Yalgaar mBed SDK for real-time messaging
Fork of MQTT by
Embed:
(wiki syntax)
Show/hide line numbers
PubSubClient.h
00001 /* 00002 PubSubClient.h - A simple client for MQTT. 00003 Nicholas O'Leary 00004 http://knolleary.net 00005 */ 00006 #include "mbed.h" 00007 #include "EthernetInterface.h" 00008 #include "TCPSocketConnection.h" 00009 00010 #ifndef PubSubClient_h 00011 #define PubSubClient_h 00012 00013 #define MQTT_CALLBACK_SIGNATURE void (*callback)(char*,char*, unsigned int) 00014 00015 // MQTT_MAX_PACKET_SIZE : Maximum packet size 00016 #define MQTT_MAX_PACKET_SIZE 2048 00017 00018 // MQTT_KEEPALIVE : keepAlive interval in Seconds 00019 #define MQTT_KEEPALIVE 60 00020 00021 #define MQTT_CONNECTION_TIMEOUT -4 00022 #define MQTT_CONNECTION_LOST -3 00023 #define MQTT_CONNECT_FAILED -2 00024 #define MQTT_DISCONNECTED -1 00025 #define MQTT_CONNECTED 0 00026 #define MQTT_CONNECT_BAD_PROTOCOL 1 00027 #define MQTT_CONNECT_BAD_CLIENT_ID 2 00028 #define MQTT_CONNECT_UNAVAILABLE 3 00029 #define MQTT_CONNECT_BAD_CREDENTIALS 4 00030 #define MQTT_CONNECT_UNAUTHORIZED 5 00031 00032 #define MQTTPROTOCOLVERSION 3 00033 #define MQTTCONNECT 1 << 4 // Client request to connect to Server 00034 #define MQTTCONNACK 2 << 4 // Connect Acknowledgment 00035 #define MQTTPUBLISH 3 << 4 // Publish message 00036 #define MQTTPUBACK 4 << 4 // Publish Acknowledgment 00037 #define MQTTPUBREC 5 << 4 // Publish Received (assured delivery part 1) 00038 #define MQTTPUBREL 6 << 4 // Publish Release (assured delivery part 2) 00039 #define MQTTPUBCOMP 7 << 4 // Publish Complete (assured delivery part 3) 00040 #define MQTTSUBSCRIBE 8 << 4 // Client Subscribe request 00041 #define MQTTSUBACK 9 << 4 // Subscribe Acknowledgment 00042 #define MQTTUNSUBSCRIBE 10 << 4 // Client Unsubscribe request 00043 #define MQTTUNSUBACK 11 << 4 // Unsubscribe Acknowledgment 00044 #define MQTTPINGREQ 12 << 4 // PING Request 00045 #define MQTTPINGRESP 13 << 4 // PING Response 00046 #define MQTTDISCONNECT 14 << 4 // Client is Disconnecting 00047 #define MQTTReserved 15 << 4 // Reserved 00048 00049 #define MQTTQOS0 (0 << 1) 00050 #define MQTTQOS1 (1 << 1) 00051 #define MQTTQOS2 (2 << 1) 00052 00053 00054 00055 class PubSubClient { 00056 private: 00057 TCPSocketConnection _client; 00058 char buffer[MQTT_MAX_PACKET_SIZE]; 00059 int nextMsgId; 00060 unsigned long lastOutActivity; 00061 unsigned long lastInActivity; 00062 bool pingOutstanding; 00063 MQTT_CALLBACK_SIGNATURE; 00064 int readPacket(int); 00065 char readByte(); 00066 bool write(short header, char* buf, int length); 00067 int writeString(char* string, char* buf, int pos); 00068 char* ip; 00069 int port; 00070 Timer t; 00071 int _state; 00072 public: 00073 PubSubClient(); 00074 PubSubClient(char*, int); 00075 00076 PubSubClient& setCallback(MQTT_CALLBACK_SIGNATURE); 00077 00078 bool connect(char *); 00079 bool connect(char *, char *, char *); 00080 bool connect(char *, char *, short, short, char *); 00081 bool connect(char *, char *, char *, char *, short, short, char*); 00082 void disconnect(); 00083 bool publish(char *, char *); 00084 bool publish(char *, char *, unsigned int); 00085 bool publish(char *, char *, unsigned int, bool); 00086 // bool publish_P(char *, short PROGMEM *, unsigned int, bool); 00087 bool subscribe(char *); 00088 bool unsubscribe(char *); 00089 bool loop(); 00090 bool connected(); 00091 int mqtt_state(); 00092 int millis(); 00093 }; 00094 00095 00096 #endif
Generated on Wed Jul 13 2022 09:17:26 by
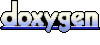