ADS1246/7/8 24bit ADC converter for Temperature Sensors class
Embed:
(wiki syntax)
Show/hide line numbers
ADS1248.h
00001 #ifndef ADS1248_H 00002 #define ADS1248_H 00003 00004 #include "mbed.h" 00005 /** ADS1248 class. 00006 * Used for read ADS1248/7/6 Temperature ADC 00007 * 00008 */ 00009 class ADS1248 00010 { 00011 public: 00012 /** Create ADS1248 instance 00013 * @param SPI SPI bus to use 00014 * @param cs pin to connect at CS input 00015 * @param rdy pin connect at !rdy output 00016 * @param start pin connect at start inpout (pullup if unused) 00017 */ 00018 ADS1248(SPI& spi, PinName cs, PinName rdy, PinName start = NC); 00019 00020 /** register name list of ADS1248 00021 */ 00022 enum reg{ 00023 MUX0 = 0x00, 00024 VBIAS = 0x01, 00025 MUX1 = 0x02, 00026 SYS0 = 0x03, 00027 OFC0 = 0x04, 00028 OFC1 = 0x05, 00029 OFC2 = 0x06, 00030 FSC0 = 0x07, 00031 FSC1 = 0x08, 00032 FSC2 = 0x09, 00033 IDAC0 = 0x0A, 00034 IDAC1 = 0x0B, 00035 GPIOCFG = 0x0C, 00036 GPIODIR = 0x0D, 00037 GPIODAT = 0x0E}; 00038 00039 /** commande list of ADS1248 00040 */ 00041 enum cmd{ 00042 WAKEUP = 0x00, 00043 SLEEP = 0x02, 00044 SYNC = 0x04, 00045 RESET = 0x06, 00046 NOP = 0xff, 00047 RDATA = 0x12, 00048 RDATAC = 0x14, 00049 SDATAC = 0x16, 00050 RREG = 0x20, 00051 WREG = 0x40, 00052 SYSOCAL = 0x60, 00053 SYSGCAL = 0x61, 00054 SELFOCAL= 0x62}; 00055 00056 /** Access to output start pin 00057 * @param en start pin state 00058 */ 00059 void start(bool en); 00060 00061 /** Wait ADS1248 to be ready (pooling method) 00062 */ 00063 void waitReady(void); 00064 00065 /** Sleep cmd of ADS1248 (ask waitReady) 00066 * @param en for wakeup or sleep 00067 */ 00068 void sleep(bool en); 00069 00070 /** Synchronisation cmd of ADS1248 00071 */ 00072 void sync(void); 00073 00074 /** Reset cmd of ADS1248 00075 */ 00076 void reset(void); 00077 00078 /** Read data when conversion was finished (ask waitReady) 00079 * @return 24bit data 2's complement 00080 */ 00081 int read(void); 00082 00083 /** Ask read 00084 * @return 24bit data 2's complement 00085 */ 00086 operator int(); 00087 00088 /** Read ADS1248 register 00089 * @param reg is register address 00090 * @return register value 00091 */ 00092 unsigned char readReg(unsigned char reg); 00093 00094 /** Read ADS1248 registers 00095 * @param reg is first register address 00096 * @param buff pointer on buffer to write 00097 * @param len lenght of data to read 00098 */ 00099 void readReg(unsigned char reg, unsigned char *buff, int len); 00100 00101 /** Write ADS1248 register 00102 * @param reg is register address 00103 * @param val value to write 00104 */ 00105 void writeReg(unsigned char reg, unsigned char val); 00106 00107 /** Write ADS1248 registers 00108 * @param reg is first register address 00109 * @param buff pointer on buffer to read 00110 * @param len lenght of data to write 00111 */ 00112 void writeReg(unsigned char reg, const unsigned char *buff, int len); 00113 00114 /** System Offset Calibration cmd of ADS1248 (ask waitReady) 00115 */ 00116 void systemOffsetCal(void); 00117 00118 /** System Gain Calibration cmd of ADS1248 (ask waitReady) 00119 */ 00120 void systemGainCal(void); 00121 00122 /** Self Offset Calibration cmd of ADS1248 (ask waitReady) 00123 */ 00124 void selfOffsetCal(void); 00125 00126 00127 private: 00128 SPI& _spi; 00129 DigitalOut _cs; 00130 DigitalIn _rdy; 00131 DigitalOut _start; 00132 }; 00133 00134 #endif
Generated on Sat Jul 16 2022 23:46:51 by
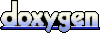