
A simple program to demonstrate how to use a RockerSW object on the 4D systems touch screen display with the mbed as a host microcontroller
main.cpp
00001 /* An mbed tutorial example using the 00002 4.3' PCT 4d systems touch screen display. 00003 00004 uses the mbed_genie library ported from the arduino 00005 visie-genie library by Christian B 00006 00007 The display serial TX and RX pins are connected to pin 9 00008 and pin 10 of the mbed, the reset pin is connected to pin 11 00009 00010 For setting up the display in Visie-Genie 00011 00012 The display has a rocker switch object, an LED object, 00013 and a slider object. 00014 00015 The program reads the status of the rocker switch 00016 and was supposed to turn on the LED. 00017 00018 The slider adjusts the flash rate of the LED 00019 00020 The baud rate of the display is set to 115200 baud 00021 00022 The Mbed4dGenie class requires 3 parameters 00023 1 - Tx pin 00024 2 - Rx pin 00025 3 - Reset pin 00026 */ 00027 00028 #include "mbed.h" 00029 #include "mbed_genie.h" 00030 00031 Mbed4dGenie lcd4d(p9,p10,p11); 00032 00033 DigitalOut whiteLED(p26); //LED 1 for indication 00034 int rockersw_val = 0; //holds the status of the 4Dbutton0 Object 00035 00036 int sliderRead = 0; 00037 float flashRate = 1; //variable to store LED flash rate 00038 int flag = 0; //flag variable to store rockerswitch state 00039 00040 //Event handler for the 4d Systems display 00041 void myGenieEventHandler(void) 00042 { 00043 genieFrame Event; 00044 lcd4d.genieDequeueEvent(&Event); 00045 00046 //event report from an object 00047 if(Event.reportObject.cmd == GENIE_REPORT_EVENT) { 00048 if (Event.reportObject.object == GENIE_OBJ_ROCKERSW) { // If the Reported Message was from a rocker switch 00049 if (Event.reportObject.index == 0) { 00050 printf("Rocker switch 0 pressed!\n\r"); 00051 rockersw_val = lcd4d.genieGetEventData(&Event); //extract the MSB and LSB values and pass them to rockersw_val 00052 00053 if (rockersw_val == 0) { //if Rockerswitch0 is off 00054 flag = 0; //"turn off" the LED 00055 printf("Rocker switch switch in off state\n\r"); //print a serial message for debugging 00056 } 00057 00058 else if (rockersw_val == 1) { //if Rockerswitch0 is on 00059 flag = 1; //"turn on" the LED 00060 printf("Rocker switch switch in ON state\n\r"); //print a serial message for debugging 00061 } 00062 } 00063 } 00064 00065 if (Event.reportObject.object == GENIE_OBJ_SLIDER) { // If the Reported Message was from a slider object 00066 if (Event.reportObject.index == 0) { // If the slider object was slider0 00067 printf("Slider object changed!\n\r"); // Display a serial message for debugging 00068 sliderRead = lcd4d.genieGetEventData(&Event); // Read the current silder0 value 00069 printf("SliderRead = %d \n\r", sliderRead); // Display the Slider0 value 00070 flashRate = 0.01; // Set flash rate initially to 10 ms On / Off 00071 flashRate = 1 - (flashRate * sliderRead); // Apply the value of slider0 object to flashRate 00072 00073 00074 } 00075 00076 //Cmd from a reported object (happens when an object read is requested) 00077 if(Event.reportObject.cmd == GENIE_REPORT_OBJ) { 00078 00079 } 00080 } 00081 } 00082 } 00083 00084 int main() 00085 00086 { 00087 lcd4d.genieAttachEventHandler(&myGenieEventHandler); // Call the event handler for the 4D display 00088 00089 lcd4d.genieWriteContrast(0); // Set the display contrast to 0 initially 00090 00091 lcd4d.genieWriteContrast(15); // Set the display contrast to 15 (full brightness) 00092 00093 printf("Langsters's mbed Visi-Genie LED Flash demo \n\r"); // Display a welcome message on the serial monitor 00094 00095 while(1) { // initialise an infinite while loop 00096 00097 if(flag == 1) { // Check if rockerSW object has been activated 00098 whiteLED = 1; // turn real LED ON 00099 wait(flashRate); // keep LED on for a short period of time 00100 whiteLED = 0; // turn real LED ON 00101 wait(flashRate); // turn LED off for a short period of time 00102 00103 } 00104 00105 if(flag == 0) { // Check if rockerSW object has been deactivated 00106 whiteLED = 0; // turn real LED OFF 00107 } 00108 } 00109 } 00110 00111
Generated on Mon Jul 25 2022 01:58:47 by
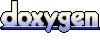