Daisen Remote library
Embed:
(wiki syntax)
Show/hide line numbers
daisenRemote.cpp
00001 /* 00002 * Daisen Remote library 00003 * Copyright (c) 2015 Hiroshi Suga 00004 * Released under the MIT License: http://mbed.org/license/mit 00005 * 00006 * 2.4GHz: http://www.daisendenshi.com/category_list.php?master_categories_id=102&categories_id=141&categories_name=2.4GHz%91%D1%96%B3%90%FC%83%8A%83%82%83R%83%93%91%97%90M%8A%ED 00007 * Infrared: http://www.daisendenshi.com/category_list.php?master_categories_id=102&categories_id=106&categories_name=%90%D4%8AO%83%8A%83%82%83R%83%93%91%97%90M%8A%ED 00008 */ 00009 00010 #include "daisenRemote.h" 00011 00012 daisenRemote::daisenRemote (PinName rx, enum remoteType type) : _ir(NC, rx) { 00013 _recv = 0; 00014 _count = 0; 00015 _type = type; 00016 if (_type == RF) { 00017 _ir.baud(38400); 00018 } else 00019 if (_type == IR) { 00020 _ir.baud(19200); 00021 } 00022 _ir.attach(this, &daisenRemote::isrRemote, Serial::RxIrq); 00023 } 00024 00025 int daisenRemote::read () { 00026 int r = _recv; 00027 _recv = 0; 00028 return r; 00029 } 00030 00031 void daisenRemote::isrRemote () { 00032 char c = _ir.getc(); 00033 00034 if (_type == RF) { 00035 // 2.4GHz Radio 00036 if (_count == 0 && c == 0x0f) { 00037 _buf[_count] = c; 00038 _count ++; 00039 } else 00040 if (_count == 1) { 00041 if (c == 0x5a) { 00042 _buf[_count] = c; 00043 _count ++; 00044 } else { 00045 _count = 0; 00046 } 00047 } else 00048 if (_count >= 2 && _count < 19) { 00049 _buf[_count] = c; 00050 _count ++; 00051 if (_count == 19) { 00052 if (_buf[13] == 0x83 && _buf[14] == 0x41 && 00053 _buf[17] != 0xff && _buf[17] == (~_buf[18] & 0xff)) { 00054 _recv = _buf[17]; 00055 _func.call(); 00056 } 00057 _count = 0; 00058 } 00059 } else { 00060 _count = 0; 00061 } 00062 } else 00063 if (_type == IR) { 00064 // Infrared 00065 if (c == 0x0a || c == 0x0d) { 00066 if (_count == 2) { 00067 _buf[_count] = 0; 00068 _recv = atoi(_buf); 00069 _func.call(); 00070 } 00071 _count = 0; 00072 } else 00073 if (_count < 2) { 00074 if (c >= '0' && c <= '9') { 00075 _buf[_count] = c; 00076 _count ++; 00077 } else { 00078 _count = 0; 00079 } 00080 } 00081 } 00082 }
Generated on Wed Jul 20 2022 04:29:19 by
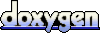