
save loops
Embed:
(wiki syntax)
Show/hide line numbers
mbedOSC.h
00001 /* mbedOSC.h 00002 This is an OSC library for the mbed, created to be compatible with Recotana's OSCClass library (http://recotana.com) for the 00003 Arduino with Ethernet shield. I have also used parts of the OSC Transceiver(Sender/Receiver) code by xshige 00004 written by: Alvaro Cassinelli, 7.10.2011 00005 00006 This library is free software; you can redistribute it and/or 00007 modify it under the terms of the GNU Lesser General Public 00008 License version 2.1 as published by the Free Software Foundation. 00009 Open Sound Control http://opensoundcontrol.org/ 00010 00011 mbedOSC version 0.1 Specification (similar to Recotana's OSCClass library) 00012 00013 ******** 00014 Address : max 2 00015 "/ard" 00016 "/ard/output" --address[0]="/ard" :max 15character 00017 --address[1]="/output" :max 15character 00018 00019 ******* 00020 TypeTag : max 2 00021 00022 "i" - long or unsigned long 00023 "f" - double 00024 00025 ******* 00026 arg : max 2 00027 00028 ******* 00029 Example of an OSC message: "/mbed/test1, if 50 32.4" (Note: this is not the byte string 00030 sent as UDP packet - there are no spaces, and arguments are in binary, BIG ENDIAN) 00031 */ 00032 00033 #ifndef mbedOSC_h 00034 #define mbedOSC_h 00035 00036 #include "mbed.h" 00037 #include "EthernetNetIf.h" 00038 #include "UDPSocket.h" 00039 00040 // setup IP of destination (computer): 00041 #define DEFAULT_SEND_PORT 12000 00042 //Host sendHost(IpAddr(10, 0, 0, 1), DEFAULT_SEND_PORT, NULL); // Send Port 00043 // set IP of origin of UDP packets - the mbed acts as a SERVER here, and needs to bind the socket to the "client" (the computer) 00044 #define DEFAULT_RECEIVE_PORT 57130 00045 //Host recHost(IpAddr(10, 0, 0, 1), DEFAULT_RECEIVE_PORT, NULL); // Receive Port 00046 //UDPSocket udpRec,udpSend; 00047 00048 00049 #define MAX_ADDRESS 2 00050 #define MAX_ARG 2 00051 00052 #define TYPE_INT 1 00053 #define TYPE_FLOAT 2 00054 #define TYPE_BLOB 3 00055 00056 00057 /* 00058 Container class for OSC messages (receiving or sending) 00059 */ 00060 class OSCMessage{ 00061 00062 private: 00063 00064 char *address[MAX_ADDRESS]; // these are strings (as char*) 00065 uint8_t addressNum; // current number of addresses in the message (ex: "/ard/test" --> the number of the addresses is 2) 00066 00067 char typeTag[MAX_ARG]; 00068 00069 void *arg[MAX_ARG]; 00070 uint8_t argNum; 00071 00072 // Information about the connection: 00073 //uint8_t ip[4]; 00074 //uint16_t port; 00075 Host host; 00076 00077 public: 00078 00079 OSCMessage(); 00080 00081 const IpAddr& getIp(); // return IpAddr object 00082 const int& getPort(); // return port 00083 00084 //ex. address patern "/adr/test" 00085 // address[2]={"/ard" , "/test"} 00086 char *getAddress(uint8_t _index); //retturn address 00087 char *getTopAddress(); //return address[0] :"/ard" 00088 char *getSubAddress(); //return address[1] :"/test" 00089 uint8_t getAddressNum(); //return 2 00090 00091 // 'i': long(int32_t) 00092 // 'f': double 00093 //ex 'if' 123 54.21 00094 char getTypeTag(uint8_t _index); //_index=0 ->'i' 00095 //_index=1 ->'f' 00096 00097 uint8_t getArgNum(); //return 2 00098 00099 int32_t getArgInt(uint8_t _index); //_index=0 -> 123 00100 double getArgFloat(uint8_t _index); //_index=1 -> 54.21 00101 00102 00103 void setTopAddress(char *_address); //set address[0] 00104 void setSubAddress(char *_address); //set address[1] 00105 void setAddress(char *_topAddress, 00106 char *_subAddress); 00107 void setAddress(uint8_t _index, //set 0,address[0] 00108 char *_address); 00109 //set 1,address[1] 00110 00111 void setIp( uint8_t *_ip ); //set ip 00112 00113 void setIp(uint8_t _ip1, //set(192, 00114 uint8_t _ip2, // 168, 00115 uint8_t _ip3, // 0, 00116 uint8_t _ip4); // 100) 00117 00118 void setPort( uint16_t _port ); 00119 00120 //ex. long v1=100 00121 // double v2=123.21 00122 void setArgs( char *types , ... ); //set ("if",&v1,&v2) 00123 void clearArgs(); // clear arguments 00124 00125 friend class OSCClass; 00126 00127 }; 00128 00129 00130 00131 /* ==================================== OSCClass for sending and receiving OSC messages using UDP protocol ===================================== */ 00132 00133 #include "UDPSocket.h" 00134 00135 class OSCClass { 00136 00137 private: 00138 00139 UDPSocket udpRec,udpSend; 00140 char rcvBuff[256]; // raw buffer for UDP packets (udpRec.recvfrom( buf, 256, &host ) )) 00141 int buflength; 00142 00143 OSCMessage *receiverMessage; 00144 OSCMessage *sendContainer; 00145 00146 char tempAddress[MAX_ADDRESS][16]; 00147 uint8_t tempArg[MAX_ARG][4]; 00148 00149 void decodePacket( OSCMessage *_mes); // makes OSC message from packet 00150 00151 public: 00152 00153 OSCClass(); 00154 OSCClass(OSCMessage *_mes); // set the receiver message container 00155 void onUDPSocketEvent(UDPSocketEvent e); 00156 00157 //init osc 00158 void begin(void (*fp)(UDPSocketEvent)); 00159 void begin(uint16_t _recievePort, void (*fp)(UDPSocketEvent)); 00160 void stop(); 00161 00162 //new OSC data in the receiver message container: 00163 bool newMessage; 00164 00165 void setReceiveMessage( OSCMessage *_mes ); //set receive OSCmessage container (note: the message has a "host" object from which we get the upd packets) 00166 OSCMessage *getMessage(); //return received OSCmessage 00167 00168 //buffer clear 00169 //void flush(); 00170 00171 //OSC send 00172 void sendOsc( OSCMessage *_mes ); //set&send OSCmessage (note: it will be sent to the host defined in the message container) 00173 00174 // AND THIS IS BAD, need a better function: 00175 void sendOscBlob( uint8_t * myblob, int sizeblob, OSCMessage *_mes ); 00176 void sendOscString( uint8_t * myblob, int sizeblo, OSCMessage *_mesb ); 00177 }; 00178 00179 #endif
Generated on Tue Jul 12 2022 21:23:04 by
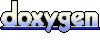