Daisen Remote library
Embed:
(wiki syntax)
Show/hide line numbers
daisenRemote.h
00001 /* 00002 * Daisen Remote library 00003 * Copyright (c) 2015 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 * 00006 * 2.4GHz: http://www.daisendenshi.com/category_list.php?master_categories_id=102&categories_id=141&categories_name=2.4GHz%91%D1%96%B3%90%FC%83%8A%83%82%83R%83%93%91%97%90M%8A%ED 00007 * Infrared: http://www.daisendenshi.com/category_list.php?master_categories_id=102&categories_id=106&categories_name=%90%D4%8AO%83%8A%83%82%83R%83%93%91%97%90M%8A%ED 00008 */ 00009 00010 #include "mbed.h" 00011 #include "FunctionPointer.h" 00012 00013 class daisenRemote { 00014 public: 00015 enum remoteType { 00016 RF, // 2.4GHz 00017 IR, // Infrared 00018 }; 00019 00020 daisenRemote (PinName rx, remoteType type = RF); 00021 00022 int read (); 00023 00024 void attach(void (*fptr)(void)) { 00025 _func.attach(fptr); 00026 } 00027 template<typename T> 00028 void attach(T *tptr, void (T::*mptr)(void)) { 00029 _func.attach(tptr, mptr); 00030 } 00031 00032 private: 00033 RawSerial _ir; 00034 remoteType _type; 00035 int _recv; 00036 int _count; 00037 char _buf[20]; 00038 00039 FunctionPointer _func; 00040 00041 void isrRemote (); 00042 };
Generated on Wed Jul 20 2022 04:29:19 by
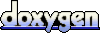