
f303k8 wav player
Dependencies: SDFileSystem mbed
main.cpp
00001 #include "mbed.h" 00002 #include <stdio.h> 00003 #include "SDFileSystem.h" 00004 #include "wavPlayer.h" 00005 #include "TIM6Driver.h" 00006 00007 void TIM6_IRQHandler(void); 00008 Ticker flipper; 00009 FATFS FatFs; 00010 wavPlayerOnDAC wavPlayer(PA_4); 00011 00012 SDFileSystem sd(PB_5, PB_4, PB_3, PA_11, "sd"); // the pinout on the mbed Cool Components workshop board 00013 DigitalIn button(PF_1,PullDown); 00014 BusIn DipSW(PA_10,PA_9,PA_12,PB_0); 00015 00016 void TIM6_IRQHandler() { 00017 TIM6ClearInt(); 00018 wavPlayer.DACOutProc(); 00019 } 00020 00021 int main(void) 00022 { 00023 FRESULT wavFileResult; 00024 FIL fil; 00025 char fileName[10]="0.wav"; 00026 uint8_t oldDipSW=0; 00027 uint8_t oldButton=0; 00028 00029 MX_TIM6_Init(); 00030 NVIC_SetVector(TIM6_DAC1_IRQn, (uint32_t)&TIM6_IRQHandler); 00031 MX_NVIC_Init(); 00032 00033 DipSW.mode(PullDown); 00034 f_mount(&FatFs,"",0); 00035 printf("power ON \r\n"); 00036 wavFileResult = f_open(&fil, "0.wav",FA_READ); 00037 00038 while(1) 00039 { 00040 while(button.read() == 0) 00041 { 00042 } 00043 if(oldDipSW != DipSW.read()) 00044 { 00045 f_close(&fil); 00046 fileName[0] = DipSW.read()+0x30; 00047 printf("name:%s\r\n",fileName); 00048 wavFileResult = f_open(&fil, fileName, FA_READ); 00049 } 00050 oldDipSW = DipSW.read(); 00051 printf("error:%d\r\n",(int)wavFileResult); 00052 wavPlayer.setFile(&fil); 00053 printf("start\r\n"); 00054 wavPlayer.rampUp(); 00055 oldButton = button.read(); 00056 while(wavPlayer.readProc() == 0) 00057 { 00058 if(button.read() == 1 && oldButton == 0) 00059 { 00060 break; 00061 } 00062 oldButton = button.read(); 00063 } 00064 printf("END\r\n"); 00065 wavPlayer.rewind(); 00066 wavPlayer.rampDown(); 00067 while(button.read() == 1 && oldButton == 1) 00068 { 00069 } 00070 00071 } 00072 }
Generated on Tue Jul 19 2022 13:35:40 by
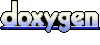