A simple WIP that logs data from a Grove sensor, and can send and receive information over USB and SMS.
Dependencies: DHT DS_1337 SDFileSystem USBDevice mbed
GroveDht22.h
00001 #ifndef __GROVE_DHT_22_H__ 00002 #define __GROVE_DHT_22_H__ 00003 00004 #include "DHT.h" 00005 #include "mbed.h" 00006 #include "AbstractHandler.h" 00007 00008 class MeasurementHandler; 00009 00010 /*! 00011 * \brief The Dht22Result struct is the information read from a Dht22 Grove sensor 00012 */ 00013 struct Dht22Result { 00014 time_t resultTime; ///< timestamp of when the result was returned from the Dht22 00015 float lastCelcius; ///< Temperature result (degC) 00016 float lastHumidity; ///< Humidity result 00017 float lastDewpoint; ///< Dewpoint result 00018 }; 00019 00020 /*! 00021 * \brief The GroveDht22 class handles the interface to the DHT22 humidity and temperature sensor. 00022 * 00023 * The state machine checks for errors and retries and will power cycle the sensor if there are 00024 * GROVE_NUM_RETRIES number of retries. 00025 00026 * The state machine also ensures that at least two seconds is left between readings. 00027 00028 * At any time the parent class can access the last good readings, or the last error. 00029 00030 * The newInfo flag exists so that the parent can decide to only notify (print to terminal or otherwise) when there 00031 * is new information available. Calling the newInfo getter will clear the newInfo flag. 00032 */ 00033 class GroveDht22 : public AbstractHandler 00034 { 00035 public: 00036 GroveDht22(MeasurementHandler *_measure, MyTimers * _timer); 00037 ~GroveDht22(); 00038 00039 void run(); 00040 00041 void setRequest(int request, void *data = 0); 00042 00043 // getters 00044 float lastCelcius() { return _lastCelcius; } 00045 float lastHumidity() { return _lastHumidity; } 00046 float lastDewPoint() { return _lastDewpoint; } 00047 eError lastError() { return _lastError; } 00048 unsigned char newInfo(); 00049 00050 private: 00051 // state machine 00052 typedef enum { 00053 dht_StartTurnOff, ///< Begin by ensuring the sensor is switched off 00054 dht_StartTurnOffWait, ///< Allow it to power down completely 00055 dht_StartTurnOn, ///< Turn the sensor on 00056 dht_StartTurnOnWait, ///< Allow sensor to settle after powering on 00057 dht_TakeMeasurement, ///< Take a measurement, check if valid, update measurment vars, set newInfo 00058 dht_WaitMeasurement ///< Wait for 2 seconds between measurements 00059 } mode_t; 00060 00061 mode_t mode; ///< The current state in the state machine 00062 00063 float _lastCelcius; ///< Last temperature reading from the Dht22 sensor 00064 float _lastHumidity; ///< Last humidity reading from the Dht22 sensor 00065 float _lastDewpoint; ///< Last dewpoint calculation from last temp, humidity vales 00066 unsigned char _newInfo; ///< This flag indicates there is new information (an error, or a measurement) 00067 int _retries; ///< Number of bad readings from the Dht22 sensor 00068 eError _lastError; ///< The last error, or lack thereof 00069 00070 /*! 00071 * \brief powerOn powers the Dht22 on or off, by toggling the enable pin 00072 * \param ON true to power on, false to power off 00073 */ 00074 void powerOn(unsigned char ON); 00075 00076 MeasurementHandler *m_measure; ///< Reference to send measurement results and errors to for handling 00077 00078 DHT *m_sensor; ///< Interface to hardware DHT sensor 00079 }; 00080 00081 #endif // __GROVE_DHT_22_H__
Generated on Tue Jul 12 2022 23:07:44 by
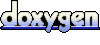