
save loops
Embed:
(wiki syntax)
Show/hide line numbers
classPointMass.h
00001 /* 00002 * pointMass.h 00003 * laserBlob 00004 * 00005 * Created by CASSINELLI ALVARO on 5/19/11. 00006 * Copyright 2011 TOKYO UNIVERSITY. All rights reserved. 00007 * 00008 */ 00009 00010 #ifndef POINTMASS_H 00011 #define POINTMASS_H 00012 00013 #include "myVectorClass.h" 00014 00015 #define VERLET_METHOD // comment this to have EULER method 00016 00017 #define MAX_PERMISSIBLE_SPEED 35 00018 00019 class pointMass 00020 { 00021 public: 00022 00023 // ==================================== Static variables and methods ============== 00024 static vector2Df maxWall, minWall; //equal for ALL THE MASS OBJECTS (declare static). But it could be per-mass. The problem with that approach would be too much wasted memory. 00025 // NOTE: - a static member variable has the same value in any instance of the class and doesn't even require an instance of the class to exist. 00026 // - a static class member cannot be initialized inside of the class declaration. In fact, if you decide to put your code in a header file, you cannot even initialize the static variable 00027 // inside of the header file; do it in a .cpp file instead. Moreover, you are required to initialize the static class member or it will not be in scope. 00028 // The syntax is a bit weird: "type class_name::static_variable = value", or in this case vector2Df pointMass::maxWall(4095, 4095) 00029 00030 // methods: 00031 static void setWallLimits(float mminx, float mminy, float mmaxx, float mmaxy); 00032 00033 // ==================================== METHODS ==================================== 00034 pointMass(); 00035 virtual ~pointMass(){}; 00036 00037 // Adding forces to total force: 00038 void resetForce(); 00039 void addForce(float x, float y); 00040 void addForce(vector2Df force); 00041 void addDampingForce(); 00042 void addInvSquareForce(float x, float y, float radiusMax, float radiusMin, float scale); 00043 void addInterInvSquareForce(pointMass &p, float radiusMin, float radiusMax, float scale); 00044 00045 // (a blob object could be defined by a "cord" of chained particles, plus a center) 00046 void addSpringForce(float x, float y, float radius, float scale); 00047 void addInterSpringForce(pointMass &p, float radius, float scale); 00048 //void addClockwiseForce(particle &p, float radius, float scale); 00049 //void addCounterClockwiseForce(particle &p, float radius, float scale); 00050 00051 //void addDampingForce(); // this work in the case of the euler integration; in case of Verlet, we need to do pseudo-damping while calculating 00052 // the acceleration... 00053 00054 // Set parameters: 00055 void setInitialCondition(float px, float py, float vx, float vy); 00056 void setInitialCondition(vector2Df _pos, vector2Df _speed); 00057 void setIntegrationStep(float _dt); 00058 00059 void setPos(float px, float py); // assuming the speed is unchanged (must do some tweaking in case of Verlet integration) 00060 00061 // dynamic update: 00062 void update(); 00063 00064 // kinematic constraints (could be based on a force too...) 00065 //void setWallLimits(float mminx, float mminy, float mmaxx, float mmaxy); // DECLARED STATIC 00066 void bounceOffWalls(); 00067 00068 vector2Df getSpeed(); // get an estimation of the speed (also update speed variable - this variable is not needed in case of VERLET) 00069 void setSpeed(const vector2Df& vel); 00070 void setSpeed(float vx, float vy); 00071 00072 // ==================================== VARIABLES ==================================== 00073 00074 int identifier; // this may be needed in particular in case we don't use vector<> (case of poor C Arduino compiler) 00075 00076 // kinematic variables: 00077 vector2Df pos, posOld; // I will use verlet integration (perhaps we could have a switch to choose the integration method?) 00078 //vector2D speed; // speed at time t (this is not explicitly calculated in case of verlet method, HENCE PRIVATE) 00079 vector2Df acc; // Acceleration at time t (equal to the total force divided by the mass). No real need to have it here, but convenient to check. 00080 vector2Df totalForce; // this is just for convenience and speeding up calculation when adding forces, before computing acc. 00081 00082 // integration step: 00083 float dt; 00084 00085 // physical parameters: 00086 float dampMotion; 00087 float dampBorder; 00088 float mass; 00089 bool bFixed; // these could act as control points that could be loaded (letters...). In fact, we could use mass to set this (like mass=-1) 00090 00091 // other things: 00092 bool bWallCollision; // this is generic (detect any collision with a side) 00093 vector2Df innerCollitionDirection; // this is smarter than a boolean, because I can detect which side was touched and do different things (but may be memory consuming) 00094 00095 protected: 00096 00097 private: 00098 vector2Df speed; // speed at time t (this is not explicitly calculated in case of verlet method, HENCE MAY BE PRIVATE) 00099 00100 }; 00101 00102 00103 #endif //POINTMASS_H
Generated on Tue Jul 12 2022 21:23:04 by
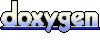