
Test Program for LPS331 I2C Library.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "LPS331_I2C.h" 00003 00004 Serial pc(USBTX, USBRX); 00005 LPS331_I2C lps331(p9, p10, LPS331_I2C_SA0_HIGH); 00006 00007 00008 int main() { 00009 pc.printf("LPS331 Test Program.\r\n"); 00010 00011 if(lps331.isAvailable()) { 00012 pc.printf("LPS331 is available!\r\n"); 00013 } else { 00014 pc.printf("LPS331 is unavailable!\r\n"); 00015 } 00016 00017 lps331.setResolution(LPS331_I2C_PRESSURE_AVG_512, LPS331_I2C_TEMP_AVG_128); 00018 lps331.setDataRate(LPS331_I2C_DATARATE_7HZ); 00019 lps331.setActive(true); 00020 00021 pc.printf("LPS331 Register map.\r\n"); 00022 00023 for(int i = 0; i < 8; i++) { 00024 pc.printf("%02x: ", i); 00025 for(int j = 0; j < 16; j++) { 00026 char value = lps331._read(j | i << 4); 00027 pc.printf("%02x ", value); 00028 } 00029 pc.printf("\r\n"); 00030 } 00031 00032 pc.printf("LPS331 Register map(multibyte read test).\r\n"); 00033 00034 for(int i = 0; i < 8; i++) { 00035 char data[16]; 00036 lps331._read_multibyte(i << 4, data, 16); 00037 pc.printf("%02x: ", i); 00038 for(int j = 0; j < 16; j++) { 00039 pc.printf("%02x ", data[j]); 00040 } 00041 pc.printf("\r\n"); 00042 } 00043 00044 wait(2.0); 00045 00046 while(true) { 00047 float pres, temp; 00048 00049 pres = lps331.getPressure(); 00050 temp = lps331.getTemperature(); 00051 00052 pc.printf("%f,%f\r\n", pres, temp); 00053 00054 wait(1/7.0); 00055 } 00056 00057 }
Generated on Sun Jul 17 2022 16:44:31 by
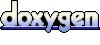