 static int w = 0; 00020 00021 // LED off 00022 w ++; 00023 if (w >= 10) { 00024 led4 = 0; 00025 w = 0; 00026 } 00027 } 00028 00029 void poll () { 00030 int i; 00031 static char buf[40]; 00032 static char count = 0; 00033 00034 // serial control 00035 if (pc.readable()) { 00036 i = pc.getc(); 00037 if (i == 0x0d || i == 0x0a) { 00038 buf[count] = 0; 00039 count = 0; 00040 led3 = 0; 00041 if (command(buf)) { 00042 led3 = 1; 00043 } 00044 } else 00045 if (i >= 0x20 && i < 0x7f && count < sizeof(buf) - 1) { 00046 buf[count] = i; 00047 count ++; 00048 } 00049 } 00050 } 00051 00052 int main(int argc, char *argv[]) 00053 { 00054 int i; 00055 00056 pc.baud(115200); 00057 pc.printf("madplayer LPC4088\r\n"); 00058 ticker.attach(&isr_ticker, 0.01); 00059 wait_ms(100); 00060 if (init_audio()) return -1; 00061 00062 led1 = 0; 00063 led2 = 1; 00064 00065 for (;;) { 00066 poll(); 00067 } 00068 }
Generated on Tue Jul 12 2022 15:53:02 by
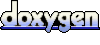