
save loops
Embed:
(wiki syntax)
Show/hide line numbers
rigidLoop.h
00001 #ifndef RIGID_LOOP 00002 #define RIGID_LOOP 00003 00004 // Include the basic objects to create the loop 00005 #include "soundSpot.h" 00006 #include "classPointMass.h" // this may be used to move the center of the RIGID loop in dynamically "real" ways 00007 #include "classSpring.h" // same remark than above 00008 00009 using namespace std; 00010 00011 enum RigidLoopMode {SPOT_FOLLOWING, SPOT_GHOST, SPOT_PACMAN, SPOT_AIR_HOCKEY, SPOT_LORENTZ_FORCE, 00012 SPOT_BOUNCING, SPOT_BOUNCING_FACTOR, 00013 SPOT_TEST, SPOT_TRACK, SPOT_TRACK_DOT, 00014 EXPLOSION}; 00015 00016 class rigidLoop : public soundSpot { 00017 00018 public: 00019 00020 // Constructor and destructor: 00021 rigidLoop(); 00022 virtual ~rigidLoop(); 00023 00024 // instantiation of the virtual methods of the base class (we don't need to append "virtual", but for clarity I do): 00025 void createBlob(int _id, RigidLoopMode _rigidBlobMode, vector2Df _initPos, vector2Df _initSpeed); 00026 virtual void setRegionMotion(float mmix, float mmiy, float mmax, float mmay); // attention: initial position posX and posY should be inside this bounding box... 00027 virtual void update(vector2Df referencePos); // update dynamics of the mass loop 00028 virtual void draw(void); // draw the blob (renders on the laser trajectory object lsdTrajectory from the base class, using the openGL laser renderer - not yet done). 00029 virtual void computeBoundingBox(); 00030 virtual void sendDataSpecific(void); 00031 virtual void setSpeed(float speed); 00032 virtual void setSize(float size); 00033 virtual void speedFactor(float speedfactor); 00034 virtual void sizeFactor(float sizeFactor); 00035 00036 virtual void explosion(); // not very nice programming style, it should be a MODE instead or something like that. 00037 virtual vector2Df getCenter(); 00038 virtual void resetPositionSpeed() {centerMass.setInitialCondition(startCenter, startSpeed);}; 00039 virtual void setPositionSpeed(vector2Df _pos, vector2Df _spe) {centerMass.setInitialCondition(_pos, _spe);}; 00040 00041 virtual void showChildParameters(); 00042 00043 // methods that are new to this class (not in base class): 00044 void initSizeBlob(int _numPoints); 00045 void createLoopFromScafold(void); // this is much simpler than the elastic blob (here, we only need to add a central mass) 00046 // void processLoopData(); // not needed, because the loop is rigid. 00047 00048 RigidLoopMode updateMode; 00049 00050 // The number of points in the loop (in this case, is just the number of points in the scaffold, as well as the number of points in the lsdTrajectory) 00051 // int numPoints; // this belongs to soundSpot base class 00052 pointMass centerMass; // this is the center of the rigidLoop. Note that it can have mass or not, this will depend on the RigidLoopMode 00053 float integrationStep; 00054 00055 // The following are common to all blobs: 00056 // float angleCorrectionForceLoop; 00057 // vector2D recenteringVectorLoop; 00058 // float angleRecenteringVector; // auxiliary variables for sending data (for the recenteringVectorLoop) 00059 // float normRecenteringVector; 00060 00061 // other modes: 00062 bool slidingDirection; // for contour following 00063 00064 // numeric parameters: 00065 float saccadeRadius; 00066 00067 // THINGS FOR CONTOUR FOLLOWING: 00068 float saccadeRadius_initial; // this is for SEARCH MODE (remember initial radius) 00069 bool justSearched; 00070 00071 00072 float rotationAngle; // note: in the future, rotation would be done by the openGL-like renderer 00073 00074 float massCenter; 00075 float dampMotionCenterMass; 00076 00077 00078 float speedContourFollowing; // this is given as a percentage of the radius of the circle 00079 float factorBouncingForce; // spring force when penetrating on dark zone (used by SPOT_BOUNCING) 00080 float factorAbsorptionShock; // factor speed damping when bouncing on dark zone (used in SPOT_BOUNCING_FACTOR) 00081 }; 00082 00083 #endif
Generated on Tue Jul 12 2022 21:23:04 by
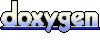